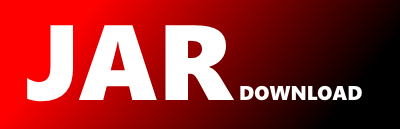
docs.GroupApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# GroupApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**addGroup**](GroupApi.md#addGroup) | **POST** /api/v1/groups | Add a Group |
| [**assignUserToGroup**](GroupApi.md#assignUserToGroup) | **PUT** /api/v1/groups/{groupId}/users/{userId} | Assign a User to a Group |
| [**deleteGroup**](GroupApi.md#deleteGroup) | **DELETE** /api/v1/groups/{groupId} | Delete a Group |
| [**getGroup**](GroupApi.md#getGroup) | **GET** /api/v1/groups/{groupId} | Retrieve a Group |
| [**listAssignedApplicationsForGroup**](GroupApi.md#listAssignedApplicationsForGroup) | **GET** /api/v1/groups/{groupId}/apps | List all Assigned Applications |
| [**listGroupUsers**](GroupApi.md#listGroupUsers) | **GET** /api/v1/groups/{groupId}/users | List all Member Users |
| [**listGroups**](GroupApi.md#listGroups) | **GET** /api/v1/groups | List all Groups |
| [**replaceGroup**](GroupApi.md#replaceGroup) | **PUT** /api/v1/groups/{groupId} | Replace a Group |
| [**unassignUserFromGroup**](GroupApi.md#unassignUserFromGroup) | **DELETE** /api/v1/groups/{groupId}/users/{userId} | Unassign a User from a Group |
## addGroup
> Group addGroup(group)
Add a Group
Adds a new Group with the `OKTA_GROUP` type to your org > **Note:** App import operations are responsible for syncing Groups with `APP_GROUP` type such as Active Directory Groups. See [About groups](https://help.okta.com/okta_help.htm?id=Directory_Groups).
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupApi apiInstance = new GroupApi(defaultClient);
AddGroupRequest group = new AddGroupRequest(); // AddGroupRequest |
try {
Group result = apiInstance.addGroup(group);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupApi#addGroup");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **group** | [**AddGroupRequest**](AddGroupRequest.md)| | |
### Return type
[**Group**](Group.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## assignUserToGroup
> assignUserToGroup(groupId, userId)
Assign a User to a Group
Assigns a User to a Group with the `OKTA_GROUP` type > **Note:** You only can modify memberships for Groups of the `OKTA_GROUP` type. App imports are responsible for managing group memberships for Groups of the `APP_GROUP` type, such as Active Directory groups.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupApi apiInstance = new GroupApi(defaultClient);
String groupId = "00g1emaKYZTWRYYRRTSK"; // String | The `id` of the group
String userId = "00ub0oNGTSWTBKOLGLNR"; // String | ID of an existing Okta user
try {
apiInstance.assignUserToGroup(groupId, userId);
} catch (ApiException e) {
System.err.println("Exception when calling GroupApi#assignUserToGroup");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupId** | **String**| The `id` of the group | |
| **userId** | **String**| ID of an existing Okta user | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deleteGroup
> deleteGroup(groupId)
Delete a Group
Deletes a Group of the `OKTA_GROUP` or `APP_GROUP` type from your org > **Note:** You can't remove Groups of type `APP_GROUP` if they are used in a group push mapping.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupApi apiInstance = new GroupApi(defaultClient);
String groupId = "00g1emaKYZTWRYYRRTSK"; // String | The `id` of the group
try {
apiInstance.deleteGroup(groupId);
} catch (ApiException e) {
System.err.println("Exception when calling GroupApi#deleteGroup");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupId** | **String**| The `id` of the group | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getGroup
> Group getGroup(groupId)
Retrieve a Group
Retrieves a specific Group by `id` from your org
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupApi apiInstance = new GroupApi(defaultClient);
String groupId = "00g1emaKYZTWRYYRRTSK"; // String | The `id` of the group
try {
Group result = apiInstance.getGroup(groupId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupApi#getGroup");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupId** | **String**| The `id` of the group | |
### Return type
[**Group**](Group.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listAssignedApplicationsForGroup
> List<Application> listAssignedApplicationsForGroup(groupId, after, limit)
List all Assigned Applications
Lists all apps that are assigned to a Group. See [Application Groups API](/openapi/okta-management/management/tag/ApplicationGroups/).
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupApi apiInstance = new GroupApi(defaultClient);
String groupId = "00g1emaKYZTWRYYRRTSK"; // String | The `id` of the group
String after = "after_example"; // String | Specifies the pagination cursor for the next page of apps
Integer limit = 20; // Integer | Specifies the number of app results for a page
try {
List result = apiInstance.listAssignedApplicationsForGroup(groupId, after, limit);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupApi#listAssignedApplicationsForGroup");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupId** | **String**| The `id` of the group | |
| **after** | **String**| Specifies the pagination cursor for the next page of apps | [optional] |
| **limit** | **Integer**| Specifies the number of app results for a page | [optional] [default to 20] |
### Return type
[**List<Application>**](Application.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listGroupUsers
> List<User> listGroupUsers(groupId, after, limit)
List all Member Users
Lists all users that are a member of a Group. The default user limit is set to a very high number due to historical reasons that are no longer valid for most orgs. This will change in a future version of this API. The recommended page limit is now `limit=200`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupApi apiInstance = new GroupApi(defaultClient);
String groupId = "00g1emaKYZTWRYYRRTSK"; // String | The `id` of the group
String after = "after_example"; // String | The cursor to use for pagination. It is an opaque string that specifies your current location in the list and is obtained from the `Link` response header. See [Pagination](https://developer.okta.com/docs/api/#pagination).
Integer limit = 1000; // Integer | Specifies the number of user results in a page
try {
List result = apiInstance.listGroupUsers(groupId, after, limit);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupApi#listGroupUsers");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupId** | **String**| The `id` of the group | |
| **after** | **String**| The cursor to use for pagination. It is an opaque string that specifies your current location in the list and is obtained from the `Link` response header. See [Pagination](https://developer.okta.com/docs/api/#pagination). | [optional] |
| **limit** | **Integer**| Specifies the number of user results in a page | [optional] [default to 1000] |
### Return type
[**List<User>**](User.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listGroups
> List<Group> listGroups(q, filter, after, limit, expand, search, sortBy, sortOrder)
List all Groups
Lists all Groups with pagination support. The number of Groups returned depends on the specified [`limit`](/openapi/okta-management/management/tag/Group/#tag/Group/operation/listGroups!in=query&path=limit&t=request), if you have a search, filter, and/or query parameter set, and if that parameter is not null. We recommend using a limit less than or equal to 200. A subset of Groups can be returned that match a supported filter expression, query, or search criteria. > **Note:** Results from the filter or query parameter are driven from an eventually consistent datasource. The synchronization lag is typically less than one second. See [Filtering](https://developer.okta.com/docs/api/#filter) for more information on expressions.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupApi apiInstance = new GroupApi(defaultClient);
String q = "West&limit=10"; // String | Finds a Group that matches the `name` property > **Note:** Paging and searching are currently mutually exclusive. You can't page a query. The default limit for a query is 300 results. Query is intended for an auto-complete picker use case where users refine their search string to constrain the results.
String filter = "id eq \"00g1emaKYZTWRYYRRTSK\""; // String | [Filter expression](https://developer.okta.com/docs/reference/core-okta-api/#filter) for Groups > **Note:** All filters must be [URL encoded](https://developer.mozilla.org/en-US/docs/Glossary/Percent-encoding). For example, `filter=lastUpdated gt \"2013-06-01T00:00:00.000Z\"` is encoded as `filter=lastUpdated%20gt%20%222013-06-01T00:00:00.000Z%22`.
String after = "after_example"; // String | Specifies the pagination cursor for the next page of Groups. The `after` cursor should be treated as an opaque value and obtained through the next link relation. See [Pagination](https://developer.okta.com/docs/api/#pagination).
Integer limit = 56; // Integer | Specifies the number of Group results in a page. Don't write code that depends on the default or maximum value, as it might change. If you receive an `HTTP 500` status code, you likely exceeded the request timeout. Retry your request with a smaller `limit` and [page the results](https://developer.okta.com/docs/api/#pagination). The Okta default Everyone group isn't returned for users with a Group Admin role. >**Note:** We strongly encourage using a limit that's less than or equal to 200. Any number greater than 200 affects performance and accuracy.
String expand = "expand_example"; // String | If specified, additional metadata is included in the response. Possible values are `stats` and `app`.
String search = "type eq \"APP_GROUP\""; // String | Searches for groups with a supported [filtering](https://developer.okta.com/docs/reference/core-okta-api/#filter) expression for all attributes except for `_embedded`, `_links`, and `objectClass`. Search currently performs a `startsWith` match but it should be considered an implementation detail and might change without notice in the future. This operation supports [pagination](https://developer.okta.com/docs/api/#pagination). Using search requires [URL encoding](https://developer.mozilla.org/en-US/docs/Glossary/Percent-encoding), for example, `search=type eq \"OKTA_GROUP\"` is encoded as `search=type+eq+%22OKTA_GROUP%22`. This operation searches many properties: * Any group profile property, including imported app group profile properties. * The top-level properties `id`, `created`, `lastMembershipUpdated`, `lastUpdated`, and `type`. * The [source](/openapi/okta-management/management/tag/Group/#tag/Group/operation/listGroups!c=200&path=_links/source&t=response) of groups with type of `APP_GROUP`, accessed as `source.id`. You can also use `sortBy` and `sortOrder` parameters.
String sortBy = "lastUpdated"; // String | Specifies field to sort by **(for search queries only)**. `sortBy` can be any single property, for example `sortBy=profile.name`.
String sortOrder = "asc"; // String | Specifies sort order: `asc` or `desc` (for search queries only). This parameter is ignored if if `sortBy` is not present. Groups with the same value for the `sortBy` property will be ordered by `id`
try {
List result = apiInstance.listGroups(q, filter, after, limit, expand, search, sortBy, sortOrder);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupApi#listGroups");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **q** | **String**| Finds a Group that matches the `name` property > **Note:** Paging and searching are currently mutually exclusive. You can't page a query. The default limit for a query is 300 results. Query is intended for an auto-complete picker use case where users refine their search string to constrain the results. | [optional] |
| **filter** | **String**| [Filter expression](https://developer.okta.com/docs/reference/core-okta-api/#filter) for Groups > **Note:** All filters must be [URL encoded](https://developer.mozilla.org/en-US/docs/Glossary/Percent-encoding). For example, `filter=lastUpdated gt \"2013-06-01T00:00:00.000Z\"` is encoded as `filter=lastUpdated%20gt%20%222013-06-01T00:00:00.000Z%22`. | [optional] |
| **after** | **String**| Specifies the pagination cursor for the next page of Groups. The `after` cursor should be treated as an opaque value and obtained through the next link relation. See [Pagination](https://developer.okta.com/docs/api/#pagination). | [optional] |
| **limit** | **Integer**| Specifies the number of Group results in a page. Don't write code that depends on the default or maximum value, as it might change. If you receive an `HTTP 500` status code, you likely exceeded the request timeout. Retry your request with a smaller `limit` and [page the results](https://developer.okta.com/docs/api/#pagination). The Okta default Everyone group isn't returned for users with a Group Admin role. >**Note:** We strongly encourage using a limit that's less than or equal to 200. Any number greater than 200 affects performance and accuracy. | [optional] |
| **expand** | **String**| If specified, additional metadata is included in the response. Possible values are `stats` and `app`. | [optional] |
| **search** | **String**| Searches for groups with a supported [filtering](https://developer.okta.com/docs/reference/core-okta-api/#filter) expression for all attributes except for `_embedded`, `_links`, and `objectClass`. Search currently performs a `startsWith` match but it should be considered an implementation detail and might change without notice in the future. This operation supports [pagination](https://developer.okta.com/docs/api/#pagination). Using search requires [URL encoding](https://developer.mozilla.org/en-US/docs/Glossary/Percent-encoding), for example, `search=type eq \"OKTA_GROUP\"` is encoded as `search=type+eq+%22OKTA_GROUP%22`. This operation searches many properties: * Any group profile property, including imported app group profile properties. * The top-level properties `id`, `created`, `lastMembershipUpdated`, `lastUpdated`, and `type`. * The [source](/openapi/okta-management/management/tag/Group/#tag/Group/operation/listGroups!c=200&path=_links/source&t=response) of groups with type of `APP_GROUP`, accessed as `source.id`. You can also use `sortBy` and `sortOrder` parameters. | [optional] |
| **sortBy** | **String**| Specifies field to sort by **(for search queries only)**. `sortBy` can be any single property, for example `sortBy=profile.name`. | [optional] |
| **sortOrder** | **String**| Specifies sort order: `asc` or `desc` (for search queries only). This parameter is ignored if if `sortBy` is not present. Groups with the same value for the `sortBy` property will be ordered by `id` | [optional] [default to asc] |
### Return type
[**List<Group>**](Group.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## replaceGroup
> Group replaceGroup(groupId, group)
Replace a Group
Replaces the profile for a Group of `OKTA_GROUP` type from your org > **Note :** You only can modify profiles for groups of the `OKTA_GROUP` type. > > App imports are responsible for updating profiles for groups of the `APP_GROUP` type, such as Active Directory groups.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupApi apiInstance = new GroupApi(defaultClient);
String groupId = "00g1emaKYZTWRYYRRTSK"; // String | The `id` of the group
AddGroupRequest group = new AddGroupRequest(); // AddGroupRequest |
try {
Group result = apiInstance.replaceGroup(groupId, group);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupApi#replaceGroup");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupId** | **String**| The `id` of the group | |
| **group** | [**AddGroupRequest**](AddGroupRequest.md)| | |
### Return type
[**Group**](Group.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## unassignUserFromGroup
> unassignUserFromGroup(groupId, userId)
Unassign a User from a Group
Unassigns a User from a Group with the `OKTA_GROUP` type > **Note:** You only can modify memberships for groups of the `OKTA_GROUP` type. > > App imports are responsible for managing group memberships for groups of the `APP_GROUP` type, such as Active Directory groups.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupApi apiInstance = new GroupApi(defaultClient);
String groupId = "00g1emaKYZTWRYYRRTSK"; // String | The `id` of the group
String userId = "00ub0oNGTSWTBKOLGLNR"; // String | ID of an existing Okta user
try {
apiInstance.unassignUserFromGroup(groupId, userId);
} catch (ApiException e) {
System.err.println("Exception when calling GroupApi#unassignUserFromGroup");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupId** | **String**| The `id` of the group | |
| **userId** | **String**| ID of an existing Okta user | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy