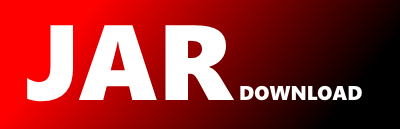
com.okworx.ilcd.validation.profile.ProfileManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ilcd-validation Show documentation
Show all versions of ilcd-validation Show documentation
A Java library for performing technical validation of data in ILCD data format.
package com.okworx.ilcd.validation.profile;
import java.io.File;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.jar.Attributes;
import java.util.jar.JarFile;
import java.util.jar.Manifest;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.FilenameUtils;
import org.apache.logging.log4j.Logger;
import com.okworx.ilcd.validation.common.Constants;
import com.okworx.ilcd.validation.exception.InvalidProfileException;
import com.okworx.ilcd.validation.util.Locator;
import com.okworx.ilcd.validation.util.StandaloneLocator;
/**
* This purpose of this class is to hold references to various profiles that
* might be used for validation. See the Profiles section in the documentation
* for more information on profiles.
*
* Example for passing parameters during initialization:
*
* new ProfileManager.ProfileManagerBuilder().cacheDir(File dir).locator(new
* EclipseLocator()).build();
*
* @author oliver.kusche
* @version $Id: $Id
*/
public class ProfileManager {
private static volatile ProfileManager SINGLETON_INSTANCE;
private final Logger log = org.apache.logging.log4j.LogManager.getLogger(this.getClass());
private Map profileStore = new HashMap();
private Locator locator = new StandaloneLocator();
private File cacheDir = new File(org.apache.commons.io.FileUtils.getTempDirectoryPath() + File.separator
+ System.currentTimeMillis());
private boolean registerDefaultProfile = true;
private boolean registerDefaultSecondaryProfiles = false;
private URL defaultProfileURL;
final String MAX_XPATH_GROUP_LIMIT = "jdk.xml.xpathExprGrpLimit";
final String MAX_XPATH_OPERATOR_LIMIT = "jdk.xml.xpathExprOpLimit";
final String MAX_XPATH_TOTAL = "jdk.xml.xpathTotalOpLimit";
private ProfileManager() {
init();
}
private ProfileManager(ProfileManagerBuilder builder) {
this.locator = builder.locator;
this.cacheDir = builder.cacheDir;
this.registerDefaultProfile = builder.registerDefaultProfile;
this.registerDefaultSecondaryProfiles = builder.registerDefaultSecondaryProfiles;
init();
}
/**
* getInstance.
*
* @return a {@link com.okworx.ilcd.validation.profile.ProfileManager} object.
*/
public static ProfileManager getInstance() {
if(SINGLETON_INSTANCE == null) {
synchronized(ProfileManager.class) {
if(SINGLETON_INSTANCE == null) {
SINGLETON_INSTANCE = new ProfileManager();
}
}
}
return SINGLETON_INSTANCE;
}
private void init() {
try {
// System.setProperty(MAX_XPATH_GROUP_LIMIT, "10");
System.setProperty(MAX_XPATH_OPERATOR_LIMIT, "200");
// System.setProperty(MAX_XPATH_TOTAL, "10000");
if (!this.cacheDir.exists()) {
if (log.isTraceEnabled()) {
log.trace("cache dir " + this.cacheDir.getAbsolutePath() + " does not exist. Creating...");
}
FileUtils.forceMkdir(this.cacheDir);
FileUtils.forceDeleteOnExit(this.cacheDir);
}
// register default profile
if (this.registerDefaultProfile) {
registerDefaultProfile();
}
// if loading of secondary default profiles is configured (which is false by default), register them
if (this.registerDefaultSecondaryProfiles) {
registerDefaultSecondaryProfiles();
}
} catch (Exception e) {
log.error(e);
e.printStackTrace();
}
}
public void registerDefaultSecondaryProfiles()
throws MalformedURLException, URISyntaxException, InvalidProfileException {
if (log.isDebugEnabled())
log.debug("Registering secondary default profiles");
for (String profilePath : Constants.DEFAULT_SECONDARY_PROFILE_JARS) {
URL url = this.getClass().getClassLoader().getResource(profilePath).toURI().toURL();
registerProfile(url);
}
}
public void registerDefaultProfile() throws IOException, InvalidProfileException {
this.defaultProfileURL = this.getClass().getClassLoader().getResource(Constants.DEFAULT_PROFILE_JAR);
if (log.isTraceEnabled()) {
log.trace("trying to register default profile " + Constants.DEFAULT_PROFILE_JAR);
log.trace("profile URL is " + this.defaultProfileURL);
log.trace("resolving to " + locator.resolve(this.defaultProfileURL).toString());
log.trace("using locator " + this.locator.getClass().getCanonicalName());
log.trace("using cache dir " + this.cacheDir.getPath());
}
registerProfile(this.defaultProfileURL);
}
public void reset() {
reset(false);
}
public void reset(boolean hardReset) {
this.profileStore = new HashMap();
if (hardReset || this.registerDefaultProfile) {
try {
this.registerDefaultProfile();
} catch (IOException | InvalidProfileException e) {
log.error("could not register default profile", e);
}
}
// if loading of secondary default profiles is configured (which is false by default), register them
if (this.registerDefaultSecondaryProfiles) {
try {
this.registerDefaultSecondaryProfiles();
} catch (IOException | InvalidProfileException | URISyntaxException e) {
log.error("could not register default secondary profiles", e);
}
}
}
/**
* registerProfile.
*
* @param url a {@link java.net.URL} object.
* @return a {@link com.okworx.ilcd.validation.profile.Profile} object.
* @throws com.okworx.ilcd.validation.exception.InvalidProfileException if any.
*/
public Profile registerProfile(URL url) throws InvalidProfileException {
if (url == null) throw new InvalidProfileException();
log.debug("registering profile at " + url.toString());
try {
Profile profile = getProfile(url);
this.profileStore.put(url, profile);
return profile;
} catch (IOException e) {
e.printStackTrace();
throw new InvalidProfileException();
} finally {
}
}
/**
* Parse a profile under the given URL and return a {@link com.okworx.ilcd.validation.profile.Profile} object
*
* @param url
* @return the {@link com.okworx.ilcd.validation.profile.Profile}
* @throws IOException
* @throws InvalidProfileException
*/
public Profile getProfile(URL url) throws IOException, InvalidProfileException {
log.trace("resolving to " + locator.resolve(url));
File physicalJar = extractJar(locator.resolve(url));
JarFile jar = new JarFile(physicalJar);
Manifest m = jar.getManifest();
Profile profile = new Profile(url);
profile.setJarFile(jar);
profile.setPath(physicalJar);
Attributes atts = m.getAttributes("ILCD-Validator-Profile");
if (atts == null) {
jar.close();
throw new InvalidProfileException();
}
profile = ProfileManifestParser.parseManifest(profile, atts);
return profile;
}
/**
* deregisterProfile.
*
* @param profile a {@link com.okworx.ilcd.validation.profile.Profile} object.
*/
public void deregisterProfile(Profile profile) {
this.profileStore.remove(profile.getURL());
}
private File extractJar(URL path) {
try {
if (log.isDebugEnabled())
log.debug("extracting profile with URL " + path + " to cache dir");
String plainName = FilenameUtils.getName(path.getFile());
File extractedJar = new File(this.cacheDir.getAbsolutePath() + File.separator + plainName);
if (!path.equals(extractedJar.toURI().toURL())) {
FileUtils.copyInputStreamToFile(path.openStream(), extractedJar);
}
if (log.isDebugEnabled())
log.debug("extracted at " + extractedJar.getAbsolutePath());
return extractedJar;
} catch (Exception ex) {
ex.printStackTrace();
log.error(ex);
}
return null;
}
protected boolean supportsMetaDataVersion(Profile profile, Double version) {
if (version == null)
return false;
return (profile.getProfileMetaDataVersion() >= version);
}
/**
* getProfiles.
*
* @return a {@link java.util.Collection} object.
*/
public Collection getProfiles() {
return this.profileStore.values();
}
/**
* getDefaultProfile.
*
* @return a {@link com.okworx.ilcd.validation.profile.Profile} object.
*/
public Profile getDefaultProfile() {
return this.profileStore.get(this.defaultProfileURL);
}
/**
* Getter for the field locator
.
*
* @return a {@link com.okworx.ilcd.validation.util.Locator} object.
*/
public Locator getLocator() {
return locator;
}
/**
* Setter for the field locator
.
*
* @param locator a {@link com.okworx.ilcd.validation.util.Locator} object.
*/
public void setLocator(Locator locator) {
this.locator = locator;
}
/**
* Getter for the field cacheDir
.
*
* @return a {@link java.io.File} object.
*/
public File getCacheDir() {
return cacheDir;
}
/**
* Setter for the field cacheDir
.
*
* @param cacheDir a {@link java.io.File} object.
*/
public void setCacheDir(File cacheDir) {
this.cacheDir = cacheDir;
}
private static void build(ProfileManagerBuilder builder) {
SINGLETON_INSTANCE = new ProfileManager(builder);
}
/**
* @return the registerDefaultProfile
*/
public boolean isRegisterDefaultProfile() {
return registerDefaultProfile;
}
/**
* @param registerDefaultProfile the registerDefaultProfile to set
*/
public void setRegisterDefaultProfile(boolean registerDefaultProfile) {
this.registerDefaultProfile = registerDefaultProfile;
}
/**
* @return the registerDefaultSecondaryProfiles
*/
public boolean isRegisterDefaultSecondaryProfiles() {
return registerDefaultSecondaryProfiles;
}
/**
* @param registerDefaultSecondaryProfiles the registerDefaultSecondaryProfiles to set
*/
public void setRegisterDefaultSecondaryProfiles(boolean registerDefaultSecondaryProfiles) {
this.registerDefaultSecondaryProfiles = registerDefaultSecondaryProfiles;
}
/**
* Use this to build an instance of ProfileManager with a custom
* configuration
*
*/
public static class ProfileManagerBuilder {
private Locator locator = new StandaloneLocator();
private File cacheDir = new File(org.apache.commons.io.FileUtils.getTempDirectoryPath() + File.separator
+ System.currentTimeMillis());
private boolean registerDefaultProfile = true;
private boolean registerDefaultSecondaryProfiles = false;
public ProfileManagerBuilder() {
}
public ProfileManagerBuilder locator(Locator locator) {
this.locator = locator;
return this;
}
public ProfileManagerBuilder cacheDir(File cacheDir) {
this.cacheDir = cacheDir;
return this;
}
public ProfileManagerBuilder registerDefaultProfiles(boolean regDefault, boolean regDefaultSec) {
this.registerDefaultProfile = regDefault;
this.registerDefaultSecondaryProfiles = regDefaultSec;
return this;
}
public void build() {
ProfileManager.build(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy