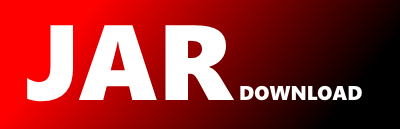
com.onemillionworlds.tamarin.openxr.InProgressXrRender Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tamarin Show documentation
Show all versions of tamarin Show documentation
A VR utilities library that extends the functionality in JMonkeyEngine
package com.onemillionworlds.tamarin.openxr;
import com.jme3.math.Matrix4f;
import com.jme3.math.Quaternion;
import com.jme3.math.Vector3f;
import com.jme3.texture.FrameBuffer;
/**
* We start a frame before the JME render and finish it after. This is a "continuation" that allows Xr to finish its
* work and also gives us the camera positions required to position the players eyes in the virtual world.
*/
public class InProgressXrRender {
public static EyePositionData NO_EYE_POSITION = new EyePositionData(new Vector3f(), new Quaternion().fromAngleNormalAxis(0, Vector3f.UNIT_Y), new FieldOfViewData(0, 0, 0, 0));
public static InProgressXrRender NO_XR_FRAME = new InProgressXrRender(false, false, 0, NO_EYE_POSITION, NO_EYE_POSITION, null, null);
boolean inProgressXr;
boolean shouldRender;
long predictedDisplayTime;
EyePositionData leftEye;
EyePositionData rightEye;
FrameBuffer leftBufferToRenderTo;
FrameBuffer rightBufferToRenderTo;
public record EyePositionData(Vector3f eyePosition, Quaternion eyeRotation, FieldOfViewData fieldOfView) {
public Matrix4f calculateProjectionMatrix(float nearClip, float farClip) {
return XrUtils.createProjectionMatrix(fieldOfView, nearClip, farClip);
}
}
/**
* The field of view data for a single eye. NOTE! the left and right angles are held seperately because the OPENXR runtime
* may request a non symmetric field of view. This is the case for the Oculus Quest 2. Do not just add left and right
* together and use that as the field of view.
* @param angleLeft angle in radians
* @param angleRight angle in radians
* @param angleUp angle in radians
* @param angleDown angle in radians
*/
public record FieldOfViewData(float angleLeft, float angleRight, float angleUp, float angleDown) {
}
public InProgressXrRender(final boolean inProgressXr, final boolean shouldRender, final long predictedDisplayTime, final EyePositionData leftEye, final EyePositionData rightEye, final FrameBuffer leftBufferToRenderTo, final FrameBuffer rightBufferToRenderTo) {
this.inProgressXr = inProgressXr;
this.shouldRender = shouldRender;
this.predictedDisplayTime = predictedDisplayTime;
this.leftEye = leftEye;
this.rightEye = rightEye;
this.leftBufferToRenderTo = leftBufferToRenderTo;
this.rightBufferToRenderTo = rightBufferToRenderTo;
}
public boolean isInProgressXr() {
return this.inProgressXr;
}
public boolean isShouldRender() {
return this.shouldRender;
}
public long getPredictedDisplayTime() {
return this.predictedDisplayTime;
}
public EyePositionData getLeftEye() {
return this.leftEye;
}
public EyePositionData getRightEye() {
return this.rightEye;
}
public FrameBuffer getLeftBufferToRenderTo() {
return this.leftBufferToRenderTo;
}
public FrameBuffer getRightBufferToRenderTo() {
return this.rightBufferToRenderTo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy