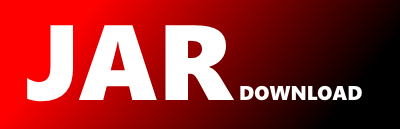
com.olapdb.obase.data.RecommendEntity Maven / Gradle / Ivy
The newest version!
package com.olapdb.obase.data;
import com.olapdb.obase.utils.Annealing;
import com.olapdb.obase.utils.VectorUtil;
import org.apache.hadoop.hbase.client.Result;
import java.util.*;
import java.util.stream.Collectors;
public abstract class RecommendEntity extends SearchableEntity{
public RecommendEntity(byte[] row){
super(row);
}
public RecommendEntity(Result r) {
super(r);
}
public double getAneCoef(){return Annealing.FIRM_COEF;}
public int getTagDimension(){return 100;}
public void learningTag(String tag, Double rate){
byte[] data = this.getAttribute("tag", tag);
double old = 0;
if(data != null){
old = Bytez.toDouble(data);
}
this.setAttribute("tag", tag, Bytez.from(Annealing.add(old, rate, this.getAneCoef())));
checkTagDimension();
}
public void learningTags_Old(List tags, Double rate){
if(tags == null || tags.isEmpty() || rate ==0)
return;
int len = tags.size();
String[] items = new String[len];
byte[][] values = new byte[len][];
for(int i=0; i tags, Double rate){
if(tags == null || tags.isEmpty() || rate ==0)
return;
List oldTags = this.getTags();
Map olds = new HashMap();
Map curs = new HashMap();
for(Tag t : oldTags){
olds.put(t.word, t.weight);
}
curs.putAll(olds);
for(Tag t:tags){
double old = curs.getOrDefault(t.word, new Double(0));
double learning = t.weight*rate;
curs.put(t.word, Annealing.add(old, learning, this.getAneCoef()));
}
List curTags = new Vector();
for(String word : curs.keySet()){
curTags.add(new Tag(word, curs.get(word)));
}
formatTags(curTags);
curs.clear();
for(Tag t:curTags){
curs.put(t.word, t.weight);
}
//update
List updates = new Vector();
for(Tag t : tags){
if(curs.containsKey(t.word)){
updates.add(t.word);
}
}
Collections.sort(updates);
if(!updates.isEmpty()){
int len = updates.size();
String[] items = new String[len];
byte[][] values = new byte[len][];
for(int i=0; i removes = new Vector();
for(Tag t : oldTags){
if(!curs.containsKey(t.word)){
removes.add(t.word);
}
}
if(!removes.isEmpty()){
this.deleteAttributes("tag", removes);
}
}
public void setTags(List tags){
if(tags == null || tags.isEmpty())
return;
formatTags(tags);
int len = tags.size();
String[] items = new String[len];
byte[][] values = new byte[len][];
for(int i=0; i getTags() {
return getAttributeItems("tag").stream()
.map(item->new Tag(item, Bytez.toDouble(this.getAttribute("tag", item))))
.sorted().collect(Collectors.toList());
}
public List getNormalTags() {
List tags = getTags();
double total = tags.stream().map(t->t.weight*t.weight).reduce(0.0, (x,y)->x+y);
if(total == 0)total = 1;
final double length = Math.sqrt(total);;
tags.forEach(t->t.weight/=length);
return tags;
}
public List getShowTags(int size){
List tags = this.getTags();
Collections.sort(tags);
tags = tags.subList(0, Math.min(size, tags.size()));
for(Tag t : tags){
t.weight = Annealing.anneValue(t.weight, this.getAneCoef());
}
return tags;
}
public List getAnneTags(){
List tags = this.getTags();
for(Tag t : tags){
t.weight = Annealing.anneValue(t.weight, this.getAneCoef());
}
return tags;
}
public double similar(RecommendEntity other){
return VectorUtil.calcSimilar(getTags(), other.getTags());
}
public static double calcSimilar(RecommendEntity a, RecommendEntity b){
return VectorUtil.calcSimilar(a.getTags(), b.getTags());
}
private void formatTags(List tags){
Collections.sort(tags);
for(int i=tags.size()-1; i > this.getTagDimension()-1; i--){
tags.remove(i);
}
}
private void checkTagDimension(){
List tags = this.getTags();
if(tags.size() <= this.getTagDimension())
return;
Collections.sort(tags);
List removes = new Vector();
for(int i=this.getTagDimension(); i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy