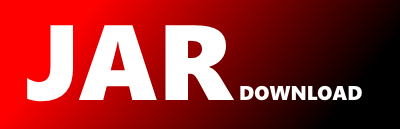
docs.TemplateApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# TemplateApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**createSmsTemplate**](TemplateApi.md#createSmsTemplate) | **POST** /api/v1/templates/sms | Create an SMS Template |
| [**deleteSmsTemplate**](TemplateApi.md#deleteSmsTemplate) | **DELETE** /api/v1/templates/sms/{templateId} | Delete an SMS Template |
| [**getSmsTemplate**](TemplateApi.md#getSmsTemplate) | **GET** /api/v1/templates/sms/{templateId} | Retrieve an SMS Template |
| [**listSmsTemplates**](TemplateApi.md#listSmsTemplates) | **GET** /api/v1/templates/sms | List all SMS Templates |
| [**replaceSmsTemplate**](TemplateApi.md#replaceSmsTemplate) | **PUT** /api/v1/templates/sms/{templateId} | Replace an SMS Template |
| [**updateSmsTemplate**](TemplateApi.md#updateSmsTemplate) | **POST** /api/v1/templates/sms/{templateId} | Update an SMS Template |
## createSmsTemplate
> SmsTemplate createSmsTemplate(smsTemplate)
Create an SMS Template
Creates a new custom SMS template
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.TemplateApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
TemplateApi apiInstance = new TemplateApi(defaultClient);
SmsTemplate smsTemplate = new SmsTemplate(); // SmsTemplate |
try {
SmsTemplate result = apiInstance.createSmsTemplate(smsTemplate);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling TemplateApi#createSmsTemplate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **smsTemplate** | [**SmsTemplate**](SmsTemplate.md)| | |
### Return type
[**SmsTemplate**](SmsTemplate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## deleteSmsTemplate
> deleteSmsTemplate(templateId)
Delete an SMS Template
Deletes an SMS template
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.TemplateApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
TemplateApi apiInstance = new TemplateApi(defaultClient);
String templateId = "6NQUJ5yR3bpgEiYmq8IC"; // String | `id` of the Template
try {
apiInstance.deleteSmsTemplate(templateId);
} catch (ApiException e) {
System.err.println("Exception when calling TemplateApi#deleteSmsTemplate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **templateId** | **String**| `id` of the Template | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getSmsTemplate
> SmsTemplate getSmsTemplate(templateId)
Retrieve an SMS Template
Retrieves a specific template by `id`
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.TemplateApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
TemplateApi apiInstance = new TemplateApi(defaultClient);
String templateId = "6NQUJ5yR3bpgEiYmq8IC"; // String | `id` of the Template
try {
SmsTemplate result = apiInstance.getSmsTemplate(templateId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling TemplateApi#getSmsTemplate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **templateId** | **String**| `id` of the Template | |
### Return type
[**SmsTemplate**](SmsTemplate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listSmsTemplates
> List<SmsTemplate> listSmsTemplates(templateType)
List all SMS Templates
Lists all custom SMS templates. A subset of templates can be returned that match a template type.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.TemplateApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
TemplateApi apiInstance = new TemplateApi(defaultClient);
SmsTemplateType templateType = SmsTemplateType.fromValue("SMS_VERIFY_CODE"); // SmsTemplateType |
try {
List result = apiInstance.listSmsTemplates(templateType);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling TemplateApi#listSmsTemplates");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **templateType** | [**SmsTemplateType**](.md)| | [optional] [enum: SMS_VERIFY_CODE] |
### Return type
[**List<SmsTemplate>**](SmsTemplate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **429** | Too Many Requests | - |
## replaceSmsTemplate
> SmsTemplate replaceSmsTemplate(templateId, smsTemplate)
Replace an SMS Template
Replaces the SMS Template > **Notes:** You can't update the default SMS Template.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.TemplateApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
TemplateApi apiInstance = new TemplateApi(defaultClient);
String templateId = "6NQUJ5yR3bpgEiYmq8IC"; // String | `id` of the Template
SmsTemplate smsTemplate = new SmsTemplate(); // SmsTemplate |
try {
SmsTemplate result = apiInstance.replaceSmsTemplate(templateId, smsTemplate);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling TemplateApi#replaceSmsTemplate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **templateId** | **String**| `id` of the Template | |
| **smsTemplate** | [**SmsTemplate**](SmsTemplate.md)| | |
### Return type
[**SmsTemplate**](SmsTemplate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## updateSmsTemplate
> SmsTemplate updateSmsTemplate(templateId, smsTemplate)
Update an SMS Template
Updates only some of the SMS Template properties: * All properties within the custom SMS Template that have values are updated. * Any translation that doesn't exist is added. * Any translation with a null or empty value is removed. * Any translation with non-empty/null value is updated.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.TemplateApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
TemplateApi apiInstance = new TemplateApi(defaultClient);
String templateId = "6NQUJ5yR3bpgEiYmq8IC"; // String | `id` of the Template
SmsTemplate smsTemplate = new SmsTemplate(); // SmsTemplate |
try {
SmsTemplate result = apiInstance.updateSmsTemplate(templateId, smsTemplate);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling TemplateApi#updateSmsTemplate");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **templateId** | **String**| `id` of the Template | |
| **smsTemplate** | [**SmsTemplate**](SmsTemplate.md)| | |
### Return type
[**SmsTemplate**](SmsTemplate.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy