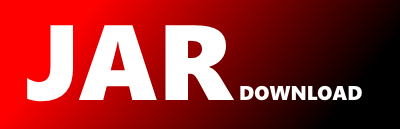
docs.PolicyApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# PolicyApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**activatePolicy**](PolicyApi.md#activatePolicy) | **POST** /api/v1/policies/{policyId}/lifecycle/activate | Activate a Policy |
| [**activatePolicyRule**](PolicyApi.md#activatePolicyRule) | **POST** /api/v1/policies/{policyId}/rules/{ruleId}/lifecycle/activate | Activate a Policy Rule |
| [**clonePolicy**](PolicyApi.md#clonePolicy) | **POST** /api/v1/policies/{policyId}/clone | Clone an existing Policy |
| [**createPolicy**](PolicyApi.md#createPolicy) | **POST** /api/v1/policies | Create a Policy |
| [**createPolicyRule**](PolicyApi.md#createPolicyRule) | **POST** /api/v1/policies/{policyId}/rules | Create a Policy Rule |
| [**createPolicySimulation**](PolicyApi.md#createPolicySimulation) | **POST** /api/v1/policies/simulate | Create a Policy Simulation |
| [**deactivatePolicy**](PolicyApi.md#deactivatePolicy) | **POST** /api/v1/policies/{policyId}/lifecycle/deactivate | Deactivate a Policy |
| [**deactivatePolicyRule**](PolicyApi.md#deactivatePolicyRule) | **POST** /api/v1/policies/{policyId}/rules/{ruleId}/lifecycle/deactivate | Deactivate a Policy Rule |
| [**deletePolicy**](PolicyApi.md#deletePolicy) | **DELETE** /api/v1/policies/{policyId} | Delete a Policy |
| [**deletePolicyResourceMapping**](PolicyApi.md#deletePolicyResourceMapping) | **DELETE** /api/v1/policies/{policyId}/mappings/{mappingId} | Delete a policy resource Mapping |
| [**deletePolicyRule**](PolicyApi.md#deletePolicyRule) | **DELETE** /api/v1/policies/{policyId}/rules/{ruleId} | Delete a Policy Rule |
| [**getPolicy**](PolicyApi.md#getPolicy) | **GET** /api/v1/policies/{policyId} | Retrieve a Policy |
| [**getPolicyMapping**](PolicyApi.md#getPolicyMapping) | **GET** /api/v1/policies/{policyId}/mappings/{mappingId} | Retrieve a policy resource Mapping |
| [**getPolicyRule**](PolicyApi.md#getPolicyRule) | **GET** /api/v1/policies/{policyId}/rules/{ruleId} | Retrieve a Policy Rule |
| [**listPolicies**](PolicyApi.md#listPolicies) | **GET** /api/v1/policies | List all Policies |
| [**listPolicyApps**](PolicyApi.md#listPolicyApps) | **GET** /api/v1/policies/{policyId}/app | List all Applications mapped to a Policy |
| [**listPolicyMappings**](PolicyApi.md#listPolicyMappings) | **GET** /api/v1/policies/{policyId}/mappings | List all resources mapped to a Policy |
| [**listPolicyRules**](PolicyApi.md#listPolicyRules) | **GET** /api/v1/policies/{policyId}/rules | List all Policy Rules |
| [**mapResourceToPolicy**](PolicyApi.md#mapResourceToPolicy) | **POST** /api/v1/policies/{policyId}/mappings | Map a resource to a Policy |
| [**replacePolicy**](PolicyApi.md#replacePolicy) | **PUT** /api/v1/policies/{policyId} | Replace a Policy |
| [**replacePolicyRule**](PolicyApi.md#replacePolicyRule) | **PUT** /api/v1/policies/{policyId}/rules/{ruleId} | Replace a Policy Rule |
## activatePolicy
> activatePolicy(policyId)
Activate a Policy
Activates a policy
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
try {
apiInstance.activatePolicy(policyId);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#activatePolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## activatePolicyRule
> activatePolicyRule(policyId, ruleId)
Activate a Policy Rule
Activates a Policy Rule identified by `policyId` and `ruleId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
String ruleId = "ruld3hJ7jZh4fn0st0g3"; // String | `id` of the Policy Rule
try {
apiInstance.activatePolicyRule(policyId, ruleId);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#activatePolicyRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **ruleId** | **String**| `id` of the Policy Rule | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## clonePolicy
> Policy clonePolicy(policyId)
Clone an existing Policy
Clones an existing policy
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
try {
Policy result = apiInstance.clonePolicy(policyId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#clonePolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
### Return type
[**Policy**](Policy.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## createPolicy
> Policy createPolicy(policy, activate)
Create a Policy
Creates a policy. There are many types of policies that you can create. See [Policies](https://developer.okta.com/docs/concepts/policies/) for an overview of the types of policies available and then links to more indepth information.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
Policy policy = new Policy(); // Policy |
Boolean activate = true; // Boolean | This query parameter is only valid for Classic Engine orgs.
try {
Policy result = apiInstance.createPolicy(policy, activate);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#createPolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policy** | [**Policy**](Policy.md)| | |
| **activate** | **Boolean**| This query parameter is only valid for Classic Engine orgs. | [optional] [default to true] |
### Return type
[**Policy**](Policy.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## createPolicyRule
> PolicyRule createPolicyRule(policyId, policyRule, limit, activate)
Create a Policy Rule
Creates a policy rule > **Note:** You can't create additional rules for the `PROFILE_ENROLLMENT` or `POST_AUTH_SESSION` policies.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
PolicyRule policyRule = new PolicyRule(); // PolicyRule |
String limit = "limit_example"; // String | Defines the number of policy rules returned. See [Pagination](https://developer.okta.com/docs/api/#pagination).
Boolean activate = true; // Boolean | Set this parameter to `false` to create an `INACTIVE` rule.
try {
PolicyRule result = apiInstance.createPolicyRule(policyId, policyRule, limit, activate);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#createPolicyRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **policyRule** | [**PolicyRule**](PolicyRule.md)| | |
| **limit** | **String**| Defines the number of policy rules returned. See [Pagination](https://developer.okta.com/docs/api/#pagination). | [optional] |
| **activate** | **Boolean**| Set this parameter to `false` to create an `INACTIVE` rule. | [optional] [default to true] |
### Return type
[**PolicyRule**](PolicyRule.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## createPolicySimulation
> List<SimulatePolicyEvaluations> createPolicySimulation(simulatePolicy, expand)
Create a Policy Simulation
Creates a policy or policy rule simulation. The access simulation evaluates policy and policy rules based on the existing policy rule configuration. The evaluation result simulates what the real-world authentication flow is and what policy rules have been applied or matched to the authentication flow.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
List simulatePolicy = Arrays.asList(); // List |
String expand = "EVALUATED"; // String | Use `expand=EVALUATED` to include a list of evaluated but not matched policies and policy rules. Use `expand=RULE` to include details about why a rule condition wasn't matched.
try {
List result = apiInstance.createPolicySimulation(simulatePolicy, expand);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#createPolicySimulation");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **simulatePolicy** | [**List<SimulatePolicyBody>**](SimulatePolicyBody.md)| | |
| **expand** | **String**| Use `expand=EVALUATED` to include a list of evaluated but not matched policies and policy rules. Use `expand=RULE` to include details about why a rule condition wasn't matched. | [optional] |
### Return type
[**List<SimulatePolicyEvaluations>**](SimulatePolicyEvaluations.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deactivatePolicy
> deactivatePolicy(policyId)
Deactivate a Policy
Deactivates a policy
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
try {
apiInstance.deactivatePolicy(policyId);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#deactivatePolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deactivatePolicyRule
> deactivatePolicyRule(policyId, ruleId)
Deactivate a Policy Rule
Deactivates a Policy Rule identified by `policyId` and `ruleId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
String ruleId = "ruld3hJ7jZh4fn0st0g3"; // String | `id` of the Policy Rule
try {
apiInstance.deactivatePolicyRule(policyId, ruleId);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#deactivatePolicyRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **ruleId** | **String**| `id` of the Policy Rule | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deletePolicy
> deletePolicy(policyId)
Delete a Policy
Deletes a policy
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
try {
apiInstance.deletePolicy(policyId);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#deletePolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deletePolicyResourceMapping
> deletePolicyResourceMapping(policyId, mappingId)
Delete a policy resource Mapping
Deletes the resource Mapping for a Policy identified by `policyId` and `mappingId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
String mappingId = "maplr2rLjZ6NsGn1P0g3"; // String | `id` of the policy resource Mapping
try {
apiInstance.deletePolicyResourceMapping(policyId, mappingId);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#deletePolicyResourceMapping");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **mappingId** | **String**| `id` of the policy resource Mapping | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deletePolicyRule
> deletePolicyRule(policyId, ruleId)
Delete a Policy Rule
Deletes a Policy Rule identified by `policyId` and `ruleId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
String ruleId = "ruld3hJ7jZh4fn0st0g3"; // String | `id` of the Policy Rule
try {
apiInstance.deletePolicyRule(policyId, ruleId);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#deletePolicyRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **ruleId** | **String**| `id` of the Policy Rule | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getPolicy
> Policy getPolicy(policyId, expand)
Retrieve a Policy
Retrieves a policy
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
String expand = ""; // String |
try {
Policy result = apiInstance.getPolicy(policyId, expand);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#getPolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **expand** | **String**| | [optional] [default to ] |
### Return type
[**Policy**](Policy.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getPolicyMapping
> PolicyMapping getPolicyMapping(policyId, mappingId)
Retrieve a policy resource Mapping
Retrieves a resource Mapping for a Policy identified by `policyId` and `mappingId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
String mappingId = "maplr2rLjZ6NsGn1P0g3"; // String | `id` of the policy resource Mapping
try {
PolicyMapping result = apiInstance.getPolicyMapping(policyId, mappingId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#getPolicyMapping");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **mappingId** | **String**| `id` of the policy resource Mapping | |
### Return type
[**PolicyMapping**](PolicyMapping.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getPolicyRule
> PolicyRule getPolicyRule(policyId, ruleId)
Retrieve a Policy Rule
Retrieves a policy rule
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
String ruleId = "ruld3hJ7jZh4fn0st0g3"; // String | `id` of the Policy Rule
try {
PolicyRule result = apiInstance.getPolicyRule(policyId, ruleId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#getPolicyRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **ruleId** | **String**| `id` of the Policy Rule | |
### Return type
[**PolicyRule**](PolicyRule.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listPolicies
> List<Policy> listPolicies(type, status, q, expand, sortBy, limit, resourceId, after)
List all Policies
Lists all policies with the specified type
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String type = "OKTA_SIGN_ON"; // String | Specifies the type of policy to return. The following policy types are available only with the Okta Identity Engine - `ACCESS_POLICY`, `PROFILE_ENROLLMENT`, `POST_AUTH_SESSION`, and `ENTITY_RISK`. The `POST_AUTH_SESSION` and `ENTITY_RISK` policy types are in . Contact your Okta account team to enable these features.
String status = "status_example"; // String | Refines the query by the `status` of the policy - `ACTIVE` or `INACTIVE`
String q = "q_example"; // String | Refines the query by policy name prefix (startWith method) passed in as `q=string`
String expand = ""; // String |
String sortBy = "sortBy_example"; // String | Refines the query by sorting on the policy `name` in ascending order
String limit = "limit_example"; // String | Defines the number of policies returned, see [Pagination](https://developer.okta.com/docs/api/#pagination)
String resourceId = "resourceId_example"; // String | Reference to the associated authorization server
String after = "after_example"; // String | End page cursor for pagination, see [Pagination](https://developer.okta.com/docs/api/#pagination)
try {
List result = apiInstance.listPolicies(type, status, q, expand, sortBy, limit, resourceId, after);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#listPolicies");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **type** | **String**| Specifies the type of policy to return. The following policy types are available only with the Okta Identity Engine - `ACCESS_POLICY`, `PROFILE_ENROLLMENT`, `POST_AUTH_SESSION`, and `ENTITY_RISK`. The `POST_AUTH_SESSION` and `ENTITY_RISK` policy types are in <x-lifecycle class=\"ea\"></x-lifecycle>. Contact your Okta account team to enable these features. | [enum: OKTA_SIGN_ON, PASSWORD, MFA_ENROLL, IDP_DISCOVERY, ACCESS_POLICY, PROFILE_ENROLLMENT, POST_AUTH_SESSION, ENTITY_RISK] |
| **status** | **String**| Refines the query by the `status` of the policy - `ACTIVE` or `INACTIVE` | [optional] |
| **q** | **String**| Refines the query by policy name prefix (startWith method) passed in as `q=string` | [optional] |
| **expand** | **String**| | [optional] [default to ] |
| **sortBy** | **String**| Refines the query by sorting on the policy `name` in ascending order | [optional] |
| **limit** | **String**| Defines the number of policies returned, see [Pagination](https://developer.okta.com/docs/api/#pagination) | [optional] |
| **resourceId** | **String**| Reference to the associated authorization server | [optional] |
| **after** | **String**| End page cursor for pagination, see [Pagination](https://developer.okta.com/docs/api/#pagination) | [optional] |
### Return type
[**List<Policy>**](Policy.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## listPolicyApps
> List<Application> listPolicyApps(policyId)
List all Applications mapped to a Policy
Lists all applications mapped to a policy identified by `policyId` > **Note:** Use [List all resources mapped to a Policy](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/Policy/#tag/Policy/operation/listPolicyMappings) to list all applications mapped to a policy.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
try {
List result = apiInstance.listPolicyApps(policyId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#listPolicyApps");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
### Return type
[**List<Application>**](Application.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listPolicyMappings
> List<PolicyMapping> listPolicyMappings(policyId)
List all resources mapped to a Policy
Lists all resources mapped to a Policy identified by `policyId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
try {
List result = apiInstance.listPolicyMappings(policyId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#listPolicyMappings");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
### Return type
[**List<PolicyMapping>**](PolicyMapping.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listPolicyRules
> List<PolicyRule> listPolicyRules(policyId, limit)
List all Policy Rules
Lists all policy rules
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
String limit = "limit_example"; // String | Defines the number of policy rules returned. See [Pagination](https://developer.okta.com/docs/api/#pagination).
try {
List result = apiInstance.listPolicyRules(policyId, limit);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#listPolicyRules");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **limit** | **String**| Defines the number of policy rules returned. See [Pagination](https://developer.okta.com/docs/api/#pagination). | [optional] |
### Return type
[**List<PolicyRule>**](PolicyRule.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## mapResourceToPolicy
> PolicyMapping mapResourceToPolicy(policyId, policyMappingRequest)
Map a resource to a Policy
Maps a resource to a Policy identified by `policyId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
PolicyMappingRequest policyMappingRequest = new PolicyMappingRequest(); // PolicyMappingRequest |
try {
PolicyMapping result = apiInstance.mapResourceToPolicy(policyId, policyMappingRequest);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#mapResourceToPolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **policyMappingRequest** | [**PolicyMappingRequest**](PolicyMappingRequest.md)| | |
### Return type
[**PolicyMapping**](PolicyMapping.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## replacePolicy
> Policy replacePolicy(policyId, policy)
Replace a Policy
Replaces the properties of a Policy identified by `policyId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
Policy policy = new Policy(); // Policy |
try {
Policy result = apiInstance.replacePolicy(policyId, policy);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#replacePolicy");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **policy** | [**Policy**](Policy.md)| | |
### Return type
[**Policy**](Policy.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## replacePolicyRule
> PolicyRule replacePolicyRule(policyId, ruleId, policyRule)
Replace a Policy Rule
Replaces the properties for a Policy Rule identified by `policyId` and `ruleId`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.PolicyApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
PolicyApi apiInstance = new PolicyApi(defaultClient);
String policyId = "00plrilJ7jZ66Gn0X0g3"; // String | `id` of the Policy
String ruleId = "ruld3hJ7jZh4fn0st0g3"; // String | `id` of the Policy Rule
PolicyRule policyRule = new PolicyRule(); // PolicyRule |
try {
PolicyRule result = apiInstance.replacePolicyRule(policyId, ruleId, policyRule);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling PolicyApi#replacePolicyRule");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **policyId** | **String**| `id` of the Policy | |
| **ruleId** | **String**| `id` of the Policy Rule | |
| **policyRule** | [**PolicyRule**](PolicyRule.md)| | |
### Return type
[**PolicyRule**](PolicyRule.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy