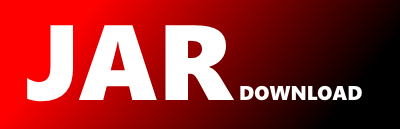
docs.ThemesApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# ThemesApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**deleteBrandThemeBackgroundImage**](ThemesApi.md#deleteBrandThemeBackgroundImage) | **DELETE** /api/v1/brands/{brandId}/themes/{themeId}/background-image | Delete the Background Image |
| [**deleteBrandThemeFavicon**](ThemesApi.md#deleteBrandThemeFavicon) | **DELETE** /api/v1/brands/{brandId}/themes/{themeId}/favicon | Delete the Favicon |
| [**deleteBrandThemeLogo**](ThemesApi.md#deleteBrandThemeLogo) | **DELETE** /api/v1/brands/{brandId}/themes/{themeId}/logo | Delete the Logo |
| [**getBrandTheme**](ThemesApi.md#getBrandTheme) | **GET** /api/v1/brands/{brandId}/themes/{themeId} | Retrieve a Theme |
| [**listBrandThemes**](ThemesApi.md#listBrandThemes) | **GET** /api/v1/brands/{brandId}/themes | List all Themes |
| [**replaceBrandTheme**](ThemesApi.md#replaceBrandTheme) | **PUT** /api/v1/brands/{brandId}/themes/{themeId} | Replace a Theme |
| [**uploadBrandThemeBackgroundImage**](ThemesApi.md#uploadBrandThemeBackgroundImage) | **POST** /api/v1/brands/{brandId}/themes/{themeId}/background-image | Upload the Background Image |
| [**uploadBrandThemeFavicon**](ThemesApi.md#uploadBrandThemeFavicon) | **POST** /api/v1/brands/{brandId}/themes/{themeId}/favicon | Upload the Favicon |
| [**uploadBrandThemeLogo**](ThemesApi.md#uploadBrandThemeLogo) | **POST** /api/v1/brands/{brandId}/themes/{themeId}/logo | Upload the Logo |
## deleteBrandThemeBackgroundImage
> deleteBrandThemeBackgroundImage(brandId, themeId)
Delete the Background Image
Deletes a Theme background image
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ThemesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ThemesApi apiInstance = new ThemesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
String themeId = "themeId_example"; // String | The ID of the theme
try {
apiInstance.deleteBrandThemeBackgroundImage(brandId, themeId);
} catch (ApiException e) {
System.err.println("Exception when calling ThemesApi#deleteBrandThemeBackgroundImage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **themeId** | **String**| The ID of the theme | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deleteBrandThemeFavicon
> deleteBrandThemeFavicon(brandId, themeId)
Delete the Favicon
Deletes a Theme favicon. The theme will use the default Okta favicon.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ThemesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ThemesApi apiInstance = new ThemesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
String themeId = "themeId_example"; // String | The ID of the theme
try {
apiInstance.deleteBrandThemeFavicon(brandId, themeId);
} catch (ApiException e) {
System.err.println("Exception when calling ThemesApi#deleteBrandThemeFavicon");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **themeId** | **String**| The ID of the theme | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deleteBrandThemeLogo
> deleteBrandThemeLogo(brandId, themeId)
Delete the Logo
Deletes a Theme logo. The theme will use the default Okta logo.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ThemesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ThemesApi apiInstance = new ThemesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
String themeId = "themeId_example"; // String | The ID of the theme
try {
apiInstance.deleteBrandThemeLogo(brandId, themeId);
} catch (ApiException e) {
System.err.println("Exception when calling ThemesApi#deleteBrandThemeLogo");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **themeId** | **String**| The ID of the theme | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## getBrandTheme
> ThemeResponse getBrandTheme(brandId, themeId)
Retrieve a Theme
Retrieves a theme for a brand
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ThemesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ThemesApi apiInstance = new ThemesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
String themeId = "themeId_example"; // String | The ID of the theme
try {
ThemeResponse result = apiInstance.getBrandTheme(brandId, themeId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ThemesApi#getBrandTheme");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **themeId** | **String**| The ID of the theme | |
### Return type
[**ThemeResponse**](ThemeResponse.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully retrieved the theme | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## listBrandThemes
> List<ThemeResponse> listBrandThemes(brandId)
List all Themes
Lists all the themes in your brand. > **Important:** Currently each org supports only one Theme, therefore this contains a single object only.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ThemesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ThemesApi apiInstance = new ThemesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
try {
List result = apiInstance.listBrandThemes(brandId);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ThemesApi#listBrandThemes");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
### Return type
[**List<ThemeResponse>**](ThemeResponse.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully returned the list of themes | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## replaceBrandTheme
> ThemeResponse replaceBrandTheme(brandId, themeId, theme)
Replace a Theme
Replaces a theme for a brand
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ThemesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ThemesApi apiInstance = new ThemesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
String themeId = "themeId_example"; // String | The ID of the theme
UpdateThemeRequest theme = new UpdateThemeRequest(); // UpdateThemeRequest |
try {
ThemeResponse result = apiInstance.replaceBrandTheme(brandId, themeId, theme);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ThemesApi#replaceBrandTheme");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **themeId** | **String**| The ID of the theme | |
| **theme** | [**UpdateThemeRequest**](UpdateThemeRequest.md)| | |
### Return type
[**ThemeResponse**](ThemeResponse.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Successfully replaced the theme | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## uploadBrandThemeBackgroundImage
> ImageUploadResponse uploadBrandThemeBackgroundImage(brandId, themeId, _file)
Upload the Background Image
Uploads and replaces the background image for the theme. The file must be in PNG, JPG, or GIF format and less than 2 MB in size.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ThemesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ThemesApi apiInstance = new ThemesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
String themeId = "themeId_example"; // String | The ID of the theme
File _file = new File("/path/to/file"); // File |
try {
ImageUploadResponse result = apiInstance.uploadBrandThemeBackgroundImage(brandId, themeId, _file);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ThemesApi#uploadBrandThemeBackgroundImage");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **themeId** | **String**| The ID of the theme | |
| **_file** | **File**| | |
### Return type
[**ImageUploadResponse**](ImageUploadResponse.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: multipart/form-data
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Content Created | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## uploadBrandThemeFavicon
> ImageUploadResponse uploadBrandThemeFavicon(brandId, themeId, _file)
Upload the Favicon
Uploads and replaces the favicon for the theme
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ThemesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ThemesApi apiInstance = new ThemesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
String themeId = "themeId_example"; // String | The ID of the theme
File _file = new File("/path/to/file"); // File |
try {
ImageUploadResponse result = apiInstance.uploadBrandThemeFavicon(brandId, themeId, _file);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ThemesApi#uploadBrandThemeFavicon");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **themeId** | **String**| The ID of the theme | |
| **_file** | **File**| | |
### Return type
[**ImageUploadResponse**](ImageUploadResponse.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: multipart/form-data
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **201** | Created | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## uploadBrandThemeLogo
> ImageUploadResponse uploadBrandThemeLogo(brandId, themeId, _file)
Upload the Logo
Uploads and replaces the logo for the theme. The file must be in PNG, JPG, or GIF format and less than 100kB in size. For best results use landscape orientation, a transparent background, and a minimum size of 300px by 50px to prevent upscaling.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.ThemesApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
ThemesApi apiInstance = new ThemesApi(defaultClient);
String brandId = "brandId_example"; // String | The ID of the brand
String themeId = "themeId_example"; // String | The ID of the theme
File _file = new File("/path/to/file"); // File |
try {
ImageUploadResponse result = apiInstance.uploadBrandThemeLogo(brandId, themeId, _file);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ThemesApi#uploadBrandThemeLogo");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **brandId** | **String**| The ID of the brand | |
| **themeId** | **String**| The ID of the theme | |
| **_file** | **File**| | |
### Return type
[**ImageUploadResponse**](ImageUploadResponse.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: multipart/form-data
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy