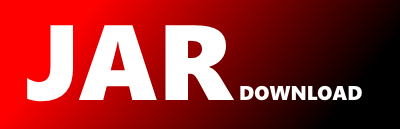
com.okworx.ilcd.validation.AbstractReferenceObjectsAwareValidator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ilcd-validation Show documentation
Show all versions of ilcd-validation Show documentation
A Java library for performing technical validation of data in ILCD data format.
package com.okworx.ilcd.validation;
import java.io.*;
import java.net.URL;
import java.util.HashMap;
import java.util.zip.GZIPInputStream;
import org.apache.commons.lang3.BooleanUtils;
import com.okworx.ilcd.validation.profile.Profile;
import com.okworx.ilcd.validation.util.PrefixBuilder;
/**
* Abstract AbstractReferenceObjectsAwareValidator class.
*
* @author oliver.kusche
* @version $Id: $Id
*/
public abstract class AbstractReferenceObjectsAwareValidator extends AbstractDatasetsValidator {
/** Constant PARAM_IGNORE_REFERENCE_OBJECTS="ignoreReferenceObjects"
*/
public static final String PARAM_IGNORE_REFERENCE_OBJECTS = "ignoreReferenceObjects";
public static final String ATTRIBUTE_TYPE = "type";
public static final String ATTRIBUTE_NAME = "name";
public static final String ATTRIBUTE_ORIGIN = "origin";
public static final String ATTRIBUTE_URI = "uri";
public static final String ATTRIBUTE_REF_OBJECT_ID = "refObjectId";
public static final String URL_PREFIX_HTTPS = "https://";
public static final String URL_PREFIX_HTTP = "http://";
protected HashMap referenceElementaryFlows = new HashMap();
protected HashMap referenceObjectsOther = new HashMap();
protected AbstractReferenceObjectsAwareValidator() {
this.parameters.put(PARAM_IGNORE_REFERENCE_OBJECTS, true);
}
@Override
public boolean validate() throws InterruptedException {
super.validate();
boolean skipReferenceObjects = BooleanUtils.isTrue((Boolean) this.parameters.get(PARAM_IGNORE_REFERENCE_OBJECTS));
log.debug("skipping reference objects: {}", skipReferenceObjects);
if (skipReferenceObjects) {
// remove all elements that are reference objects from objects to validate
for (String key : referenceElementaryFlows.keySet()) {
this.objectsToValidate.remove(key);
}
for (String key : referenceObjectsOther.keySet()) {
this.objectsToValidate.remove(key);
}
}
return false;
}
/** {@inheritDoc} */
@Override
public void setProfile(Profile profile) {
super.setProfile(profile);
log.debug("profile is null: " + (profile == null));
if ( profile != null ) {
log.debug("getReferenceElementaryFlows is null: " + (profile.getReferenceElementaryFlows() == null));
if (profile.getReferenceElementaryFlows() != null)
setupReferenceElementaryFlows(profile.getReferenceElementaryFlows());
log.debug("getReferenceObjectsOther is null: " + (profile.getReferenceObjectsOther() == null));
if (profile.getReferenceObjectsOther() != null)
setupReferenceObjectsOther(profile.getReferenceObjectsOther());
}
}
/**
* Setter for the field referenceElementaryFlows
.
*
* @param map a {@link java.util.HashMap} object.
*/
public void setReferenceElementaryFlows(final HashMap map) {
this.referenceElementaryFlows = map;
}
/**
* Getter for the field referenceElementaryFlows
.
*
* @return a {@link java.util.HashMap} object.
*/
public HashMap getReferenceElementaryFlows() {
return this.referenceElementaryFlows;
}
/**
* Getter for the field referenceObjectsOther
.
*
* @return a {@link java.util.HashMap} object.
*/
public HashMap getReferenceObjectsOther() {
return referenceObjectsOther;
}
/**
* Setter for the field referenceObjectsOther
.
*
* @param referenceObjectsOther a {@link java.util.HashMap} object.
*/
public void setReferenceObjectsOther(final HashMap referenceObjectsOther) {
this.referenceObjectsOther = referenceObjectsOther;
}
private void setupReferenceElementaryFlows(Profile.Bundle bundle) {
HashMap map = readReferenceObjects(bundle);
this.setReferenceElementaryFlows(map);
}
private void setupReferenceObjectsOther(Profile.Bundle[] bundles) {
HashMap map = new HashMap();
for (Profile.Bundle bundle : bundles) {
HashMap bundleMap = readReferenceObjects(bundle);
if (log.isDebugEnabled()) {
if ( bundleMap != null )
log.debug("adding " + bundleMap.size() + " other reference objects");
else
log.debug("no other reference objects found");
}
map.putAll(bundleMap);
}
this.setReferenceObjectsOther(map);
if (log.isDebugEnabled())
log.debug("using total of " + map.size() + " other reference objects");
}
@SuppressWarnings("unchecked")
private HashMap readReferenceObjects(Profile.Bundle bundle) {
HashMap map = new HashMap();
try {
URL url = new URL(PrefixBuilder.buildPath(bundle.getJarPath(), bundle.getUrlPrefix()) + "/" + bundle.getResource());
InputStream is = url.openStream();
GZIPInputStream gz = new GZIPInputStream(is);
ObjectInputStream ois = new ObjectInputStream(gz);
map = (HashMap) ois.readObject();
ois.close();
} catch (FileNotFoundException e) {
log.error(e);
} catch (ClassNotFoundException e) {
log.error(e);
} catch (IOException e) {
log.error(e);
} catch (Exception e) {
log.error(e);
}
log.debug("reference objects map has " + map.size() + " items");
return map;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy