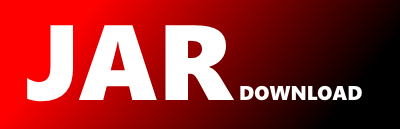
docs.GroupOwnerApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
# GroupOwnerApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**assignGroupOwner**](GroupOwnerApi.md#assignGroupOwner) | **POST** /api/v1/groups/{groupId}/owners | Assign a Group Owner |
| [**deleteGroupOwner**](GroupOwnerApi.md#deleteGroupOwner) | **DELETE** /api/v1/groups/{groupId}/owners/{ownerId} | Delete a Group Owner |
| [**listGroupOwners**](GroupOwnerApi.md#listGroupOwners) | **GET** /api/v1/groups/{groupId}/owners | List all Group Owners |
## assignGroupOwner
> GroupOwner assignGroupOwner(groupId, assignGroupOwnerRequestBody)
Assign a Group Owner
Assigns a group owner
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupOwnerApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupOwnerApi apiInstance = new GroupOwnerApi(defaultClient);
String groupId = "00g1emaKYZTWRYYRRTSK"; // String | The `id` of the group
AssignGroupOwnerRequestBody assignGroupOwnerRequestBody = new AssignGroupOwnerRequestBody(); // AssignGroupOwnerRequestBody |
try {
GroupOwner result = apiInstance.assignGroupOwner(groupId, assignGroupOwnerRequestBody);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupOwnerApi#assignGroupOwner");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupId** | **String**| The `id` of the group | |
| **assignGroupOwnerRequestBody** | [**AssignGroupOwnerRequestBody**](AssignGroupOwnerRequestBody.md)| | |
### Return type
[**GroupOwner**](GroupOwner.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deleteGroupOwner
> deleteGroupOwner(groupId, ownerId)
Delete a Group Owner
Deletes a group owner from a specific group
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupOwnerApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupOwnerApi apiInstance = new GroupOwnerApi(defaultClient);
String groupId = "00g1emaKYZTWRYYRRTSK"; // String | The `id` of the group
String ownerId = "00u1emaK22TWRYd3TtG"; // String | The `id` of the group owner
try {
apiInstance.deleteGroupOwner(groupId, ownerId);
} catch (ApiException e) {
System.err.println("Exception when calling GroupOwnerApi#deleteGroupOwner");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupId** | **String**| The `id` of the group | |
| **ownerId** | **String**| The `id` of the group owner | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listGroupOwners
> List<GroupOwner> listGroupOwners(groupId, search, after, limit)
List all Group Owners
Lists all owners for a specific group
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupOwnerApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupOwnerApi apiInstance = new GroupOwnerApi(defaultClient);
String groupId = "00g1emaKYZTWRYYRRTSK"; // String | The `id` of the group
String search = "search_example"; // String | SCIM Filter expression for group owners. Allows to filter owners by type.
String after = "after_example"; // String | Specifies the pagination cursor for the next page of owners
Integer limit = 1000; // Integer | Specifies the number of owner results in a page
try {
List result = apiInstance.listGroupOwners(groupId, search, after, limit);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupOwnerApi#listGroupOwners");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupId** | **String**| The `id` of the group | |
| **search** | **String**| SCIM Filter expression for group owners. Allows to filter owners by type. | [optional] |
| **after** | **String**| Specifies the pagination cursor for the next page of owners | [optional] |
| **limit** | **Integer**| Specifies the number of owner results in a page | [optional] [default to 1000] |
### Return type
[**List<GroupOwner>**](GroupOwner.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy