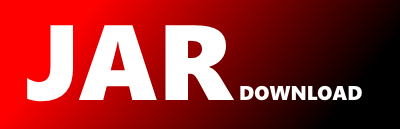
com.okworx.ilcd.validation.ValidatorChain Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ilcd-validation Show documentation
Show all versions of ilcd-validation Show documentation
A Java library for performing technical validation of data in ILCD data format.
package com.okworx.ilcd.validation;
import com.okworx.ilcd.validation.analyze.SummaryExtractor;
import com.okworx.ilcd.validation.analyze.flows.FlowsAnalyzer;
import com.okworx.ilcd.validation.events.EventsList;
import com.okworx.ilcd.validation.log.XLSLog;
import com.okworx.ilcd.validation.profile.ProfileManager;
import com.okworx.ilcd.validation.util.IUpdateEventListener;
import org.apache.commons.lang3.StringUtils;
import java.io.File;
import java.io.IOException;
import java.util.*;
/**
* Allows for chaining multiple Validators that all work on the same set of Objects
*
* @author oliver.kusche
* @version $Id: $Id
*/
public class ValidatorChain extends AbstractDatasetsValidator implements IUpdateEventListener {
protected List validators = new ArrayList<>();
private boolean batchMode = false;
private String aspectName;
private String aspectDescription;
private int finishedValidators = 0;
private HashMap extraProperties = null;
public HashMap getExtraProperties() {
if (extraProperties == null)
extraProperties = new LinkedHashMap<>();
return extraProperties;
}
public void setExtraProperties(LinkedHashMap extraProperties) {
this.extraProperties = extraProperties;
}
/**
* Constructor for ValidatorChain.
*
* @param aspectName a {@link java.lang.String} object.
*/
public ValidatorChain(String aspectName) {
super();
this.aspectName = aspectName;
this.eventsList.setAspectName(aspectName);
}
/**
* Constructor for ValidatorChain.
*/
public ValidatorChain() {
super();
this.setProfile(ProfileManager.getInstance().getDefaultProfile());
}
/**
* validate.
*
* @return a boolean.
*/
public boolean validate() {
boolean pass = this.eventsList.isPositive();
boolean validateArchiveFlag = true;
this.statistics = null;
for (IDatasetsValidator v : this.validators) {
if (validateArchiveFlag) {
v.setValidateArchives(false);
validateArchiveFlag=false;
}
v.setUpdateEventListener(this);
v.setUpdateInterval(this.getUpdateInterval());
v.setReportSuccesses(this.reportSuccesses);
v.setProfile(this.profile);
if (v instanceof AbstractDatasetsValidator)
((AbstractDatasetsValidator) v).setFileSource(this.fileSource);
}
try {
XLSLog xlsLog = null;
boolean log2Xls = batchMode && (this.fileSource != null);
// if object to validate is a ZIP or dir, create new XLS log
if (log2Xls) {
StringBuilder path = new StringBuilder();
if (this.getFileSource().getParentFile() != null) {
path.append(this.getFileSource().getParentFile().getName());
path.append(File.separator);
}
path.append(this.getFileSource().getName());
xlsLog = new XLSLog(this.validators, path.toString(), extraProperties);
}
boolean result;
EventsList events = new EventsList("General");
for (IDatasetsValidator v : this.validators) {
if (Thread.currentThread().isInterrupted()) {
log.info("operation was interrupted, aborting");
updateStatusCancelled();
break;
}
v.setObjectsToValidate(this.objectsToValidate);
if (log2Xls) {
if (v instanceof FlowsAnalyzer)
((FlowsAnalyzer) v).setWorkbook(xlsLog.getWorkbook());
}
result = v.validate();
if (!result || !v.getEventsList().isPositive())
pass = false;
events.addAll(v.getEventsList().getEvents());
if (this.statistics == null)
this.statistics = v.getStatistics();
else
this.statistics.merge(v.getStatistics());
if (log2Xls) {
// add line to summary
if (!(v instanceof FlowsAnalyzer))
xlsLog.validationSummaryAddResult(result, v);
// write messages to log
xlsLog.writeEvents(v);
}
finishedValidators++;
}
// any general events like invalid XML are stored in this.eventsList,
// we need to make sure these are also honored in batch mode
if (log.isDebugEnabled())
log.debug("general events: " + this.eventsList.getErrorCount());
this.setAspectName("General");
boolean passGeneral = !this.eventsList.hasErrors();
// document any general errors
if (log2Xls && !passGeneral) {
// add line to summary
xlsLog.validationSummaryAddResult(false, this);
// write messages to log
xlsLog.writeEvents(this);
}
// now that we have processed the general events,
// we add all events from the validators
this.statistics.merge(this.getStatistics());
this.eventsList.addAll(events.getEvents());
this.statistics.setAspects(getAllAspects());
log.info(this.eventsList.getEvents().size() + " events total");
if (log2Xls) {
// write stats to log
xlsLog.writeStats(this.statistics, this.eventsList);
// write processes summary
xlsLog.writeDatasetsSummary(this.validators);
// close log
xlsLog.writeAndClose(this.getFileSource().getAbsolutePath(), pass);
}
} catch (InterruptedException e) {
updateStatusCancelled();
updateProgress(0);
return false;
} catch (IOException e) {
return false;
}
return pass;
}
/**
* getAllAspects.
*
* @return a {@link java.lang.String} object.
*/
public String getAllAspects() {
StringBuilder sb = new StringBuilder();
boolean firstItem = true;
for (IValidator v : this.validators) {
if (!firstItem) {
sb.append(", ");
} else {
firstItem = false;
}
sb.append(v.getAspectName());
log.debug(v.getAspectName());
}
return sb.toString();
}
/**
* add.
*
* @param validator a {@link com.okworx.ilcd.validation.IDatasetsValidator} object.
*/
public void add(IDatasetsValidator validator) {
this.validators.add(validator);
}
public void initPresetValidators() {
if (this.profile != null && StringUtils.isNotBlank(this.profile.getActiveAspects())) {
StringTokenizer st = new StringTokenizer(profile.getActiveAspects(), ",");
while (st.hasMoreTokens()) {
String token = st.nextToken();
this.add(createValidator(token));
}
}
}
private IDatasetsValidator createValidator(String aspectName) {
switch (aspectName) {
case "Links":
return new LinkValidator();
case "Orphaned Items":
return new OrphansValidator();
case "XML Schema Validity":
return new SchemaValidator();
case "Profile Specific Rules":
case "Custom Validity":
return new XSLTStylesheetValidator();
case "Reference Flows":
return new ReferenceFlowValidator();
case "Categories":
return new CategoryValidator();
case "DSSummary":
return new SummaryExtractor();
}
return null;
}
/**
* Getter for the field validators
.
*
* @return a {@link java.util.List} object.
*/
public List getValidators() {
return validators;
}
/**
* Setter for the field validators
.
*
* @param validators a {@link java.util.List} object.
*/
public void setValidators(List validators) {
this.validators = validators;
}
/**
* Getter for the field aspectName
.
*
* @return a {@link java.lang.String} object.
*/
public String getAspectName() {
return aspectName;
}
/**
* Setter for the field aspectName
.
*
* @param aspectName a {@link java.lang.String} object.
*/
public void setAspectName(String aspectName) {
this.aspectName = aspectName;
this.eventsList.setAspectName(aspectName);
}
/**
* Getter for the field aspectDescription
.
*
* @return a {@link java.lang.String} object.
*/
public String getAspectDescription() {
return aspectDescription;
}
/**
* Setter for the field aspectDescription
.
*
* @param aspectDescription a {@link java.lang.String} object.
*/
public void setAspectDescription(String aspectDescription) {
this.aspectDescription = aspectDescription;
}
/** {@inheritDoc} */
public void updateProgress(double percentFinished) {
if (this.getUpdateEventListener() != null)
this.getUpdateEventListener().updateProgress((this.finishedValidators + percentFinished ) / this.validators.size());
}
/** {@inheritDoc} */
public void updateStatus(String statusMessage) {
if (this.getUpdateEventListener() != null)
this.getUpdateEventListener().updateStatus(statusMessage);
}
public boolean isBatchMode() {
return batchMode;
}
public void setBatchMode(boolean batchMode) {
this.batchMode = batchMode;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy