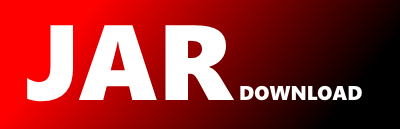
com.olapdb.core.Olap Maven / Gradle / Ivy
The newest version!
package com.olapdb.core;
import com.alibaba.fastjson.JSONObject;
import com.olapdb.core.config.*;
import com.olapdb.core.hll.HLLDistinct;
import com.olapdb.core.tables.*;
import com.olapdb.core.utils.DwUtil;
import com.olapdb.core.utils.TimeUtil;
import com.olapdb.core.workingarea.WorkingAreaManager;
import com.olapdb.obase.data.Entity;
import com.olapdb.obase.utils.Obase;
import lombok.extern.slf4j.Slf4j;
import org.apache.hadoop.hbase.HConstants;
import org.apache.hadoop.hbase.HRegionLocation;
import org.apache.hadoop.hbase.client.Durability;
import org.apache.hadoop.hbase.client.RegionInfo;
import org.apache.hadoop.hbase.client.Scan;
import java.net.InetAddress;
import java.util.*;
import java.util.concurrent.atomic.AtomicLong;
import java.util.concurrent.atomic.AtomicReference;
import java.util.stream.Collectors;
@Slf4j
public class Olap {
private final static long serialVersionUID = 0x01B21DF2138E4000L;
/**
* submit precompiled segment to olap database
* @param cubeName, cube name
* @param facts, fact list
* @throws Exception
*/
public static void submitSegment(String cubeName, List facts) throws Exception {
Cube cube = new Cube(cubeName);
if (cube.needConnect()) {
log.info("Cube with name {} not exist.", cubeName);
throw new Exception("Cube not exist.");
}
if (!cube.getEnable()) {
log.info("Cube with name {} is disable.", cubeName);
throw new Exception("Cube is disable");
}
///////////////////////////////////////////////////////////////////////////////////////////
//If a time dimension is specified, replace the time dimension with a hierarchical time dimension group
///////////////////////////////////////////////////////////////////////////////////////////
String timeDim = cube.getTimeDimension();
String timeDimType = timeDim.isEmpty()?null:cube.getDimensionType(timeDim);
AtomicLong youngestDataTime = new AtomicLong(0);
AtomicLong eldestDataTime = new AtomicLong(0);
///////////////////////////////////////////////////////////////////////////////////////////
if (facts == null || facts.isEmpty()) {
log.info("Table {} facts is empty", cubeName);
throw new Exception("facts is empty");
}
log.info("CubeName= [{}] start prebuild", cube.getIdenticalName());
Segment segment = null;
SegBuildTask job = null;
long segId = WorkingAreaManager.allocateSegmentId();
try{
DwUtil.execFlushSegment(segId);
segment = Segment.newInstance(cube, SegmentLevel.LEVEL_0, segId);
segment.setType(SegmentType.BUILD);
segment.setPhase(SegmentPhase.CREATED);
segment.setFactCount(facts.size());
segment.connect();
job = SegBuildTask.newInstance(segment);
job.setPhase(TaskPhase.CREATED);
job.setStartTime(System.currentTimeMillis());
job.connect();
List sortedDimList = cube.getDimensionList();
String cuboidName = cube.getIdenticalName() + ":" + DwUtil.unify(sortedDimList, cube.getIndexDimensionList());
Cuboid cuboid = Cuboid.stream(cube.getIdenticalName())
.filter(e -> e.getPhase() == CuboidPhase.CHARGING || e.getPhase() == CuboidPhase.PRODUCTIVE)
.filter(e -> e.getName().equals(cuboidName))
.findFirst()
.get();
if (cuboid == null) {
log.info("Cube [{}] prebuild failed.", cube.getIdenticalName());
throw new Exception("Cuboid " + cuboidName + " not found.");
}
try {
job.setHostName(InetAddress.getLocalHost().getHostName());
} catch (Exception e) {
}
Map voxelMap = new HashMap<>();
long startTime = System.currentTimeMillis();
final AtomicLong factCounter = new AtomicLong(0);
final long segid = segment.getId();
String cubodingNamePrefix = segid + ":" + cuboid.getId() + ":";
AtomicReference exceptionReference = new AtomicReference<>();
Optional find = facts.stream().parallel().filter(e -> {
try {
JSONObject jo = JSONObject.parseObject(e);
if(!timeDim.isEmpty()){
TimeUtil.replaceTimeDimensionToTimeHierachy(jo, timeDim,timeDimType, youngestDataTime, eldestDataTime);
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy