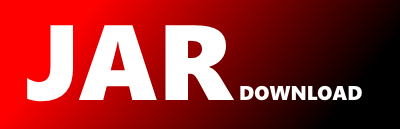
docs.SchemaApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
The newest version!
# SchemaApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**getApplicationUserSchema**](SchemaApi.md#getApplicationUserSchema) | **GET** /api/v1/meta/schemas/apps/{appId}/default | Retrieve the default App User Schema for an App |
| [**getGroupSchema**](SchemaApi.md#getGroupSchema) | **GET** /api/v1/meta/schemas/group/default | Retrieve the default Group Schema |
| [**getLogStreamSchema**](SchemaApi.md#getLogStreamSchema) | **GET** /api/v1/meta/schemas/logStream/{logStreamType} | Retrieve the Log Stream Schema for the schema type |
| [**getUserSchema**](SchemaApi.md#getUserSchema) | **GET** /api/v1/meta/schemas/user/{schemaId} | Retrieve a User Schema |
| [**listLogStreamSchemas**](SchemaApi.md#listLogStreamSchemas) | **GET** /api/v1/meta/schemas/logStream | List the Log Stream Schemas |
| [**updateApplicationUserProfile**](SchemaApi.md#updateApplicationUserProfile) | **POST** /api/v1/meta/schemas/apps/{appId}/default | Update the App User Profile Schema for an App |
| [**updateGroupSchema**](SchemaApi.md#updateGroupSchema) | **POST** /api/v1/meta/schemas/group/default | Update the Group Profile Schema |
| [**updateUserProfile**](SchemaApi.md#updateUserProfile) | **POST** /api/v1/meta/schemas/user/{schemaId} | Update a User Schema |
## getApplicationUserSchema
> UserSchema getApplicationUserSchema(appId)
Retrieve the default App User Schema for an App
Retrieves the default Schema for an App User. The [User Types](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/UserType/) feature does not extend to apps. All users assigned to a given app use the same App User Schema. Therefore, unlike the User Schema operations, the App User Schema operations all specify `default` and don't accept a Schema ID.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SchemaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
SchemaApi apiInstance = new SchemaApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
try {
UserSchema result = apiInstance.getApplicationUserSchema(appId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SchemaApi#getApplicationUserSchema");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
### Return type
[**UserSchema**](UserSchema.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | successful operation | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getGroupSchema
> GroupSchema getGroupSchema()
Retrieve the default Group Schema
Retrieves the Group Schema The [User Types](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/UserType/) feature does not extend to groups. All groups use the same Group Schema. Unlike User Schema operations, Group Schema operations all specify `default` and don't accept a Schema ID.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SchemaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
SchemaApi apiInstance = new SchemaApi(defaultClient);
try {
GroupSchema result = apiInstance.getGroupSchema();
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SchemaApi#getGroupSchema");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
This endpoint does not need any parameter.
### Return type
[**GroupSchema**](GroupSchema.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | successful operation | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## getLogStreamSchema
> LogStreamSchema getLogStreamSchema(logStreamType)
Retrieve the Log Stream Schema for the schema type
Retrieves the Schema for a Log Stream type. The `logStreamType` element in the URL specifies the Log Stream type, which is either `aws_eventbridge` or `splunk_cloud_logstreaming`. Use the `aws_eventbridge` literal to retrieve the AWS EventBridge type schema, and use the `splunk_cloud_logstreaming` literal retrieve the Splunk Cloud type schema.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SchemaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
SchemaApi apiInstance = new SchemaApi(defaultClient);
LogStreamType logStreamType = LogStreamType.fromValue("aws_eventbridge"); // LogStreamType |
try {
LogStreamSchema result = apiInstance.getLogStreamSchema(logStreamType);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SchemaApi#getLogStreamSchema");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **logStreamType** | [**LogStreamType**](.md)| | [enum: aws_eventbridge, splunk_cloud_logstreaming] |
### Return type
[**LogStreamSchema**](LogStreamSchema.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | successful operation | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getUserSchema
> UserSchema getUserSchema(schemaId)
Retrieve a User Schema
Retrieves the Schema for a User Type
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SchemaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
SchemaApi apiInstance = new SchemaApi(defaultClient);
String schemaId = "schemaId_example"; // String |
try {
UserSchema result = apiInstance.getUserSchema(schemaId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SchemaApi#getUserSchema");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **schemaId** | **String**| | |
### Return type
[**UserSchema**](UserSchema.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listLogStreamSchemas
> List<LogStreamSchema> listLogStreamSchemas()
List the Log Stream Schemas
Lists the Schema for all Log Stream types visible for this org
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SchemaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
SchemaApi apiInstance = new SchemaApi(defaultClient);
try {
List result = apiInstance.listLogStreamSchemas();
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SchemaApi#listLogStreamSchemas");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
This endpoint does not need any parameter.
### Return type
[**List<LogStreamSchema>**](LogStreamSchema.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | successful operation | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## updateApplicationUserProfile
> UserSchema updateApplicationUserProfile(appId, body)
Update the App User Profile Schema for an App
Updates the App User Schema. This updates, adds, or removes one or more custom profile properties or the nullability of a base property in the App User Schema for an app. Changing a base property's nullability (for example, the value of its `required` field) is allowed only if it is nullable in the default predefined Schema for the App. The [User Types](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/UserType/) feature does not extend to apps. All users assigned to a given app use the same App User Schema. Therefore, unlike the User Schema operations, the App User Schema operations all specify `default` and don't accept a Schema ID.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SchemaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
SchemaApi apiInstance = new SchemaApi(defaultClient);
String appId = "0oafxqCAJWWGELFTYASJ"; // String | Application ID
UserSchema body = new UserSchema(); // UserSchema |
try {
UserSchema result = apiInstance.updateApplicationUserProfile(appId, body);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SchemaApi#updateApplicationUserProfile");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **appId** | **String**| Application ID | |
| **body** | [**UserSchema**](UserSchema.md)| | [optional] |
### Return type
[**UserSchema**](UserSchema.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | successful operation | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## updateGroupSchema
> GroupSchema updateGroupSchema(groupSchema)
Update the Group Profile Schema
Updates the Group Profile schema. This updates, adds, or removes one or more custom profile properties in a Group Schema. Currently Okta does not support changing base Group Profile properties. The [User Types](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/UserType/) feature does not extend to groups. All groups use the same Group Schema. Unlike User Schema operations, Group Schema operations all specify `default` and don't accept a Schema ID. **Note:** Since POST is interpreted as a partial update, you must set properties explicitly to null to remove them from the Schema.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SchemaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
SchemaApi apiInstance = new SchemaApi(defaultClient);
GroupSchema groupSchema = new GroupSchema(); // GroupSchema |
try {
GroupSchema result = apiInstance.updateGroupSchema(groupSchema);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SchemaApi#updateGroupSchema");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **groupSchema** | [**GroupSchema**](GroupSchema.md)| | [optional] |
### Return type
[**GroupSchema**](GroupSchema.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | successful operation | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## updateUserProfile
> UserSchema updateUserProfile(schemaId, userSchema)
Update a User Schema
Updates a User Schema. This updates, adds, or removes one or more profile properties in a User Schema. Unlike custom User Profile properties, limited changes are allowed to base User Profile properties (permissions, nullability of the `firstName` and `lastName` properties, or pattern for `login`). A property cannot be removed from the default Schema if it is being referenced as a [matchAttribute](https://developer.okta.com/docs/api/openapi/okta-management/management/tag/IdentityProvider/) in SAML2 IdPs. Currently, all validation of SAML assertions is only performed against the default user type.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SchemaApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
SchemaApi apiInstance = new SchemaApi(defaultClient);
String schemaId = "schemaId_example"; // String |
UserSchema userSchema = new UserSchema(); // UserSchema |
try {
UserSchema result = apiInstance.updateUserProfile(schemaId, userSchema);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SchemaApi#updateUserProfile");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **schemaId** | **String**| | |
| **userSchema** | [**UserSchema**](UserSchema.md)| | |
### Return type
[**UserSchema**](UserSchema.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy