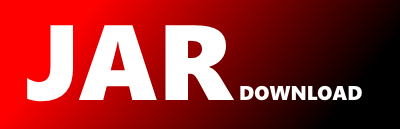
docs.CaptchaApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# CaptchaApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**createCaptchaInstance**](CaptchaApi.md#createCaptchaInstance) | **POST** /api/v1/captchas | Create a CAPTCHA instance |
| [**deleteCaptchaInstance**](CaptchaApi.md#deleteCaptchaInstance) | **DELETE** /api/v1/captchas/{captchaId} | Delete a CAPTCHA Instance |
| [**deleteOrgCaptchaSettings**](CaptchaApi.md#deleteOrgCaptchaSettings) | **DELETE** /api/v1/org/captcha | Delete the Org-wide CAPTCHA Settings |
| [**getCaptchaInstance**](CaptchaApi.md#getCaptchaInstance) | **GET** /api/v1/captchas/{captchaId} | Retrieve a CAPTCHA Instance |
| [**getOrgCaptchaSettings**](CaptchaApi.md#getOrgCaptchaSettings) | **GET** /api/v1/org/captcha | Retrieve the Org-wide CAPTCHA Settings |
| [**listCaptchaInstances**](CaptchaApi.md#listCaptchaInstances) | **GET** /api/v1/captchas | List all CAPTCHA Instances |
| [**replaceCaptchaInstance**](CaptchaApi.md#replaceCaptchaInstance) | **PUT** /api/v1/captchas/{captchaId} | Replace a CAPTCHA Instance |
| [**replacesOrgCaptchaSettings**](CaptchaApi.md#replacesOrgCaptchaSettings) | **PUT** /api/v1/org/captcha | Replace the Org-wide CAPTCHA Settings |
| [**updateCaptchaInstance**](CaptchaApi.md#updateCaptchaInstance) | **POST** /api/v1/captchas/{captchaId} | Update a CAPTCHA Instance |
## createCaptchaInstance
> CAPTCHAInstance createCaptchaInstance(instance)
Create a CAPTCHA instance
Creates a new CAPTCHA instance. Currently, an org can only configure a single CAPTCHA instance.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CaptchaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CaptchaApi apiInstance = new CaptchaApi(defaultClient);
CAPTCHAInstance instance = new CAPTCHAInstance(); // CAPTCHAInstance |
try {
CAPTCHAInstance result = apiInstance.createCaptchaInstance(instance);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CaptchaApi#createCaptchaInstance");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **instance** | [**CAPTCHAInstance**](CAPTCHAInstance.md)| | |
### Return type
[**CAPTCHAInstance**](CAPTCHAInstance.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Created | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## deleteCaptchaInstance
> deleteCaptchaInstance(captchaId)
Delete a CAPTCHA Instance
Deletes a specified CAPTCHA instance > **Note:** If your CAPTCHA instance is still associated with your org, the request fails. You must first update your Org-wide CAPTCHA settings to remove the CAPTCHA instance.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CaptchaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CaptchaApi apiInstance = new CaptchaApi(defaultClient);
String captchaId = "captchaId_example"; // String | The unique key used to identify your CAPTCHA instance
try {
apiInstance.deleteCaptchaInstance(captchaId);
} catch (ApiException e) {
System.err.println("Exception when calling CaptchaApi#deleteCaptchaInstance");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **captchaId** | **String**| The unique key used to identify your CAPTCHA instance | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deleteOrgCaptchaSettings
> deleteOrgCaptchaSettings()
Delete the Org-wide CAPTCHA Settings
Deletes the CAPTCHA settings object for your organization
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CaptchaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CaptchaApi apiInstance = new CaptchaApi(defaultClient);
try {
apiInstance.deleteOrgCaptchaSettings();
} catch (ApiException e) {
System.err.println("Exception when calling CaptchaApi#deleteOrgCaptchaSettings");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
This endpoint does not need any parameter.
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getCaptchaInstance
> CAPTCHAInstance getCaptchaInstance(captchaId)
Retrieve a CAPTCHA Instance
Retrieves the properties of a specified CAPTCHA instance
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CaptchaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CaptchaApi apiInstance = new CaptchaApi(defaultClient);
String captchaId = "captchaId_example"; // String | The unique key used to identify your CAPTCHA instance
try {
CAPTCHAInstance result = apiInstance.getCaptchaInstance(captchaId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CaptchaApi#getCaptchaInstance");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **captchaId** | **String**| The unique key used to identify your CAPTCHA instance | |
### Return type
[**CAPTCHAInstance**](CAPTCHAInstance.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getOrgCaptchaSettings
> OrgCAPTCHASettings getOrgCaptchaSettings()
Retrieve the Org-wide CAPTCHA Settings
Retrieves the CAPTCHA settings object for your organization > **Note**: If the current organization hasn't configured CAPTCHA Settings, the request returns an empty object.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CaptchaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CaptchaApi apiInstance = new CaptchaApi(defaultClient);
try {
OrgCAPTCHASettings result = apiInstance.getOrgCaptchaSettings();
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CaptchaApi#getOrgCaptchaSettings");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
This endpoint does not need any parameter.
### Return type
[**OrgCAPTCHASettings**](OrgCAPTCHASettings.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## listCaptchaInstances
> List<CAPTCHAInstance> listCaptchaInstances()
List all CAPTCHA Instances
Lists all CAPTCHA instances with pagination support. A subset of CAPTCHA instances can be returned that match a supported filter expression or query.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CaptchaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CaptchaApi apiInstance = new CaptchaApi(defaultClient);
try {
List result = apiInstance.listCaptchaInstances();
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CaptchaApi#listCaptchaInstances");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
This endpoint does not need any parameter.
### Return type
[**List<CAPTCHAInstance>**](CAPTCHAInstance.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## replaceCaptchaInstance
> CAPTCHAInstance replaceCaptchaInstance(captchaId, instance)
Replace a CAPTCHA Instance
Replaces the properties for a specified CAPTCHA instance
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CaptchaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CaptchaApi apiInstance = new CaptchaApi(defaultClient);
String captchaId = "captchaId_example"; // String | The unique key used to identify your CAPTCHA instance
CAPTCHAInstance instance = new CAPTCHAInstance(); // CAPTCHAInstance |
try {
CAPTCHAInstance result = apiInstance.replaceCaptchaInstance(captchaId, instance);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CaptchaApi#replaceCaptchaInstance");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **captchaId** | **String**| The unique key used to identify your CAPTCHA instance | |
| **instance** | [**CAPTCHAInstance**](CAPTCHAInstance.md)| | |
### Return type
[**CAPTCHAInstance**](CAPTCHAInstance.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## replacesOrgCaptchaSettings
> OrgCAPTCHASettings replacesOrgCaptchaSettings(orgCAPTCHASettings)
Replace the Org-wide CAPTCHA Settings
Replaces the CAPTCHA settings object for your organization > **Note**: You can disable CAPTCHA for your organization by setting `captchaId` and `enabledPages` to `null`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CaptchaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CaptchaApi apiInstance = new CaptchaApi(defaultClient);
OrgCAPTCHASettings orgCAPTCHASettings = new OrgCAPTCHASettings(); // OrgCAPTCHASettings |
try {
OrgCAPTCHASettings result = apiInstance.replacesOrgCaptchaSettings(orgCAPTCHASettings);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CaptchaApi#replacesOrgCaptchaSettings");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **orgCAPTCHASettings** | [**OrgCAPTCHASettings**](OrgCAPTCHASettings.md)| | |
### Return type
[**OrgCAPTCHASettings**](OrgCAPTCHASettings.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## updateCaptchaInstance
> CAPTCHAInstance updateCaptchaInstance(captchaId, instance)
Update a CAPTCHA Instance
Partially updates the properties of a specified CAPTCHA instance
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CaptchaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CaptchaApi apiInstance = new CaptchaApi(defaultClient);
String captchaId = "captchaId_example"; // String | The unique key used to identify your CAPTCHA instance
CAPTCHAInstance instance = new CAPTCHAInstance(); // CAPTCHAInstance |
try {
CAPTCHAInstance result = apiInstance.updateCaptchaInstance(captchaId, instance);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CaptchaApi#updateCaptchaInstance");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **captchaId** | **String**| The unique key used to identify your CAPTCHA instance | |
| **instance** | [**CAPTCHAInstance**](CAPTCHAInstance.md)| | |
### Return type
[**CAPTCHAInstance**](CAPTCHAInstance.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy