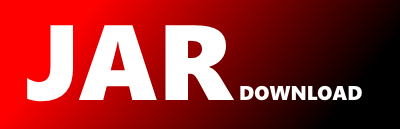
docs.SessionApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# SessionApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**closeCurrentSession**](SessionApi.md#closeCurrentSession) | **DELETE** /api/v1/sessions/me | Close the current Session |
| [**createSession**](SessionApi.md#createSession) | **POST** /api/v1/sessions | Create a Session with session token |
| [**getCurrentSession**](SessionApi.md#getCurrentSession) | **GET** /api/v1/sessions/me | Retrieve the current Session |
| [**getSession**](SessionApi.md#getSession) | **GET** /api/v1/sessions/{sessionId} | Retrieve a Session |
| [**refreshCurrentSession**](SessionApi.md#refreshCurrentSession) | **POST** /api/v1/sessions/me/lifecycle/refresh | Refresh the current Session |
| [**refreshSession**](SessionApi.md#refreshSession) | **POST** /api/v1/sessions/{sessionId}/lifecycle/refresh | Refresh a Session |
| [**revokeSession**](SessionApi.md#revokeSession) | **DELETE** /api/v1/sessions/{sessionId} | Revoke a Session |
## closeCurrentSession
> closeCurrentSession(cookie)
Close the current Session
Closes the Session for the user who is currently signed in. Use this method in a browser-based application to sign out a user. > **Note:** This operation requires a session cookie for the user. An API token isn't allowed for this operation.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SessionApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
SessionApi apiInstance = new SessionApi(defaultClient);
String cookie = "sid=abcde-123 or idx=abcde-123"; // String |
try {
apiInstance.closeCurrentSession(cookie);
} catch (ApiException e) {
System.err.println("Exception when calling SessionApi#closeCurrentSession");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **cookie** | **String**| | [optional] |
### Return type
null (empty response body)
### Authorization
No authorization required
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **404** | Not Found | - |
## createSession
> Session createSession(createSessionRequest)
Create a Session with session token
Creates a new Session for a user with a valid session token. Use this API if, for example, you want to set the session cookie yourself instead of allowing Okta to set it, or want to hold the session ID to delete a session through the API instead of visiting the logout URL.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SessionApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
SessionApi apiInstance = new SessionApi(defaultClient);
CreateSessionRequest createSessionRequest = new CreateSessionRequest(); // CreateSessionRequest |
try {
Session result = apiInstance.createSession(createSessionRequest);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SessionApi#createSession");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **createSessionRequest** | [**CreateSessionRequest**](CreateSessionRequest.md)| | |
### Return type
[**Session**](Session.md)
### Authorization
[apiToken](../README.md#apiToken)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## getCurrentSession
> Session getCurrentSession(cookie)
Retrieve the current Session
Retrieves Session information for the current user. Use this method in a browser-based application to determine if the user is signed in. > **Note:** This operation requires a session cookie for the user. An API token isn't allowed for this operation.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SessionApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
SessionApi apiInstance = new SessionApi(defaultClient);
String cookie = "sid=abcde-123 or idx=abcde-123"; // String |
try {
Session result = apiInstance.getCurrentSession(cookie);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SessionApi#getCurrentSession");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **cookie** | **String**| | [optional] |
### Return type
[**Session**](Session.md)
### Authorization
No authorization required
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **404** | Not Found | - |
## getSession
> Session getSession(sessionId)
Retrieve a Session
Retrieves information about the Session specified by the given session ID
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SessionApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
SessionApi apiInstance = new SessionApi(defaultClient);
String sessionId = "l7FbDVqS8zHSy65uJD85"; // String | `id` of the Session
try {
Session result = apiInstance.getSession(sessionId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SessionApi#getSession");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **sessionId** | **String**| `id` of the Session | |
### Return type
[**Session**](Session.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## refreshCurrentSession
> Session refreshCurrentSession(cookie)
Refresh the current Session
Refreshes the Session for the current user > **Note:** This operation requires a session cookie for the user. An API token isn't allowed for this operation.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SessionApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
SessionApi apiInstance = new SessionApi(defaultClient);
String cookie = "sid=abcde-123 or idx=abcde-123"; // String |
try {
Session result = apiInstance.refreshCurrentSession(cookie);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SessionApi#refreshCurrentSession");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **cookie** | **String**| | [optional] |
### Return type
[**Session**](Session.md)
### Authorization
No authorization required
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **404** | Not Found | - |
## refreshSession
> Session refreshSession(sessionId)
Refresh a Session
Refreshes an existing Session using the `id` for that Session. A successful response contains the refreshed Session with an updated `expiresAt` timestamp.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SessionApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
SessionApi apiInstance = new SessionApi(defaultClient);
String sessionId = "l7FbDVqS8zHSy65uJD85"; // String | `id` of the Session
try {
Session result = apiInstance.refreshSession(sessionId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling SessionApi#refreshSession");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **sessionId** | **String**| `id` of the Session | |
### Return type
[**Session**](Session.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## revokeSession
> revokeSession(sessionId)
Revoke a Session
Revokes the specified Session
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.SessionApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
SessionApi apiInstance = new SessionApi(defaultClient);
String sessionId = "l7FbDVqS8zHSy65uJD85"; // String | `id` of the Session
try {
apiInstance.revokeSession(sessionId);
} catch (ApiException e) {
System.err.println("Exception when calling SessionApi#revokeSession");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **sessionId** | **String**| `id` of the Session | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy