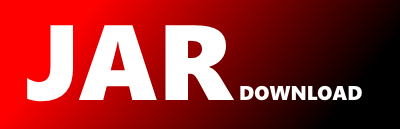
docs.UserLifecycleApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# UserLifecycleApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**activateUser**](UserLifecycleApi.md#activateUser) | **POST** /api/v1/users/{userId}/lifecycle/activate | Activate a User |
| [**deactivateUser**](UserLifecycleApi.md#deactivateUser) | **POST** /api/v1/users/{userId}/lifecycle/deactivate | Deactivate a User |
| [**reactivateUser**](UserLifecycleApi.md#reactivateUser) | **POST** /api/v1/users/{id}/lifecycle/reactivate | Reactivate a User |
| [**resetFactors**](UserLifecycleApi.md#resetFactors) | **POST** /api/v1/users/{userId}/lifecycle/reset_factors | Reset Factors |
| [**suspendUser**](UserLifecycleApi.md#suspendUser) | **POST** /api/v1/users/{userId}/lifecycle/suspend | Suspend a User |
| [**unlockUser**](UserLifecycleApi.md#unlockUser) | **POST** /api/v1/users/{userId}/lifecycle/unlock | Unlock a User |
| [**unsuspendUser**](UserLifecycleApi.md#unsuspendUser) | **POST** /api/v1/users/{userId}/lifecycle/unsuspend | Unsuspend a User |
## activateUser
> UserActivationToken activateUser(userId, sendEmail)
Activate a User
Activates a User. This operation can only be performed on Users with a `STAGED` or `DEPROVISIONED` status. Activation of a User is an asynchronous operation. * The User will have the `transitioningToStatus` property with an `ACTIVE` value during activation to indicate that the user hasn't completed the asynchronous operation. * The User will have an `ACTIVE` status when the activation process completes. Users who don't have a password must complete the welcome flow by visiting the activation link to complete the transition to `ACTIVE` status. > **Note:** If you want to send a branded User Activation email, change the subdomain of your request to the custom domain that's associated with the brand. > For example, change `subdomain.okta.com` to `custom.domain.one`. See [Multibrand and custom domains](https://developer.okta.com/docs/concepts/brands/#multibrand-and-custom-domains). > **Note:** If you have Optional Password enabled, visiting the activation link is optional for users who aren't required to enroll a password. > See [Create user with Optional Password enabled](https://developer.okta.com/docs/reference/api/users/#create-user-with-optional-password-enabled). > **Legal disclaimer** > After a user is added to the Okta directory, they receive an activation email. As part of signing up for this service, > you agreed not to use Okta's service/product to spam and/or send unsolicited messages. > Please refrain from adding unrelated accounts to the directory as Okta is not responsible for, and disclaims any and all > liability associated with, the activation email's content. You, and you alone, bear responsibility for the emails sent to any recipients.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.UserLifecycleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
UserLifecycleApi apiInstance = new UserLifecycleApi(defaultClient);
String userId = "00ub0oNGTSWTBKOLGLNR"; // String | ID of an existing Okta user
Boolean sendEmail = true; // Boolean | Sends an activation email to the user if `true`
try {
UserActivationToken result = apiInstance.activateUser(userId, sendEmail);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling UserLifecycleApi#activateUser");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **userId** | **String**| ID of an existing Okta user | |
| **sendEmail** | **Boolean**| Sends an activation email to the user if `true` | [optional] [default to true] |
### Return type
[**UserActivationToken**](UserActivationToken.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## deactivateUser
> deactivateUser(userId, sendEmail, prefer)
Deactivate a User
Deactivates a User. This operation can only be performed on Users that do not have a `DEPROVISIONED` status. * The User's `transitioningToStatus` property is `DEPROVISIONED` during deactivation to indicate that the user hasn't completed the asynchronous operation. * The User's status is `DEPROVISIONED` when the deactivation process is complete. > **Important:** Deactivating a User is a **destructive** operation. The User is deprovisioned from all assigned apps, which might destroy their data such as email or files. **This action cannot be recovered!** You can also perform user deactivation asynchronously. To invoke asynchronous user deactivation, pass an HTTP header `Prefer: respond-async` with the request.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.UserLifecycleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
UserLifecycleApi apiInstance = new UserLifecycleApi(defaultClient);
String userId = "00ub0oNGTSWTBKOLGLNR"; // String | ID of an existing Okta user
Boolean sendEmail = false; // Boolean | Sends a deactivation email to the admin if `true`
String prefer = "respond-async"; // String | Request asynchronous processing
try {
apiInstance.deactivateUser(userId, sendEmail, prefer);
} catch (ApiException e) {
System.err.println("Exception when calling UserLifecycleApi#deactivateUser");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **userId** | **String**| ID of an existing Okta user | |
| **sendEmail** | **Boolean**| Sends a deactivation email to the admin if `true` | [optional] [default to false] |
| **prefer** | **String**| Request asynchronous processing | [optional] [enum: respond-async] |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## reactivateUser
> UserActivationToken reactivateUser(id, sendEmail)
Reactivate a User
Reactivates a user. This operation can only be performed on Users with a `PROVISIONED` or `RECOVERY` [status](/openapi/okta-management/management/tag/User/#tag/User/operation/listUsers!c=200&path=status&t=response). This operation restarts the activation workflow if for some reason the user activation wasn't completed when using the `activationToken` from [Activate User](/openapi/okta-management/management/tag/UserLifecycle/#tag/UserLifecycle/operation/activateUser). Users that don't have a password must complete the flow by completing [Reset Password](/openapi/okta-management/management/tag/UserCred/#tag/UserCred/operation/resetPassword) and MFA enrollment steps to transition the user to `ACTIVE` status. If `sendEmail` is `false`, returns an activation link for the user to set up their account. The activation token can be used to create a custom activation link.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.UserLifecycleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
UserLifecycleApi apiInstance = new UserLifecycleApi(defaultClient);
String id = "id_example"; // String | `id`, `login`, or `login shortname` (as long as it is unambiguous) of user
Boolean sendEmail = false; // Boolean | Sends an activation email to the user if `true`
try {
UserActivationToken result = apiInstance.reactivateUser(id, sendEmail);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling UserLifecycleApi#reactivateUser");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **id** | **String**| `id`, `login`, or `login shortname` (as long as it is unambiguous) of user | |
| **sendEmail** | **Boolean**| Sends an activation email to the user if `true` | [optional] [default to false] |
### Return type
[**UserActivationToken**](UserActivationToken.md)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## resetFactors
> resetFactors(userId)
Reset Factors
Resets all factors for the specified User. All MFA factor enrollments return to the unenrolled state. The User's status remains `ACTIVE`. This link is present only if the User is currently enrolled in one or more MFA factors.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.UserLifecycleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
UserLifecycleApi apiInstance = new UserLifecycleApi(defaultClient);
String userId = "00ub0oNGTSWTBKOLGLNR"; // String | ID of an existing Okta user
try {
apiInstance.resetFactors(userId);
} catch (ApiException e) {
System.err.println("Exception when calling UserLifecycleApi#resetFactors");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **userId** | **String**| ID of an existing Okta user | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## suspendUser
> suspendUser(userId)
Suspend a User
Suspends a user. This operation can only be performed on Users with an `ACTIVE` status. The User has a `SUSPENDED` status when the process completes. Suspended users can't sign in to Okta. They can only be unsuspended or deactivated. Their group and app assignments are retained.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.UserLifecycleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
UserLifecycleApi apiInstance = new UserLifecycleApi(defaultClient);
String userId = "00ub0oNGTSWTBKOLGLNR"; // String | ID of an existing Okta user
try {
apiInstance.suspendUser(userId);
} catch (ApiException e) {
System.err.println("Exception when calling UserLifecycleApi#suspendUser");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **userId** | **String**| ID of an existing Okta user | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## unlockUser
> unlockUser(userId)
Unlock a User
Unlocks a User with a `LOCKED_OUT` status or unlocks a User with an `ACTIVE` status that is blocked from unknown devices. Unlocked Users have an `ACTIVE` status and can sign in with their current password. > **Note:** This operation works with Okta-sourced users. It doesn't support directory-sourced accounts such as Active Directory.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.UserLifecycleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
UserLifecycleApi apiInstance = new UserLifecycleApi(defaultClient);
String userId = "00ub0oNGTSWTBKOLGLNR"; // String | ID of an existing Okta user
try {
apiInstance.unlockUser(userId);
} catch (ApiException e) {
System.err.println("Exception when calling UserLifecycleApi#unlockUser");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **userId** | **String**| ID of an existing Okta user | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## unsuspendUser
> unsuspendUser(userId)
Unsuspend a User
Unsuspends a user and returns them to the `ACTIVE` state. This operation can only be performed on users that have a `SUSPENDED` status.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.UserLifecycleApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
UserLifecycleApi apiInstance = new UserLifecycleApi(defaultClient);
String userId = "00ub0oNGTSWTBKOLGLNR"; // String | ID of an existing Okta user
try {
apiInstance.unsuspendUser(userId);
} catch (ApiException e) {
System.err.println("Exception when calling UserLifecycleApi#unsuspendUser");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **userId** | **String**| ID of an existing Okta user | |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | Success | - |
| **400** | Bad Request | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy