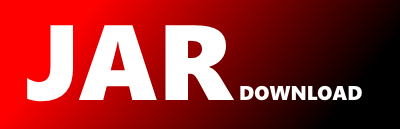
docs.RealmAssignmentApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# RealmAssignmentApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**activateRealmAssignment**](RealmAssignmentApi.md#activateRealmAssignment) | **POST** /api/v1/realm-assignments/{assignmentId}/lifecycle/activate | Activate a Realm Assignment |
| [**createRealmAssignment**](RealmAssignmentApi.md#createRealmAssignment) | **POST** /api/v1/realm-assignments | Create a Realm Assignment |
| [**deactivateRealmAssignment**](RealmAssignmentApi.md#deactivateRealmAssignment) | **POST** /api/v1/realm-assignments/{assignmentId}/lifecycle/deactivate | Deactivate a Realm Assignment |
| [**deleteRealmAssignment**](RealmAssignmentApi.md#deleteRealmAssignment) | **DELETE** /api/v1/realm-assignments/{assignmentId} | Delete a Realm Assignment |
| [**executeRealmAssignment**](RealmAssignmentApi.md#executeRealmAssignment) | **POST** /api/v1/realm-assignments/operations | Execute a Realm Assignment |
| [**getRealmAssignment**](RealmAssignmentApi.md#getRealmAssignment) | **GET** /api/v1/realm-assignments/{assignmentId} | Retrieve a Realm Assignment |
| [**listRealmAssignmentOperations**](RealmAssignmentApi.md#listRealmAssignmentOperations) | **GET** /api/v1/realm-assignments/operations | List all Realm Assignment operations |
| [**listRealmAssignments**](RealmAssignmentApi.md#listRealmAssignments) | **GET** /api/v1/realm-assignments | List all Realm Assignments |
| [**replaceRealmAssignment**](RealmAssignmentApi.md#replaceRealmAssignment) | **PUT** /api/v1/realm-assignments/{assignmentId} | Replace a Realm Assignment |
## activateRealmAssignment
> activateRealmAssignment(assignmentId)
Activate a Realm Assignment
Activates a Realm Assignment
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.RealmAssignmentApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
RealmAssignmentApi apiInstance = new RealmAssignmentApi(defaultClient);
String assignmentId = "rul2jy7jLUlnO3ng00g4"; // String | `id` of the Realm Assignment
try {
apiInstance.activateRealmAssignment(assignmentId);
} catch (ApiException e) {
System.err.println("Exception when calling RealmAssignmentApi#activateRealmAssignment");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **assignmentId** | **String**| `id` of the Realm Assignment | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## createRealmAssignment
> RealmAssignment createRealmAssignment(body)
Create a Realm Assignment
Creates a new Realm Assignment
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.RealmAssignmentApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
RealmAssignmentApi apiInstance = new RealmAssignmentApi(defaultClient);
CreateRealmAssignmentRequest body = new CreateRealmAssignmentRequest(); // CreateRealmAssignmentRequest |
try {
RealmAssignment result = apiInstance.createRealmAssignment(body);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling RealmAssignmentApi#createRealmAssignment");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **body** | [**CreateRealmAssignmentRequest**](CreateRealmAssignmentRequest.md)| | |
### Return type
[**RealmAssignment**](RealmAssignment.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Created | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## deactivateRealmAssignment
> deactivateRealmAssignment(assignmentId)
Deactivate a Realm Assignment
Deactivates a Realm Assignment
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.RealmAssignmentApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
RealmAssignmentApi apiInstance = new RealmAssignmentApi(defaultClient);
String assignmentId = "rul2jy7jLUlnO3ng00g4"; // String | `id` of the Realm Assignment
try {
apiInstance.deactivateRealmAssignment(assignmentId);
} catch (ApiException e) {
System.err.println("Exception when calling RealmAssignmentApi#deactivateRealmAssignment");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **assignmentId** | **String**| `id` of the Realm Assignment | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deleteRealmAssignment
> deleteRealmAssignment(assignmentId)
Delete a Realm Assignment
Deletes a Realm Assignment
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.RealmAssignmentApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
RealmAssignmentApi apiInstance = new RealmAssignmentApi(defaultClient);
String assignmentId = "rul2jy7jLUlnO3ng00g4"; // String | `id` of the Realm Assignment
try {
apiInstance.deleteRealmAssignment(assignmentId);
} catch (ApiException e) {
System.err.println("Exception when calling RealmAssignmentApi#deleteRealmAssignment");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **assignmentId** | **String**| `id` of the Realm Assignment | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## executeRealmAssignment
> OperationResponse executeRealmAssignment(body)
Execute a Realm Assignment
Executes a Realm Assignment
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.RealmAssignmentApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
RealmAssignmentApi apiInstance = new RealmAssignmentApi(defaultClient);
OperationRequest body = new OperationRequest(); // OperationRequest |
try {
OperationResponse result = apiInstance.executeRealmAssignment(body);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling RealmAssignmentApi#executeRealmAssignment");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **body** | [**OperationRequest**](OperationRequest.md)| | |
### Return type
[**OperationResponse**](OperationResponse.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Created | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## getRealmAssignment
> RealmAssignment getRealmAssignment(assignmentId)
Retrieve a Realm Assignment
Retrieves a Realm Assignment
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.RealmAssignmentApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
RealmAssignmentApi apiInstance = new RealmAssignmentApi(defaultClient);
String assignmentId = "rul2jy7jLUlnO3ng00g4"; // String | `id` of the Realm Assignment
try {
RealmAssignment result = apiInstance.getRealmAssignment(assignmentId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling RealmAssignmentApi#getRealmAssignment");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **assignmentId** | **String**| `id` of the Realm Assignment | |
### Return type
[**RealmAssignment**](RealmAssignment.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listRealmAssignmentOperations
> List<OperationResponse> listRealmAssignmentOperations(limit, after)
List all Realm Assignment operations
Lists all Realm Assignment operations. The upper limit is 200 and operations are sorted in descending order from most recent to oldest by id
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.RealmAssignmentApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
RealmAssignmentApi apiInstance = new RealmAssignmentApi(defaultClient);
Integer limit = 20; // Integer | A limit on the number of objects to return
String after = "after_example"; // String | The cursor to use for pagination. It is an opaque string that specifies your current location in the list and is obtained from the `Link` response header. See [Pagination](https://developer.okta.com/docs/api/#pagination).
try {
List result = apiInstance.listRealmAssignmentOperations(limit, after);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling RealmAssignmentApi#listRealmAssignmentOperations");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **limit** | **Integer**| A limit on the number of objects to return | [optional] [default to 20] |
| **after** | **String**| The cursor to use for pagination. It is an opaque string that specifies your current location in the list and is obtained from the `Link` response header. See [Pagination](https://developer.okta.com/docs/api/#pagination). | [optional] |
### Return type
[**List<OperationResponse>**](OperationResponse.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## listRealmAssignments
> List<RealmAssignment> listRealmAssignments(limit, after)
List all Realm Assignments
Lists all Realm Assignments
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.RealmAssignmentApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
RealmAssignmentApi apiInstance = new RealmAssignmentApi(defaultClient);
Integer limit = 20; // Integer | A limit on the number of objects to return
String after = "after_example"; // String | The cursor to use for pagination. It is an opaque string that specifies your current location in the list and is obtained from the `Link` response header. See [Pagination](https://developer.okta.com/docs/api/#pagination).
try {
List result = apiInstance.listRealmAssignments(limit, after);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling RealmAssignmentApi#listRealmAssignments");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **limit** | **Integer**| A limit on the number of objects to return | [optional] [default to 20] |
| **after** | **String**| The cursor to use for pagination. It is an opaque string that specifies your current location in the list and is obtained from the `Link` response header. See [Pagination](https://developer.okta.com/docs/api/#pagination). | [optional] |
### Return type
[**List<RealmAssignment>**](RealmAssignment.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## replaceRealmAssignment
> RealmAssignment replaceRealmAssignment(assignmentId, body)
Replace a Realm Assignment
Replaces a Realm Assignment
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.RealmAssignmentApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
RealmAssignmentApi apiInstance = new RealmAssignmentApi(defaultClient);
String assignmentId = "rul2jy7jLUlnO3ng00g4"; // String | `id` of the Realm Assignment
UpdateRealmAssignmentRequest body = new UpdateRealmAssignmentRequest(); // UpdateRealmAssignmentRequest |
try {
RealmAssignment result = apiInstance.replaceRealmAssignment(assignmentId, body);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling RealmAssignmentApi#replaceRealmAssignment");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **assignmentId** | **String**| `id` of the Realm Assignment | |
| **body** | [**UpdateRealmAssignmentRequest**](UpdateRealmAssignmentRequest.md)| | |
### Return type
[**RealmAssignment**](RealmAssignment.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy