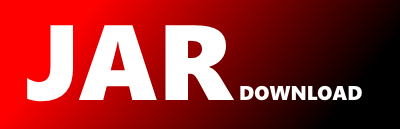
docs.OrgSettingSupportApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# OrgSettingSupportApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**extendOktaSupport**](OrgSettingSupportApi.md#extendOktaSupport) | **POST** /api/v1/org/privacy/oktaSupport/extend | Extend Okta Support Access |
| [**getAerialConsent**](OrgSettingSupportApi.md#getAerialConsent) | **GET** /api/v1/org/privacy/aerial | Retrieve Okta Aerial consent for your Org |
| [**getOrgOktaSupportSettings**](OrgSettingSupportApi.md#getOrgOktaSupportSettings) | **GET** /api/v1/org/privacy/oktaSupport | Retrieve the Okta Support Settings |
| [**grantAerialConsent**](OrgSettingSupportApi.md#grantAerialConsent) | **POST** /api/v1/org/privacy/aerial/grant | Grant Okta Aerial access to your Org |
| [**grantOktaSupport**](OrgSettingSupportApi.md#grantOktaSupport) | **POST** /api/v1/org/privacy/oktaSupport/grant | Grant Okta Support Access |
| [**revokeAerialConsent**](OrgSettingSupportApi.md#revokeAerialConsent) | **POST** /api/v1/org/privacy/aerial/revoke | Revoke Okta Aerial access to your Org |
| [**revokeOktaSupport**](OrgSettingSupportApi.md#revokeOktaSupport) | **POST** /api/v1/org/privacy/oktaSupport/revoke | Revoke Okta Support Access |
## extendOktaSupport
> OrgOktaSupportSettingsObj extendOktaSupport()
Extend Okta Support Access
Extends the length of time that Okta Support can access your org by 24 hours. This means that 24 hours are added to the remaining access time.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.OrgSettingSupportApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
OrgSettingSupportApi apiInstance = new OrgSettingSupportApi(defaultClient);
try {
OrgOktaSupportSettingsObj result = apiInstance.extendOktaSupport();
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling OrgSettingSupportApi#extendOktaSupport");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
This endpoint does not need any parameter.
### Return type
[**OrgOktaSupportSettingsObj**](OrgOktaSupportSettingsObj.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## getAerialConsent
> OrgAerialConsentDetails getAerialConsent()
Retrieve Okta Aerial consent for your Org
Retrieves the Okta Aerial consent grant details for your Org. Returns a 404 Not Found error if no consent has been granted.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.OrgSettingSupportApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
OrgSettingSupportApi apiInstance = new OrgSettingSupportApi(defaultClient);
try {
OrgAerialConsentDetails result = apiInstance.getAerialConsent();
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling OrgSettingSupportApi#getAerialConsent");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
This endpoint does not need any parameter.
### Return type
[**OrgAerialConsentDetails**](OrgAerialConsentDetails.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Can't complete request due to errors | - |
| **403** | Forbidden | - |
| **404** | Consent hasn't been given and there are no grants to any Aerial Accounts | - |
| **429** | Too Many Requests | - |
## getOrgOktaSupportSettings
> OrgOktaSupportSettingsObj getOrgOktaSupportSettings()
Retrieve the Okta Support Settings
Retrieves Okta Support Settings for your org
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.OrgSettingSupportApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
OrgSettingSupportApi apiInstance = new OrgSettingSupportApi(defaultClient);
try {
OrgOktaSupportSettingsObj result = apiInstance.getOrgOktaSupportSettings();
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling OrgSettingSupportApi#getOrgOktaSupportSettings");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
This endpoint does not need any parameter.
### Return type
[**OrgOktaSupportSettingsObj**](OrgOktaSupportSettingsObj.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## grantAerialConsent
> OrgAerialConsentDetails grantAerialConsent(orgAerialConsent)
Grant Okta Aerial access to your Org
Grants an Okta Aerial account access to your Org. If the org is a child org, consent is taken from the parent org. Grant calls directly to the child are not allowed.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.OrgSettingSupportApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
OrgSettingSupportApi apiInstance = new OrgSettingSupportApi(defaultClient);
OrgAerialConsent orgAerialConsent = new OrgAerialConsent(); // OrgAerialConsent |
try {
OrgAerialConsentDetails result = apiInstance.grantAerialConsent(orgAerialConsent);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling OrgSettingSupportApi#grantAerialConsent");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **orgAerialConsent** | [**OrgAerialConsent**](OrgAerialConsent.md)| | [optional] |
### Return type
[**OrgAerialConsentDetails**](OrgAerialConsentDetails.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Can't complete request due to errors | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## grantOktaSupport
> OrgOktaSupportSettingsObj grantOktaSupport()
Grant Okta Support Access
Grants Okta Support temporary access your org as an administrator for eight hours
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.OrgSettingSupportApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
OrgSettingSupportApi apiInstance = new OrgSettingSupportApi(defaultClient);
try {
OrgOktaSupportSettingsObj result = apiInstance.grantOktaSupport();
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling OrgSettingSupportApi#grantOktaSupport");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
This endpoint does not need any parameter.
### Return type
[**OrgOktaSupportSettingsObj**](OrgOktaSupportSettingsObj.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## revokeAerialConsent
> OrgAerialConsentRevoked revokeAerialConsent(orgAerialConsent)
Revoke Okta Aerial access to your Org
Revokes access of an Okta Aerial account to your Org. The revoke operation will fail if the org has already been added to an Aerial account.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.OrgSettingSupportApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
OrgSettingSupportApi apiInstance = new OrgSettingSupportApi(defaultClient);
OrgAerialConsent orgAerialConsent = new OrgAerialConsent(); // OrgAerialConsent |
try {
OrgAerialConsentRevoked result = apiInstance.revokeAerialConsent(orgAerialConsent);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling OrgSettingSupportApi#revokeAerialConsent");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **orgAerialConsent** | [**OrgAerialConsent**](OrgAerialConsent.md)| | [optional] |
### Return type
[**OrgAerialConsentRevoked**](OrgAerialConsentRevoked.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Can't complete request due to errors | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## revokeOktaSupport
> OrgOktaSupportSettingsObj revokeOktaSupport()
Revoke Okta Support Access
Revokes Okta Support access to your org
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.OrgSettingSupportApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
OrgSettingSupportApi apiInstance = new OrgSettingSupportApi(defaultClient);
try {
OrgOktaSupportSettingsObj result = apiInstance.revokeOktaSupport();
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling OrgSettingSupportApi#revokeOktaSupport");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
This endpoint does not need any parameter.
### Return type
[**OrgOktaSupportSettingsObj**](OrgOktaSupportSettingsObj.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy