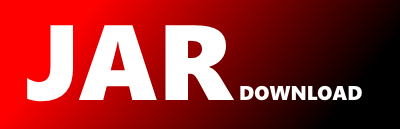
docs.CustomPagesApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# CustomPagesApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**deleteCustomizedErrorPage**](CustomPagesApi.md#deleteCustomizedErrorPage) | **DELETE** /api/v1/brands/{brandId}/pages/error/customized | Delete the Customized Error Page |
| [**deleteCustomizedSignInPage**](CustomPagesApi.md#deleteCustomizedSignInPage) | **DELETE** /api/v1/brands/{brandId}/pages/sign-in/customized | Delete the Customized Sign-in Page |
| [**deletePreviewErrorPage**](CustomPagesApi.md#deletePreviewErrorPage) | **DELETE** /api/v1/brands/{brandId}/pages/error/preview | Delete the Preview Error Page |
| [**deletePreviewSignInPage**](CustomPagesApi.md#deletePreviewSignInPage) | **DELETE** /api/v1/brands/{brandId}/pages/sign-in/preview | Delete the Preview Sign-in Page |
| [**getCustomizedErrorPage**](CustomPagesApi.md#getCustomizedErrorPage) | **GET** /api/v1/brands/{brandId}/pages/error/customized | Retrieve the Customized Error Page |
| [**getCustomizedSignInPage**](CustomPagesApi.md#getCustomizedSignInPage) | **GET** /api/v1/brands/{brandId}/pages/sign-in/customized | Retrieve the Customized Sign-in Page |
| [**getDefaultErrorPage**](CustomPagesApi.md#getDefaultErrorPage) | **GET** /api/v1/brands/{brandId}/pages/error/default | Retrieve the Default Error Page |
| [**getDefaultSignInPage**](CustomPagesApi.md#getDefaultSignInPage) | **GET** /api/v1/brands/{brandId}/pages/sign-in/default | Retrieve the Default Sign-in Page |
| [**getErrorPage**](CustomPagesApi.md#getErrorPage) | **GET** /api/v1/brands/{brandId}/pages/error | Retrieve the Error Page Sub-Resources |
| [**getPreviewErrorPage**](CustomPagesApi.md#getPreviewErrorPage) | **GET** /api/v1/brands/{brandId}/pages/error/preview | Retrieve the Preview Error Page Preview |
| [**getPreviewSignInPage**](CustomPagesApi.md#getPreviewSignInPage) | **GET** /api/v1/brands/{brandId}/pages/sign-in/preview | Retrieve the Preview Sign-in Page Preview |
| [**getSignInPage**](CustomPagesApi.md#getSignInPage) | **GET** /api/v1/brands/{brandId}/pages/sign-in | Retrieve the Sign-in Page Sub-Resources |
| [**getSignOutPageSettings**](CustomPagesApi.md#getSignOutPageSettings) | **GET** /api/v1/brands/{brandId}/pages/sign-out/customized | Retrieve the Sign-out Page Settings |
| [**listAllSignInWidgetVersions**](CustomPagesApi.md#listAllSignInWidgetVersions) | **GET** /api/v1/brands/{brandId}/pages/sign-in/widget-versions | List all Sign-in Widget Versions |
| [**replaceCustomizedErrorPage**](CustomPagesApi.md#replaceCustomizedErrorPage) | **PUT** /api/v1/brands/{brandId}/pages/error/customized | Replace the Customized Error Page |
| [**replaceCustomizedSignInPage**](CustomPagesApi.md#replaceCustomizedSignInPage) | **PUT** /api/v1/brands/{brandId}/pages/sign-in/customized | Replace the Customized Sign-in Page |
| [**replacePreviewErrorPage**](CustomPagesApi.md#replacePreviewErrorPage) | **PUT** /api/v1/brands/{brandId}/pages/error/preview | Replace the Preview Error Page |
| [**replacePreviewSignInPage**](CustomPagesApi.md#replacePreviewSignInPage) | **PUT** /api/v1/brands/{brandId}/pages/sign-in/preview | Replace the Preview Sign-in Page |
| [**replaceSignOutPageSettings**](CustomPagesApi.md#replaceSignOutPageSettings) | **PUT** /api/v1/brands/{brandId}/pages/sign-out/customized | Replace the Sign-out Page Settings |
## deleteCustomizedErrorPage
> deleteCustomizedErrorPage(brandId)
Delete the Customized Error Page
Deletes the customized error page. As a result, the default error page appears in your live environment.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
apiInstance.deleteCustomizedErrorPage(brandId);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#deleteCustomizedErrorPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | Successfully deleted the customized error page. | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deleteCustomizedSignInPage
> deleteCustomizedSignInPage(brandId)
Delete the Customized Sign-in Page
Deletes the customized sign-in page. As a result, the default sign-in page appears in your live environment.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
apiInstance.deleteCustomizedSignInPage(brandId);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#deleteCustomizedSignInPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | Successfully deleted the sign-in page. | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deletePreviewErrorPage
> deletePreviewErrorPage(brandId)
Delete the Preview Error Page
Deletes the preview error page. The preview error page contains unpublished changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/error/preview`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
apiInstance.deletePreviewErrorPage(brandId);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#deletePreviewErrorPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | Successfully deleted the preview error page. | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deletePreviewSignInPage
> deletePreviewSignInPage(brandId)
Delete the Preview Sign-in Page
Deletes the preview sign-in page. The preview sign-in page contains unpublished changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/login/preview`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
apiInstance.deletePreviewSignInPage(brandId);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#deletePreviewSignInPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | Successfully deleted the preview sign-in page. | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getCustomizedErrorPage
> ErrorPage getCustomizedErrorPage(brandId)
Retrieve the Customized Error Page
Retrieves the customized error page. The customized error page appears in your live environment.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
ErrorPage result = apiInstance.getCustomizedErrorPage(brandId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#getCustomizedErrorPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
[**ErrorPage**](ErrorPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully retrieved the customized error page. | * Location -
|
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getCustomizedSignInPage
> SignInPage getCustomizedSignInPage(brandId)
Retrieve the Customized Sign-in Page
Retrieves the customized sign-in page. The customized sign-in page appears in your live environment.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
SignInPage result = apiInstance.getCustomizedSignInPage(brandId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#getCustomizedSignInPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
[**SignInPage**](SignInPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully retrieved the customized sign-in page. | * Location -
|
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getDefaultErrorPage
> ErrorPage getDefaultErrorPage(brandId)
Retrieve the Default Error Page
Retrieves the default error page. The default error page appears when no customized error page exists.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
ErrorPage result = apiInstance.getDefaultErrorPage(brandId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#getDefaultErrorPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
[**ErrorPage**](ErrorPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully retrieved the default error page. | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getDefaultSignInPage
> SignInPage getDefaultSignInPage(brandId)
Retrieve the Default Sign-in Page
Retrieves the default sign-in page. The default sign-in page appears when no customized sign-in page exists.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
SignInPage result = apiInstance.getDefaultSignInPage(brandId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#getDefaultSignInPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
[**SignInPage**](SignInPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully retrieved the default sign-in page. | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getErrorPage
> PageRoot getErrorPage(brandId, expand)
Retrieve the Error Page Sub-Resources
Retrieves the error page sub-resources. The `expand` query parameter specifies which sub-resources to include in the response.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
List expand = Arrays.asList(); // List | Specifies additional metadata to be included in the response
try {
PageRoot result = apiInstance.getErrorPage(brandId, expand);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#getErrorPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **expand** | [**List<String>**](String.md)| Specifies additional metadata to be included in the response | [optional] [enum: default, customized, customizedUrl, preview, previewUrl] |
### Return type
[**PageRoot**](PageRoot.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully retrieved the error page. | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getPreviewErrorPage
> ErrorPage getPreviewErrorPage(brandId)
Retrieve the Preview Error Page Preview
Retrieves the preview error page. The preview error page contains unpublished changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/error/preview`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
ErrorPage result = apiInstance.getPreviewErrorPage(brandId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#getPreviewErrorPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
[**ErrorPage**](ErrorPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully retrieved the preview error page. | * Location -
|
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getPreviewSignInPage
> SignInPage getPreviewSignInPage(brandId)
Retrieve the Preview Sign-in Page Preview
Retrieves the preview sign-in page. The preview sign-in page contains unpublished changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/login/preview`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
SignInPage result = apiInstance.getPreviewSignInPage(brandId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#getPreviewSignInPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
[**SignInPage**](SignInPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully retrieved the preview sign-in page. | * Location -
|
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getSignInPage
> PageRoot getSignInPage(brandId, expand)
Retrieve the Sign-in Page Sub-Resources
Retrieves the sign-in page sub-resources. The `expand` query parameter specifies which sub-resources to include in the response.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
List expand = Arrays.asList(); // List | Specifies additional metadata to be included in the response
try {
PageRoot result = apiInstance.getSignInPage(brandId, expand);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#getSignInPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **expand** | [**List<String>**](String.md)| Specifies additional metadata to be included in the response | [optional] [enum: default, customized, customizedUrl, preview, previewUrl] |
### Return type
[**PageRoot**](PageRoot.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully retrieved the sign-in page. | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getSignOutPageSettings
> HostedPage getSignOutPageSettings(brandId)
Retrieve the Sign-out Page Settings
Retrieves the sign-out page settings
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
HostedPage result = apiInstance.getSignOutPageSettings(brandId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#getSignOutPageSettings");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
[**HostedPage**](HostedPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully retrieved the sign-out page settings. | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listAllSignInWidgetVersions
> List<String> listAllSignInWidgetVersions(brandId)
List all Sign-in Widget Versions
Lists all sign-in widget versions supported by the current org
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
List result = apiInstance.listAllSignInWidgetVersions(brandId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#listAllSignInWidgetVersions");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
**List<String>**
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully listed the sign-in widget versions. | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## replaceCustomizedErrorPage
> ErrorPage replaceCustomizedErrorPage(brandId, errorPage)
Replace the Customized Error Page
Replaces the customized error page. The customized error page appears in your live environment.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
ErrorPage errorPage = new ErrorPage(); // ErrorPage |
try {
ErrorPage result = apiInstance.replaceCustomizedErrorPage(brandId, errorPage);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#replaceCustomizedErrorPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **errorPage** | [**ErrorPage**](ErrorPage.md)| | |
### Return type
[**ErrorPage**](ErrorPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully replaced the customized error page. | * Location -
|
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## replaceCustomizedSignInPage
> SignInPage replaceCustomizedSignInPage(brandId, signInPage)
Replace the Customized Sign-in Page
Replaces the customized sign-in page. The customized sign-in page appears in your live environment.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
SignInPage signInPage = new SignInPage(); // SignInPage |
try {
SignInPage result = apiInstance.replaceCustomizedSignInPage(brandId, signInPage);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#replaceCustomizedSignInPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **signInPage** | [**SignInPage**](SignInPage.md)| | |
### Return type
[**SignInPage**](SignInPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully replaced the customized sign-in page. | * Location -
|
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## replacePreviewErrorPage
> ErrorPage replacePreviewErrorPage(brandId, errorPage)
Replace the Preview Error Page
Replaces the preview error page. The preview error page contains unpublished changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/error/preview`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
ErrorPage errorPage = new ErrorPage(); // ErrorPage |
try {
ErrorPage result = apiInstance.replacePreviewErrorPage(brandId, errorPage);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#replacePreviewErrorPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **errorPage** | [**ErrorPage**](ErrorPage.md)| | |
### Return type
[**ErrorPage**](ErrorPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully replaced the preview error page. | * Location -
|
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## replacePreviewSignInPage
> SignInPage replacePreviewSignInPage(brandId, signInPage)
Replace the Preview Sign-in Page
Replaces the preview sign-in page. The preview sign-in page contains unpublished changes and isn't shown in your live environment. Preview it at `${yourOktaDomain}/login/preview`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
SignInPage signInPage = new SignInPage(); // SignInPage |
try {
SignInPage result = apiInstance.replacePreviewSignInPage(brandId, signInPage);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#replacePreviewSignInPage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **signInPage** | [**SignInPage**](SignInPage.md)| | |
### Return type
[**SignInPage**](SignInPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully replaced the preview sign-in page. | * Location -
|
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## replaceSignOutPageSettings
> HostedPage replaceSignOutPageSettings(brandId, hostedPage)
Replace the Sign-out Page Settings
Replaces the sign-out page settings
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.CustomPagesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
CustomPagesApi apiInstance = new CustomPagesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
HostedPage hostedPage = new HostedPage(); // HostedPage |
try {
HostedPage result = apiInstance.replaceSignOutPageSettings(brandId, hostedPage);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling CustomPagesApi#replaceSignOutPageSettings");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **hostedPage** | [**HostedPage**](HostedPage.md)| | |
### Return type
[**HostedPage**](HostedPage.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully replaced the sign-out page settings. | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy