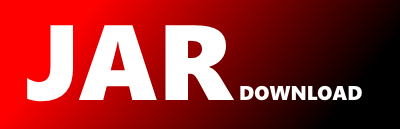
com.omertron.themoviedbapi.wrapper.WrapperImages Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of themoviedbapi Show documentation
Show all versions of themoviedbapi Show documentation
API for the TheMovieDb.org website
/*
* Copyright (c) 2004-2013 Stuart Boston
*
* This file is part of TheMovieDB API.
*
* TheMovieDB API is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* any later version.
*
* TheMovieDB API is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with TheMovieDB API. If not, see .
*
*/
package com.omertron.themoviedbapi.wrapper;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.omertron.themoviedbapi.model.Artwork;
import com.omertron.themoviedbapi.model.ArtworkType;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
/**
*
* @author Stuart
*/
public class WrapperImages extends AbstractWrapperAll implements Serializable {
private static final long serialVersionUID = 1L;
@JsonProperty("backdrops")
private List backdrops = Collections.EMPTY_LIST;
@JsonProperty("posters")
private List posters = Collections.EMPTY_LIST;
@JsonProperty("profiles")
private List profiles = Collections.EMPTY_LIST;
public List getBackdrops() {
return backdrops;
}
public List getPosters() {
return posters;
}
public List getProfiles() {
return profiles;
}
public void setBackdrops(List backdrops) {
this.backdrops = backdrops;
}
public void setPosters(List posters) {
this.posters = posters;
}
public void setProfiles(List profiles) {
this.profiles = profiles;
}
/**
* Return a list of all the artwork with their types.
*
* Leaving the parameters blank will return all types
*
* @param artworkList
* @return
*/
public List getAll(ArtworkType... artworkList) {
List artwork = new ArrayList();
List types;
if (artworkList.length > 0) {
types = new ArrayList(Arrays.asList(artworkList));
} else {
types = new ArrayList(Arrays.asList(ArtworkType.values()));
}
// Add all the posters to the list
if (types.contains(ArtworkType.POSTER)) {
updateArtworkType(posters, ArtworkType.POSTER);
artwork.addAll(posters);
}
// Add all the backdrops to the list
if (types.contains(ArtworkType.BACKDROP)) {
updateArtworkType(backdrops, ArtworkType.BACKDROP);
artwork.addAll(backdrops);
}
// Add all the backdrops to the list
if (types.contains(ArtworkType.PROFILE)) {
updateArtworkType(profiles, ArtworkType.PROFILE);
artwork.addAll(profiles);
}
return artwork;
}
/**
* Update the artwork type for the artwork list
*
* @param artworkList
* @param type
*/
private void updateArtworkType(List artworkList, ArtworkType type) {
for (Artwork artwork : artworkList) {
artwork.setArtworkType(type);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy