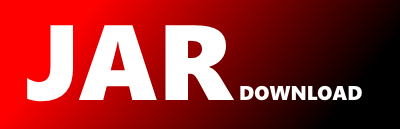
com.okworx.ilcd.validation.profile.Profile Maven / Gradle / Ivy
Show all versions of ilcd-validation Show documentation
package com.okworx.ilcd.validation.profile;
import com.okworx.ilcd.validation.util.PrefixBuilder;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.Objects;
import java.util.jar.JarFile;
/**
* Profile class.
*
* @author oliver.kusche
* @version $Id: $Id
*/
public class Profile {
private URL jarUrl = null;
private File path = null;
private Double profileMetaDataVersion = null;
private String name = null;
private String version = null;
private String description = null;
private Bundle[] schemaBundles = null;
private Bundle categoriesBundle;
private Bundle[] stylesheetBundles = null;
private String stylesheetsPath = null;
private Bundle referenceElementaryFlows = null;
private Bundle[] referenceObjectsOther = null;
private String activeAspects = null;
// Profile-SupportedAspects
private String supportedAspects = null;
private String technicalChangelog = null;
private String semanticChangelog = null;
private IncludedProfile[] includes = null;
private JarFile jarFile = null;
/**
* Constructor for Profile.
*
* @param jarUrl a {@link java.net.URL} object.
*/
public Profile(URL jarUrl) {
this.jarUrl = jarUrl;
}
/**
* getResourceAsStream.
*
* @param name a {@link java.lang.String} object.
* @return a {@link java.io.InputStream} object.
* @throws java.io.IOException if any.
*/
public InputStream getResourceAsStream(String name) throws IOException {
return jarFile.getInputStream(jarFile.getEntry(name));
}
/**
* getURLPrefix.
*
* @param resourcePath a {@link java.lang.String} object.
* @return a {@link java.lang.String} object.
*/
public String getURLPrefix(String resourcePath) {
return PrefixBuilder.buildPrefix(this.jarUrl.toString(), resourcePath);
}
/**
* getURLPrefix.
*
* @return a {@link java.lang.String} object.
*/
public String getURLPrefix() {
return PrefixBuilder.buildPrefix(this.jarUrl.toString(), "");
}
/**
* getURL.
*
* @return a {@link java.net.URL} object.
*/
public URL getURL() {
return jarUrl;
}
/**
* setURL.
*
* @param jarUrl a {@link java.net.URL} object.
*/
public void setURL(URL jarUrl) {
this.jarUrl = jarUrl;
}
/**
* Getter for the field name
.
*
* @return a {@link java.lang.String} object.
*/
public String getName() {
return name;
}
/**
* Setter for the field name
.
*
* @param name a {@link java.lang.String} object.
*/
public void setName(String name) {
this.name = name;
}
/**
* Getter for the field schemaBundles
.
*
* @return an array of {@link java.lang.String} objects.
*/
public Bundle[] getSchemaBundles() {
return schemaBundles;
}
/**
* Setter for the field schemaBundles
.
*
* @param schemaBundles an array of {@link java.lang.String} objects.
*/
public void setSchemaBundles(Bundle[] schemaBundles) {
this.schemaBundles = schemaBundles;
}
/**
* Getter for the field categoriesFile
.
*
* @return a {@link java.lang.String} object.
*/
public Bundle getCategoriesBundle() {
return categoriesBundle;
}
/**
* Setter for the field categoriesFile
.
*
* @param categoriesBundle a {@link java.lang.String} object.
*/
public void setCategoriesBundle(Bundle categoriesBundle) {
this.categoriesBundle = categoriesBundle;
}
/**
* Getter for the field stylesheetsPath
.
*
* @return a {@link java.lang.String} object.
*/
public String getStylesheetsPath() {
return stylesheetsPath;
}
/**
* Setter for the field stylesheetsPath
.
*
* @param stylesheetsPath a {@link java.lang.String} object.
*/
public void setStylesheetsPath(String stylesheetsPath) {
this.stylesheetsPath = stylesheetsPath;
}
/**
* Getter for the field stylesheetBundles
.
*
* @return a {@link java.lang.String} object.
*/
public Bundle[] getStylesheetBundles() {
return this.stylesheetBundles;
}
/**
* Setter for the field stylesheetBundles
.
*
* @param stylesheetBundles a {@link java.lang.String} object.
*/
public void setStylesheetBundles(Bundle[] stylesheetBundles) {
this.stylesheetBundles = stylesheetBundles;
}
/**
* Getter for the field jarFile
.
*
* @return a {@link java.util.jar.JarFile} object.
*/
public JarFile getJarFile() {
return jarFile;
}
/**
* Setter for the field jarFile
.
*
* @param jarFile a {@link java.util.jar.JarFile} object.
*/
public void setJarFile(JarFile jarFile) {
this.jarFile = jarFile;
}
/**
* Getter for the field version
.
*
* @return a {@link java.lang.String} object.
*/
public String getVersion() {
return version;
}
/**
* Setter for the field version
.
*
* @param version a {@link java.lang.String} object.
*/
public void setVersion(String version) {
this.version = version;
}
/**
* Getter for the field path
.
*
* @return a {@link java.io.File} object.
*/
public File getPath() {
return path;
}
/**
* Setter for the field path
.
*
* @param path a {@link java.io.File} object.
*/
public void setPath(File path) {
this.path = path;
}
/**
* Getter for the field referenceElementaryFlows
.
*
* @return a {@link java.lang.String} object.
*/
public Bundle getReferenceElementaryFlows() {
return referenceElementaryFlows;
}
/**
* Setter for the field referenceElementaryFlows
.
*
* @param referenceElementaryFlows a {@link java.lang.String} object.
*/
public void setReferenceElementaryFlows(Bundle referenceElementaryFlows) {
this.referenceElementaryFlows = referenceElementaryFlows;
}
/**
* Getter for the field referenceObjectsOther
.
*
* @return a {@link java.lang.String} object.
*/
public Bundle[] getReferenceObjectsOther() {
return referenceObjectsOther;
}
/**
* Setter for the field referenceObjectsOther
.
*
* @param referenceObjects a {@link java.lang.String} object.
*/
public void setReferenceObjectsOther(Bundle[] referenceObjects) {
this.referenceObjectsOther = referenceObjects;
}
/**
* Getter for the field description
.
*
* @return a {@link java.lang.String} object.
*/
public String getDescription() {
return description;
}
/**
* Setter for the field description
.
*
* @param description a {@link java.lang.String} object.
*/
public void setDescription(String description) {
this.description = description;
}
/**
* Getter for the field profileMetaDataVersion
.
*
* @return a {@link java.lang.Double} object.
*/
public Double getProfileMetaDataVersion() {
return profileMetaDataVersion;
}
/**
* Setter for the field profileMetaDataVersion
.
*
* @param profileMetaDataVersion a {@link java.lang.String} object.
*/
public void setProfileMetaDataVersion(Double profileMetaDataVersion) {
this.profileMetaDataVersion = profileMetaDataVersion;
}
/**
* Setter for the field profileMetaDataVersion
.
*
* @param profileMetaDataVersion a {@link java.lang.String} object.
*/
public void setProfileMetaDataVersion(String profileMetaDataVersion) {
try {
this.profileMetaDataVersion = Double.parseDouble(profileMetaDataVersion);
} catch (NumberFormatException | NullPointerException e) {
this.profileMetaDataVersion = null;
}
}
public String getActiveAspects() {
return activeAspects;
}
/**
* Setter for the field activeAspects
.
*
* @param activeAspects a {@link java.lang.String} object.
*/
public void setActiveAspects(String activeAspects) {
this.activeAspects = activeAspects;
}
public String getSupportedAspects() {
return this.supportedAspects;
}
/**
* Setter for the field supportedAspects
.
*
* @param supportedAspects a {@link java.lang.String} object.
*/
public void setSupportedAspects(String supportedAspects) {
this.supportedAspects = supportedAspects;
}
public boolean isComposed() {
return includes != null && includes.length > 0;
}
public String getTechnicalChangelog() {
return this.technicalChangelog;
}
/**
* Setter for the field technicalChangelog
.
*
* @param technicalChangelog a {@link java.lang.String} object.
*/
public void setTechnicalChangelog(String technicalChangelog) {
this.technicalChangelog = technicalChangelog;
}
public String getSemanticChangelog() {
return this.semanticChangelog;
}
/**
* Setter for the field semanticChangelog
.
*
* @param semanticChangelog a {@link java.lang.String} object.
*/
public void setSemanticChangelog(String semanticChangelog) {
this.semanticChangelog = semanticChangelog;
}
/**
* Setter for the field includes
.
*
* @param includes a {@link java.lang.String} object.
*/
public void setIncludes(IncludedProfile[] includes) {
this.includes = includes;
}
public IncludedProfile[] getIncludes() {
return this.includes;
}
@Override
public int hashCode() {
return Objects.hash(name, version);
}
@Override
public boolean equals(final Object obj) {
if (obj instanceof Profile) {
final Profile other = (Profile) obj;
return Objects.equals(name, other.name)
&& version == other.version;
} else {
return false;
}
}
public static class IncludedProfile {
private Profile profile;
private String select;
public IncludedProfile(Profile profile, String select) {
this.profile = profile;
this.select = select;
}
public Profile getProfile() {
return profile;
}
public void setProfile(Profile profile) {
this.profile = profile;
}
public String getSelect() {
return select;
}
public void setSelect(String select) {
this.select = select;
}
}
public static class Bundle {
private String urlPrefix;
private String jarPath;
private String description;
private T resource;
public Bundle(String jarPath, String urlPrefix, String description, T resource) {
this.jarPath = jarPath;
this.urlPrefix = urlPrefix;
this.description = description;
this.resource = resource;
}
public Bundle(String jarPath, String urlPrefix, T resource) {
this(jarPath, urlPrefix, null, resource);
}
public String getJarPath() {
return jarPath;
}
public void setJarPath(String jarPath) {
this.jarPath = jarPath;
}
public String getUrlPrefix() {
return urlPrefix;
}
public void setUrlPrefix(String urlPrefix) {
this.urlPrefix = urlPrefix;
}
public T getResource() {
return resource;
}
public void setResource(T resource) {
this.resource = resource;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
}
}