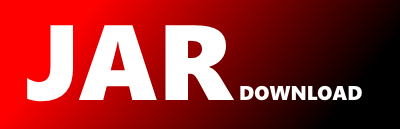
com.omgm.speedy.eps.soap.model.EPSProvider Maven / Gradle / Ivy
package com.omgm.speedy.eps.soap.model;
import java.util.List;
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebResult;
import javax.jws.WebService;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.datatype.XMLGregorianCalendar;
import javax.xml.ws.Action;
import javax.xml.ws.FaultAction;
import javax.xml.ws.RequestWrapper;
import javax.xml.ws.ResponseWrapper;
/**
* This class was generated by the JAX-WS RI.
* JAX-WS RI 2.2.4-b01
* Generated source version: 2.2
*
*/
@WebService(name = "EPSProvider", targetNamespace = "http://ver01.eps.speedy.sirma.com/")
@XmlSeeAlso({
ObjectFactory.class
})
public interface EPSProvider {
/**
*
* @param username
* @param password
* @return
* returns com.omgm.speedy.eps.soap.model.ResultLogin
* @throws InvalidUsernameOrPasswordException_Exception
* @throws NoUserPermissionsException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "login", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.Login")
@ResponseWrapper(localName = "loginResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.LoginResponse")
public ResultLogin login(
@WebParam(name = "username", targetNamespace = "")
String username,
@WebParam(name = "password", targetNamespace = "")
String password)
throws InvalidUsernameOrPasswordException_Exception, NoUserPermissionsException_Exception
;
/**
*
* @param sessionId
* @param refreshSession
* @return
* returns boolean
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "isSessionActive", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.IsSessionActive")
@ResponseWrapper(localName = "isSessionActiveResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.IsSessionActiveResponse")
public boolean isSessionActive(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "refreshSession", targetNamespace = "")
boolean refreshSession);
/**
*
* @param sessionId
* @param date
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listServices", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListServices")
@ResponseWrapper(localName = "listServicesResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListServicesResponse")
public List listServices(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "date", targetNamespace = "")
XMLGregorianCalendar date)
throws InvalidSessionException_Exception
;
/**
*
* @param senderSiteId
* @param sessionId
* @param receiverSiteId
* @param date
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listServicesForSites", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListServicesForSites")
@ResponseWrapper(localName = "listServicesForSitesResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListServicesForSitesResponse")
public List listServicesForSites(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "date", targetNamespace = "")
XMLGregorianCalendar date,
@WebParam(name = "senderSiteId", targetNamespace = "")
long senderSiteId,
@WebParam(name = "receiverSiteId", targetNamespace = "")
long receiverSiteId)
throws InvalidSessionException_Exception
;
/**
*
* @param senderSiteId
* @param documents
* @param sessionId
* @param receiverSiteId
* @param serviceTypeId
* @param date
* @return
* returns com.omgm.speedy.eps.soap.model.ResultMinMaxReal
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getWeightInterval", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetWeightInterval")
@ResponseWrapper(localName = "getWeightIntervalResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetWeightIntervalResponse")
public ResultMinMaxReal getWeightInterval(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "serviceTypeId", targetNamespace = "")
long serviceTypeId,
@WebParam(name = "senderSiteId", targetNamespace = "")
long senderSiteId,
@WebParam(name = "receiverSiteId", targetNamespace = "")
long receiverSiteId,
@WebParam(name = "date", targetNamespace = "")
XMLGregorianCalendar date,
@WebParam(name = "documents", targetNamespace = "")
boolean documents)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @param nomenType
* @return
* returns java.lang.String
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getAddressNomenclature", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetAddressNomenclature")
@ResponseWrapper(localName = "getAddressNomenclatureResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetAddressNomenclatureResponse")
public String getAddressNomenclature(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "nomenType", targetNamespace = "")
int nomenType)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listAllSites", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListAllSites")
@ResponseWrapper(localName = "listAllSitesResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListAllSitesResponse")
public List listAllSites(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "language", targetNamespace = "")
ParamLanguage language)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @param name
* @param type
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listSites", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListSites")
@ResponseWrapper(localName = "listSitesResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListSitesResponse")
public List listSites(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "type", targetNamespace = "")
String type,
@WebParam(name = "name", targetNamespace = "")
String name,
@WebParam(name = "language", targetNamespace = "")
ParamLanguage language)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @param filter
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listSitesEx", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListSitesEx")
@ResponseWrapper(localName = "listSitesExResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListSitesExResponse")
public List listSitesEx(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "filter", targetNamespace = "")
ParamFilterSite filter,
@WebParam(name = "language", targetNamespace = "")
ParamLanguage language)
throws InvalidSessionException_Exception
;
/**
*
* @param siteId
* @param sessionId
* @return
* returns com.omgm.speedy.eps.soap.model.ResultSite
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getSiteById", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetSiteById")
@ResponseWrapper(localName = "getSiteByIdResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetSiteByIdResponse")
public ResultSite getSiteById(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "siteId", targetNamespace = "")
Long siteId)
throws InvalidSessionException_Exception
;
/**
*
* @param addrNomen
* @param sessionId
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getSitesByAddrNomenType", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetSitesByAddrNomenType")
@ResponseWrapper(localName = "getSitesByAddrNomenTypeResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetSitesByAddrNomenTypeResponse")
public List getSitesByAddrNomenType(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "addrNomen", targetNamespace = "")
AddrNomen addrNomen)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listStreetTypes", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListStreetTypes")
@ResponseWrapper(localName = "listStreetTypesResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListStreetTypesResponse")
public List listStreetTypes(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "language", targetNamespace = "")
ParamLanguage language)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listQuarterTypes", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListQuarterTypes")
@ResponseWrapper(localName = "listQuarterTypesResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListQuarterTypesResponse")
public List listQuarterTypes(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "language", targetNamespace = "")
ParamLanguage language)
throws InvalidSessionException_Exception
;
/**
*
* @param siteId
* @param sessionId
* @param name
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listStreets", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListStreets")
@ResponseWrapper(localName = "listStreetsResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListStreetsResponse")
public List listStreets(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "name", targetNamespace = "")
String name,
@WebParam(name = "siteId", targetNamespace = "")
long siteId,
@WebParam(name = "language", targetNamespace = "")
ParamLanguage language)
throws InvalidSessionException_Exception
;
/**
*
* @param siteId
* @param sessionId
* @param name
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listQuarters", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListQuarters")
@ResponseWrapper(localName = "listQuartersResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListQuartersResponse")
public List listQuarters(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "name", targetNamespace = "")
String name,
@WebParam(name = "siteId", targetNamespace = "")
long siteId,
@WebParam(name = "language", targetNamespace = "")
ParamLanguage language)
throws InvalidSessionException_Exception
;
/**
*
* @param siteId
* @param sessionId
* @param name
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listCommonObjects", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListCommonObjects")
@ResponseWrapper(localName = "listCommonObjectsResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListCommonObjectsResponse")
public List listCommonObjects(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "name", targetNamespace = "")
String name,
@WebParam(name = "siteId", targetNamespace = "")
long siteId)
throws InvalidSessionException_Exception
;
/**
*
* @param siteId
* @param sessionId
* @param name
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listBlocks", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListBlocks")
@ResponseWrapper(localName = "listBlocksResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListBlocksResponse")
public List listBlocks(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "name", targetNamespace = "")
String name,
@WebParam(name = "siteId", targetNamespace = "")
long siteId)
throws InvalidSessionException_Exception
;
/**
*
* @param siteId
* @param sessionId
* @param name
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listOffices", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListOffices")
@ResponseWrapper(localName = "listOfficesResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListOfficesResponse")
public List listOffices(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "name", targetNamespace = "")
String name,
@WebParam(name = "siteId", targetNamespace = "")
Long siteId)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @param clientId
* @return
* returns com.omgm.speedy.eps.soap.model.ResultClientData
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getClientById", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetClientById")
@ResponseWrapper(localName = "getClientByIdResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetClientByIdResponse")
public ResultClientData getClientById(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "clientId", targetNamespace = "")
long clientId)
throws InvalidSessionException_Exception
;
/**
*
* @param clientQuery
* @param sessionId
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "searchClients", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.SearchClients")
@ResponseWrapper(localName = "searchClientsResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.SearchClientsResponse")
public List searchClients(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "clientQuery", targetNamespace = "")
ParamClientSearch clientQuery)
throws InvalidSessionException_Exception
;
/**
*
* @param picking
* @param sessionId
* @return
* returns com.omgm.speedy.eps.soap.model.ResultBOL
* @throws InvalidSessionException_Exception
* @throws PickingValidationException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "createBillOfLading", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CreateBillOfLading")
@ResponseWrapper(localName = "createBillOfLadingResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CreateBillOfLadingResponse")
public ResultBOL createBillOfLading(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "picking", targetNamespace = "")
ParamPicking picking)
throws InvalidSessionException_Exception, PickingValidationException_Exception
;
/**
*
* @param includeAutoPrintJS
* @param sessionId
* @param billOfLading
* @return
* returns byte[]
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "createBillOfLadingPDF", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CreateBillOfLadingPDF")
@ResponseWrapper(localName = "createBillOfLadingPDFResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CreateBillOfLadingPDFResponse")
public byte[] createBillOfLadingPDF(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "billOfLading", targetNamespace = "")
long billOfLading,
@WebParam(name = "includeAutoPrintJS", targetNamespace = "")
boolean includeAutoPrintJS)
throws InvalidSessionException_Exception
;
/**
*
* @param picking
* @param sessionId
* @return
* returns com.omgm.speedy.eps.soap.model.ResultBOL
* @throws InvalidSessionException_Exception
* @throws PickingValidationException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "updateBillOfLading", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.UpdateBillOfLading")
@ResponseWrapper(localName = "updateBillOfLadingResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.UpdateBillOfLadingResponse")
public ResultBOL updateBillOfLading(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "picking", targetNamespace = "")
ParamPicking picking)
throws InvalidSessionException_Exception, PickingValidationException_Exception
;
/**
*
* @param order
* @param sessionId
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "createOrder", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CreateOrder")
@ResponseWrapper(localName = "createOrderResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CreateOrderResponse")
public List createOrder(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "order", targetNamespace = "")
ParamOrder order)
throws InvalidSessionException_Exception
;
/**
*
* @param parcelId
* @param sessionId
* @return
* returns byte[]
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "createCustomTravelLabelPDFType1", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CreateCustomTravelLabelPDFType1")
@ResponseWrapper(localName = "createCustomTravelLabelPDFType1Response", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CreateCustomTravelLabelPDFType1Response")
public byte[] createCustomTravelLabelPDFType1(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "parcelId", targetNamespace = "")
long parcelId)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @param params
* @return
* returns byte[]
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "createPDF", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CreatePDF")
@ResponseWrapper(localName = "createPDFResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CreatePDFResponse")
public byte[] createPDF(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "params", targetNamespace = "")
ParamPDF params)
throws InvalidSessionException_Exception
;
/**
*
* @param calculation
* @param sessionId
* @return
* returns com.omgm.speedy.eps.soap.model.ResultCalculation
* @throws InvalidSessionException_Exception
* @throws PickingValidationException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "calculate", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.Calculate")
@ResponseWrapper(localName = "calculateResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CalculateResponse")
public ResultCalculation calculate(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "calculation", targetNamespace = "")
ParamCalculation calculation)
throws InvalidSessionException_Exception, PickingValidationException_Exception
;
/**
*
* @param calculation
* @param serviceTypeIds
* @param sessionId
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
* @throws PickingValidationException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "calculateMultipleServices", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CalculateMultipleServices")
@ResponseWrapper(localName = "calculateMultipleServicesResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CalculateMultipleServicesResponse")
public List calculateMultipleServices(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "calculation", targetNamespace = "")
ParamCalculation calculation,
@WebParam(name = "serviceTypeIds", targetNamespace = "")
List serviceTypeIds)
throws InvalidSessionException_Exception, PickingValidationException_Exception
;
/**
*
* @param picking
* @param sessionId
* @return
* returns com.omgm.speedy.eps.soap.model.ResultCalculation
* @throws InvalidSessionException_Exception
* @throws PickingValidationException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "calculatePicking", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CalculatePicking")
@ResponseWrapper(localName = "calculatePickingResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.CalculatePickingResponse")
public ResultCalculation calculatePicking(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "picking", targetNamespace = "")
ParamPicking picking)
throws InvalidSessionException_Exception, PickingValidationException_Exception
;
/**
*
* @param sessionId
* @param billOfLading
* @throws InvalidSessionException_Exception
*/
@WebMethod
@RequestWrapper(localName = "invalidatePicking", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.InvalidatePicking")
@ResponseWrapper(localName = "invalidatePickingResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.InvalidatePickingResponse")
public void invalidatePicking(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "billOfLading", targetNamespace = "")
long billOfLading)
throws InvalidSessionException_Exception
;
/**
*
* @param parcel
* @param sessionId
* @return
* returns long
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "addParcel", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.AddParcel")
@ResponseWrapper(localName = "addParcelResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.AddParcelResponse")
public long addParcel(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "parcel", targetNamespace = "")
ParamParcel parcel)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @param billOfLading
* @return
* returns com.omgm.speedy.eps.soap.model.ResultBOL
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "finalizeBillOfLadingCreation", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.FinalizeBillOfLadingCreation")
@ResponseWrapper(localName = "finalizeBillOfLadingCreationResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.FinalizeBillOfLadingCreationResponse")
public ResultBOL finalizeBillOfLadingCreation(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "billOfLading", targetNamespace = "")
long billOfLading)
throws InvalidSessionException_Exception
;
/**
*
* @param senderOfficeId
* @param senderSiteId
* @param sessionId
* @param serviceTypeId
* @param minDate
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getAllowedDaysForTaking", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetAllowedDaysForTaking")
@ResponseWrapper(localName = "getAllowedDaysForTakingResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetAllowedDaysForTakingResponse")
public List getAllowedDaysForTaking(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "serviceTypeId", targetNamespace = "")
long serviceTypeId,
@WebParam(name = "senderSiteId", targetNamespace = "")
Long senderSiteId,
@WebParam(name = "senderOfficeId", targetNamespace = "")
Long senderOfficeId,
@WebParam(name = "minDate", targetNamespace = "")
XMLGregorianCalendar minDate)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @param billOfLading
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getPickingParcels", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetPickingParcels")
@ResponseWrapper(localName = "getPickingParcelsResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetPickingParcelsResponse")
public List getPickingParcels(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "billOfLading", targetNamespace = "")
long billOfLading)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @param billOfLading
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "trackPicking", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.TrackPicking")
@ResponseWrapper(localName = "trackPickingResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.TrackPickingResponse")
public List trackPicking(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "billOfLading", targetNamespace = "")
long billOfLading)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @param language
* @param billOfLading
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "trackPickingEx", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.TrackPickingEx")
@ResponseWrapper(localName = "trackPickingExResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.TrackPickingExResponse")
public List trackPickingEx(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "billOfLading", targetNamespace = "")
long billOfLading,
@WebParam(name = "language", targetNamespace = "")
ParamLanguage language)
throws InvalidSessionException_Exception
;
/**
*
* @param parcelId
* @param sessionId
* @param language
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "trackParcel", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.TrackParcel")
@ResponseWrapper(localName = "trackParcelResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.TrackParcelResponse")
public List trackParcel(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "parcelId", targetNamespace = "")
long parcelId,
@WebParam(name = "language", targetNamespace = "")
ParamLanguage language)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @param params
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "searchPickingsByRefNumber", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.SearchPickingsByRefNumber")
@ResponseWrapper(localName = "searchPickingsByRefNumberResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.SearchPickingsByRefNumberResponse")
public List searchPickingsByRefNumber(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "params", targetNamespace = "")
ParamSearchByRefNum params)
throws InvalidSessionException_Exception
;
/**
*
* @param address
* @param sessionId
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "addressSearch", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.AddressSearch")
@ResponseWrapper(localName = "addressSearchResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.AddressSearchResponse")
public List addressSearch(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "address", targetNamespace = "")
ParamAddressSearch address)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @param coordY
* @param coordX
* @return
* returns long
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getMicroregionId", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetMicroregionId")
@ResponseWrapper(localName = "getMicroregionIdResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetMicroregionIdResponse")
public long getMicroregionId(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "coordX", targetNamespace = "")
double coordX,
@WebParam(name = "coordY", targetNamespace = "")
double coordY)
throws InvalidSessionException_Exception
;
/**
*
* @param sessionId
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listSpecialDeliveryRequirements", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListSpecialDeliveryRequirements")
@ResponseWrapper(localName = "listSpecialDeliveryRequirementsResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListSpecialDeliveryRequirementsResponse")
@Action(input = "http://ver01.eps.speedy.sirma.com/EPSProvider/listSpecialDeliveryRequirementsRequest", output = "http://ver01.eps.speedy.sirma.com/EPSProvider/listSpecialDeliveryRequirementsResponse", fault = {
@FaultAction(className = InvalidSessionException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/listSpecialDeliveryRequirements/Fault/InvalidSessionException")
})
public List listSpecialDeliveryRequirements(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId)
throws InvalidSessionException_Exception
;
/**
*
* @param address
* @param sessionId
* @param validationMode
* @return
* returns boolean
* @throws PickingValidationException_Exception
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "validateAddress", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ValidateAddress")
@ResponseWrapper(localName = "validateAddressResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ValidateAddressResponse")
@Action(input = "http://ver01.eps.speedy.sirma.com/EPSProvider/validateAddressRequest", output = "http://ver01.eps.speedy.sirma.com/EPSProvider/validateAddressResponse", fault = {
@FaultAction(className = InvalidSessionException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/validateAddress/Fault/InvalidSessionException"),
@FaultAction(className = PickingValidationException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/validateAddress/Fault/PickingValidationException")
})
public boolean validateAddress(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "address", targetNamespace = "")
ParamAddress address,
@WebParam(name = "validationMode", targetNamespace = "")
Integer validationMode)
throws InvalidSessionException_Exception, PickingValidationException_Exception
;
/**
*
* @param sessionId
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listContractClients", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListContractClients")
@ResponseWrapper(localName = "listContractClientsResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListContractClientsResponse")
@Action(input = "http://ver01.eps.speedy.sirma.com/EPSProvider/listContractClientsRequest", output = "http://ver01.eps.speedy.sirma.com/EPSProvider/listContractClientsResponse", fault = {
@FaultAction(className = InvalidSessionException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/listContractClients/Fault/InvalidSessionException")
})
public List listContractClients(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId)
throws InvalidSessionException_Exception
;
/**
*
* @param siteId
* @param sessionId
* @param name
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "listOfficesEx", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListOfficesEx")
@ResponseWrapper(localName = "listOfficesExResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.ListOfficesExResponse")
@Action(input = "http://ver01.eps.speedy.sirma.com/EPSProvider/listOfficesExRequest", output = "http://ver01.eps.speedy.sirma.com/EPSProvider/listOfficesExResponse", fault = {
@FaultAction(className = InvalidSessionException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/listOfficesEx/Fault/InvalidSessionException")
})
public List listOfficesEx(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "name", targetNamespace = "")
String name,
@WebParam(name = "siteId", targetNamespace = "")
Long siteId)
throws InvalidSessionException_Exception
;
/**
*
* @param address
* @param sessionId
* @return
* returns com.omgm.speedy.eps.soap.model.ParamAddress
* @throws PickingValidationException_Exception
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "deserializeAddress", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.DeserializeAddress")
@ResponseWrapper(localName = "deserializeAddressResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.DeserializeAddressResponse")
@Action(input = "http://ver01.eps.speedy.sirma.com/EPSProvider/deserializeAddressRequest", output = "http://ver01.eps.speedy.sirma.com/EPSProvider/deserializeAddressResponse", fault = {
@FaultAction(className = InvalidSessionException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/deserializeAddress/Fault/InvalidSessionException"),
@FaultAction(className = PickingValidationException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/deserializeAddress/Fault/PickingValidationException")
})
public ParamAddress deserializeAddress(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "address", targetNamespace = "")
String address)
throws InvalidSessionException_Exception, PickingValidationException_Exception
;
/**
*
* @param address
* @param sessionId
* @return
* returns java.lang.String
* @throws PickingValidationException_Exception
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "serializeAddress", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.SerializeAddress")
@ResponseWrapper(localName = "serializeAddressResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.SerializeAddressResponse")
@Action(input = "http://ver01.eps.speedy.sirma.com/EPSProvider/serializeAddressRequest", output = "http://ver01.eps.speedy.sirma.com/EPSProvider/serializeAddressResponse", fault = {
@FaultAction(className = InvalidSessionException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/serializeAddress/Fault/InvalidSessionException"),
@FaultAction(className = PickingValidationException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/serializeAddress/Fault/PickingValidationException")
})
public String serializeAddress(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "address", targetNamespace = "")
ParamAddress address)
throws InvalidSessionException_Exception, PickingValidationException_Exception
;
/**
*
* @param address
* @param sessionId
* @return
* returns com.omgm.speedy.eps.soap.model.ResultAddressString
* @throws PickingValidationException_Exception
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "makeAddressString", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.MakeAddressString")
@ResponseWrapper(localName = "makeAddressStringResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.MakeAddressStringResponse")
@Action(input = "http://ver01.eps.speedy.sirma.com/EPSProvider/makeAddressStringRequest", output = "http://ver01.eps.speedy.sirma.com/EPSProvider/makeAddressStringResponse", fault = {
@FaultAction(className = InvalidSessionException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/makeAddressString/Fault/InvalidSessionException"),
@FaultAction(className = PickingValidationException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/makeAddressString/Fault/PickingValidationException")
})
public ResultAddressString makeAddressString(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "address", targetNamespace = "")
ParamAddress address)
throws InvalidSessionException_Exception, PickingValidationException_Exception
;
/**
*
* @param sessionId
* @param date
* @return
* returns java.util.List
* @throws InvalidSessionException_Exception
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "getAdditionalUserParams", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetAdditionalUserParams")
@ResponseWrapper(localName = "getAdditionalUserParamsResponse", targetNamespace = "http://ver01.eps.speedy.sirma.com/", className = "com.omgm.speedy.eps.soap.model.GetAdditionalUserParamsResponse")
@Action(input = "http://ver01.eps.speedy.sirma.com/EPSProvider/getAdditionalUserParamsRequest", output = "http://ver01.eps.speedy.sirma.com/EPSProvider/getAdditionalUserParamsResponse", fault = {
@FaultAction(className = InvalidSessionException_Exception.class, value = "http://ver01.eps.speedy.sirma.com/EPSProvider/getAdditionalUserParams/Fault/InvalidSessionException")
})
public List getAdditionalUserParams(
@WebParam(name = "sessionId", targetNamespace = "")
String sessionId,
@WebParam(name = "date", targetNamespace = "")
XMLGregorianCalendar date)
throws InvalidSessionException_Exception
;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy