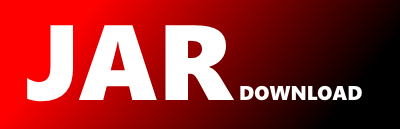
docs.UserSessionsApi.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of okta-sdk-api Show documentation
Show all versions of okta-sdk-api Show documentation
The Okta Java SDK API .jar provides a Java API that your code can use to make calls to the Okta
API. This .jar is the only compile-time dependency within the Okta SDK project that your code should
depend on. Implementations of this API (implementation .jars) should be runtime dependencies only.
# UserSessionsApi
All URIs are relative to *https://subdomain.okta.com*
| Method | HTTP request | Description |
|------------- | ------------- | -------------|
| [**endUserSessions**](UserSessionsApi.md#endUserSessions) | **POST** /api/v1/users/me/lifecycle/delete_sessions | End a current User session |
| [**revokeUserSessions**](UserSessionsApi.md#revokeUserSessions) | **DELETE** /api/v1/users/{userId}/sessions | Revoke all User sessions |
## endUserSessions
> endUserSessions(keepCurrent)
End a current User session
Ends Okta sessions for the currently signed in User. By default, the current session remains active. Use this method in a browser-based app. > **Note:** This operation requires a session cookie for the User. The API token isn't allowed for this operation.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.UserSessionsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
UserSessionsApi apiInstance = new UserSessionsApi(defaultClient);
KeepCurrent keepCurrent = new KeepCurrent(); // KeepCurrent |
try {
apiInstance.endUserSessions(keepCurrent);
} catch (ApiException e) {
System.err.println("Exception when calling UserSessionsApi#endUserSessions");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **keepCurrent** | [**KeepCurrent**](KeepCurrent.md)| | [optional] |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: application/json
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **200** | OK | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
## revokeUserSessions
> revokeUserSessions(userId, oauthTokens)
Revoke all User sessions
Revokes all active Identity Provider sessions of the User. This forces the user to authenticate on the next operation. Optionally revokes OpenID Connect and OAuth refresh and access tokens issued to the User. > **Note:** This operation doesn't clear the sessions created for web or native apps.
### Example
```java
// Import classes:
import com.okta.sdk.resource.client.ApiClient;
import com.okta.sdk.resource.client.ApiException;
import com.okta.sdk.resource.client.Configuration;
import com.okta.sdk.resource.client.auth.*;
import com.okta.sdk.resource.client.models.*;
import com.okta.sdk.resource.api.UserSessionsApi;
public class Example {
public static void main(String[] args) {
ApiClient defaultClient = Configuration.getDefaultApiClient();
defaultClient.setBasePath("https://subdomain.okta.com");
// Configure API key authorization: apiToken
ApiKeyAuth apiToken = (ApiKeyAuth) defaultClient.getAuthentication("apiToken");
apiToken.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiToken.setApiKeyPrefix("Token");
// Configure OAuth2 access token for authorization: oauth2
OAuth oauth2 = (OAuth) defaultClient.getAuthentication("oauth2");
oauth2.setAccessToken("YOUR ACCESS TOKEN");
UserSessionsApi apiInstance = new UserSessionsApi(defaultClient);
String userId = "00ub0oNGTSWTBKOLGLNR"; // String | ID of an existing Okta user
Boolean oauthTokens = false; // Boolean | Revoke issued OpenID Connect and OAuth refresh and access tokens
try {
apiInstance.revokeUserSessions(userId, oauthTokens);
} catch (ApiException e) {
System.err.println("Exception when calling UserSessionsApi#revokeUserSessions");
System.err.println("Status code: " + e.getCode());
System.err.println("Reason: " + e.getResponseBody());
System.err.println("Response headers: " + e.getResponseHeaders());
e.printStackTrace();
}
}
}
```
### Parameters
| Name | Type | Description | Notes |
|------------- | ------------- | ------------- | -------------|
| **userId** | **String**| ID of an existing Okta user | |
| **oauthTokens** | **Boolean**| Revoke issued OpenID Connect and OAuth refresh and access tokens | [optional] [default to false] |
### Return type
null (empty response body)
### Authorization
[apiToken](../README.md#apiToken), [oauth2](../README.md#oauth2)
### HTTP request headers
- **Content-Type**: Not defined
- **Accept**: application/json
### HTTP response details
| Status code | Description | Response headers |
|-------------|-------------|------------------|
| **204** | No Content | - |
| **403** | Forbidden | - |
| **404** | Not Found | - |
| **429** | Too Many Requests | - |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy