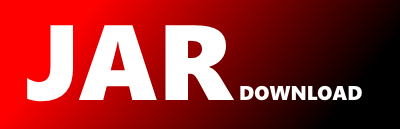
docs.GroupRuleApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# GroupRuleApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**activateGroupRule**](GroupRuleApi.md#activateGroupRule) | **POST** /api/v1/groups/rules/{groupRuleId}/lifecycle/activate | Activate a Group Rule |
| [**createGroupRule**](GroupRuleApi.md#createGroupRule) | **POST** /api/v1/groups/rules | Create a Group rule |
| [**deactivateGroupRule**](GroupRuleApi.md#deactivateGroupRule) | **POST** /api/v1/groups/rules/{groupRuleId}/lifecycle/deactivate | Deactivate a Group Rule |
| [**deleteGroupRule**](GroupRuleApi.md#deleteGroupRule) | **DELETE** /api/v1/groups/rules/{groupRuleId} | Delete a Group Rule |
| [**getGroupRule**](GroupRuleApi.md#getGroupRule) | **GET** /api/v1/groups/rules/{groupRuleId} | Retrieve a Group rule |
| [**listGroupRules**](GroupRuleApi.md#listGroupRules) | **GET** /api/v1/groups/rules | List all Group rules |
| [**replaceGroupRule**](GroupRuleApi.md#replaceGroupRule) | **PUT** /api/v1/groups/rules/{groupRuleId} | Replace a Group rule |
## activateGroupRule
> activateGroupRule(groupRuleId)
Activate a Group Rule
Activates a specific Group rule by ID from your org
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupRuleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupRuleApi apiInstance = new GroupRuleApi(defaultClient);
String groupRuleId = "0pr3f7zMZZHPgUoWO0g4"; // String | The `id` of the group rule
try {
apiInstance.activateGroupRule(groupRuleId);
} catch (ApiException e) {
System.err.println("Exception when calling GroupRuleApi#activateGroupRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupRuleId** | **String**| The `id` of the group rule | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## createGroupRule
> GroupRule createGroupRule(groupRule)
Create a Group rule
Creates a Group rule to dynamically add Users to the specified Group if they match the condition > **Note:** Group rules are created with the status set to `'INACTIVE'`.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupRuleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupRuleApi apiInstance = new GroupRuleApi(defaultClient);
CreateGroupRuleRequest groupRule = new CreateGroupRuleRequest(); // CreateGroupRuleRequest |
try {
GroupRule result = apiInstance.createGroupRule(groupRule);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupRuleApi#createGroupRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupRule** | [**CreateGroupRuleRequest**](CreateGroupRuleRequest.md)| | |
### Return type
[**GroupRule**](GroupRule.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## deactivateGroupRule
> deactivateGroupRule(groupRuleId)
Deactivate a Group Rule
Deactivates a specific Group rule by ID from your org
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupRuleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupRuleApi apiInstance = new GroupRuleApi(defaultClient);
String groupRuleId = "0pr3f7zMZZHPgUoWO0g4"; // String | The `id` of the group rule
try {
apiInstance.deactivateGroupRule(groupRuleId);
} catch (ApiException e) {
System.err.println("Exception when calling GroupRuleApi#deactivateGroupRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupRuleId** | **String**| The `id` of the group rule | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deleteGroupRule
> deleteGroupRule(groupRuleId, removeUsers)
Delete a Group Rule
Deletes a specific group rule by `groupRuleId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupRuleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupRuleApi apiInstance = new GroupRuleApi(defaultClient);
String groupRuleId = "0pr3f7zMZZHPgUoWO0g4"; // String | The `id` of the group rule
Boolean removeUsers = false; // Boolean | If set to `true`, removes Users from Groups assigned by this rule
try {
apiInstance.deleteGroupRule(groupRuleId, removeUsers);
} catch (ApiException e) {
System.err.println("Exception when calling GroupRuleApi#deleteGroupRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupRuleId** | **String**| The `id` of the group rule | |
| **removeUsers** | **Boolean**| If set to `true`, removes Users from Groups assigned by this rule | [optional] [default to false] |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **202** | Accepted | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getGroupRule
> GroupRule getGroupRule(groupRuleId, expand)
Retrieve a Group rule
Retrieves a specific Group rule by ID from your org
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupRuleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupRuleApi apiInstance = new GroupRuleApi(defaultClient);
String groupRuleId = "0pr3f7zMZZHPgUoWO0g4"; // String | The `id` of the group rule
String expand = "expand_example"; // String | If specified as `groupIdToGroupNameMap`, then show Group names
try {
GroupRule result = apiInstance.getGroupRule(groupRuleId, expand);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupRuleApi#getGroupRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupRuleId** | **String**| The `id` of the group rule | |
| **expand** | **String**| If specified as `groupIdToGroupNameMap`, then show Group names | [optional] |
### Return type
[**GroupRule**](GroupRule.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listGroupRules
> List<GroupRule> listGroupRules(limit, after, search, expand)
List all Group rules
Lists all Group rules for your org
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupRuleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupRuleApi apiInstance = new GroupRuleApi(defaultClient);
Integer limit = 50; // Integer | Specifies the number of rule results in a page
String after = "after_example"; // String | Specifies the pagination cursor for the next page of rules
String search = "search_example"; // String | Specifies the keyword to search rules for
String expand = "expand_example"; // String | If specified as `groupIdToGroupNameMap`, then displays group names
try {
List result = apiInstance.listGroupRules(limit, after, search, expand);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupRuleApi#listGroupRules");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **limit** | **Integer**| Specifies the number of rule results in a page | [optional] [default to 50] |
| **after** | **String**| Specifies the pagination cursor for the next page of rules | [optional] |
| **search** | **String**| Specifies the keyword to search rules for | [optional] |
| **expand** | **String**| If specified as `groupIdToGroupNameMap`, then displays group names | [optional] |
### Return type
[**List<GroupRule>**](GroupRule.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## replaceGroupRule
> GroupRule replaceGroupRule(groupRuleId, groupRule)
Replace a Group rule
Replaces a Group rule > **Notes:** You only can update rules with a Group whose status is set to `'INACTIVE'`. > > You currently can't update the `action` section.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.GroupRuleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
GroupRuleApi apiInstance = new GroupRuleApi(defaultClient);
String groupRuleId = "0pr3f7zMZZHPgUoWO0g4"; // String | The `id` of the group rule
GroupRule groupRule = new GroupRule(); // GroupRule |
try {
GroupRule result = apiInstance.replaceGroupRule(groupRuleId, groupRule);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling GroupRuleApi#replaceGroupRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupRuleId** | **String**| The `id` of the group rule | |
| **groupRule** | [**GroupRule**](GroupRule.md)| | |
### Return type
[**GroupRule**](GroupRule.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy