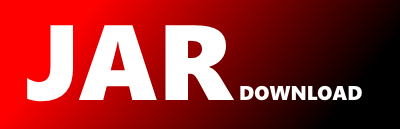
com.okworx.ilcd.validation.AbstractValidator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ilcd-validation Show documentation
Show all versions of ilcd-validation Show documentation
A Java library for performing technical validation of data in ILCD data format.
package com.okworx.ilcd.validation;
import java.io.BufferedInputStream;
import java.io.InputStream;
import java.util.HashMap;
import java.util.Map;
import com.okworx.ilcd.validation.events.Severity;
import com.okworx.ilcd.validation.events.ValidationEvent;
import org.apache.commons.io.input.BOMInputStream;
import org.apache.logging.log4j.Logger;
import com.okworx.ilcd.validation.common.DefaultValidationContext;
import com.okworx.ilcd.validation.common.IContextAwareComponent;
import com.okworx.ilcd.validation.common.IValidationContext;
import com.okworx.ilcd.validation.events.EventsList;
import com.okworx.ilcd.validation.util.IUpdateEventListener;
/**
* Abstract AbstractValidator class.
*
* @author oliver.kusche
* @version $Id: $Id
*/
public abstract class AbstractValidator implements IValidator, IContextAwareComponent {
protected final Logger log = org.apache.logging.log4j.LogManager.getLogger(this.getClass());
/**
* getAspectName.
*
* @return a {@link java.lang.String} object.
*/
public abstract String getAspectName();
/**
* getAspectDescription.
*
* @return a {@link java.lang.String} object.
*/
public abstract String getAspectDescription();
protected EventsList eventsList = new EventsList(this.getAspectName());
/**
* validate.
*
* @return a boolean.
* @throws java.lang.InterruptedException if any.
*/
public abstract boolean validate() throws InterruptedException;
protected IUpdateEventListener updateEventListener;
protected boolean reportSuccesses = false;
protected Map parameters = new HashMap();
protected IValidationContext validationContext = new DefaultValidationContext();
/**
* Getter for the field eventsList
.
*
* @return a {@link com.okworx.ilcd.validation.events.EventsList} object.
*/
public EventsList getEventsList() {
return eventsList;
}
/** {@inheritDoc} */
public void setParameter(String parameterName, Object obj) {
if (obj != null)
this.parameters.put(parameterName, obj);
else
this.parameters.remove(parameterName);
}
/**
* getParameter.
*
* @param parameterName a {@link java.lang.String} object.
* @return a {@link java.lang.Object} object.
*/
public Object getParameter(String parameterName) {
return this.parameters.get(parameterName);
}
/**
* @return the Map with all parameters
*/
public Map getParameters() {
return this.parameters;
}
/**
* reset.
*/
public void reset() {
this.eventsList = new EventsList(this.getAspectName());
this.parameters = new HashMap();
}
/**
* Constructor for AbstractValidator.
*/
public AbstractValidator() {
}
/**
* Getter for the field updateEventListener
.
*
* @return a {@link com.okworx.ilcd.validation.util.IUpdateEventListener} object.
*/
public IUpdateEventListener getUpdateEventListener() {
return updateEventListener;
}
/**
* Setter for the field updateEventListener
.
*
* @param updateEventListener a {@link com.okworx.ilcd.validation.util.IUpdateEventListener} object.
*/
public void setUpdateEventListener(
IUpdateEventListener updateEventListener) {
this.updateEventListener = updateEventListener;
}
/**
* updateProgress.
*
* @param percentFinished a double.
*/
protected void updateProgress(double percentFinished) {
if (this.getUpdateEventListener() != null)
this.getUpdateEventListener().updateProgress(percentFinished);
}
/**
* updateStatus.
*
* @param message a {@link java.lang.String} object.
*/
protected void updateStatus(String message) {
if (this.getUpdateEventListener() != null)
this.getUpdateEventListener().updateStatus(message);
}
/**
* updateStatusSetup.
*/
protected void updateStatusSetup() {
updateStatus("Setting up...");
}
/**
* updateStatusValidating.
*/
protected void updateStatusValidating() {
updateStatus("Validating...");
}
/**
* updateStatusDone.
*/
protected void updateStatusDone() {
updateStatus("Done.");
}
/**
* updateStatusCancelled.
*/
protected void updateStatusCancelled() {
updateStatus("Cancelled");
}
/**
* interrupted.
*
* @param e a {@link java.lang.InterruptedException} object.
* @throws java.lang.InterruptedException if any.
*/
protected void interrupted(InterruptedException e) throws InterruptedException {
log.info("operation was interrupted, aborting");
updateStatusCancelled();
updateProgress(0);
throw e;
}
/**
* interrupted.
*
* @throws java.lang.InterruptedException if any.
*/
protected void interrupted() throws InterruptedException {
interrupted(new InterruptedException());
}
/**
* isReportSuccesses.
*
* @return a boolean.
*/
public boolean isReportSuccesses() {
return reportSuccesses;
}
/** {@inheritDoc} */
public void setReportSuccesses(boolean reportSuccesses) {
this.reportSuccesses = reportSuccesses;
}
/**
* wrapInputStream.
*
* @param inputStream a {@link java.io.InputStream} object.
* @return a {@link java.io.InputStream} object.
*/
public InputStream wrapInputStream(InputStream inputStream) {
BOMInputStream bOMInputStream = new BOMInputStream(inputStream);
return new BufferedInputStream(bOMInputStream);
}
/**
* Getter for the field validationContext
.
*
* @return a {@link com.okworx.ilcd.validation.common.IValidationContext} object.
*/
public IValidationContext getValidationContext() {
return validationContext;
}
/** {@inheritDoc} */
public void setValidationContext(IValidationContext validationContext) {
this.validationContext = validationContext;
}
/*
If an error occurs during processing, add a FATAL validation event and log the error
*/
protected void raiseApplicationError(String message, Throwable exception) {
log.error("error configuring transformer", exception);
this.eventsList.add(new ValidationEvent(this.getAspectName(), null, Severity.FATAL, null, message + " Cause: " + exception.getMessage()));
exception.printStackTrace();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy