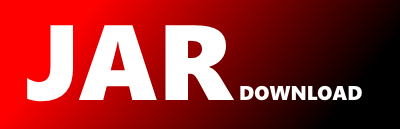
com.oneops.infoblox.$AutoValue_InfobloxClient Maven / Gradle / Ivy
package com.oneops.infoblox;
import com.oneops.infoblox.model.Redacted;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_InfobloxClient extends InfobloxClient {
private final String endPoint;
private final String wapiVersion;
private final String userName;
private final String password;
private final String dnsView;
private final boolean tlsVerify;
private final boolean debug;
private final int timeout;
$AutoValue_InfobloxClient(
String endPoint,
String wapiVersion,
String userName,
String password,
String dnsView,
boolean tlsVerify,
boolean debug,
int timeout) {
if (endPoint == null) {
throw new NullPointerException("Null endPoint");
}
this.endPoint = endPoint;
if (wapiVersion == null) {
throw new NullPointerException("Null wapiVersion");
}
this.wapiVersion = wapiVersion;
if (userName == null) {
throw new NullPointerException("Null userName");
}
this.userName = userName;
if (password == null) {
throw new NullPointerException("Null password");
}
this.password = password;
if (dnsView == null) {
throw new NullPointerException("Null dnsView");
}
this.dnsView = dnsView;
this.tlsVerify = tlsVerify;
this.debug = debug;
this.timeout = timeout;
}
@Override
public String endPoint() {
return endPoint;
}
@Override
public String wapiVersion() {
return wapiVersion;
}
@Redacted
@Override
public String userName() {
return userName;
}
@Redacted
@Override
public String password() {
return password;
}
@Override
public String dnsView() {
return dnsView;
}
@Override
public boolean tlsVerify() {
return tlsVerify;
}
@Override
public boolean debug() {
return debug;
}
@Override
public int timeout() {
return timeout;
}
@Override
public String toString() {
return "InfobloxClient{"
+ "endPoint=" + endPoint + ", "
+ "wapiVersion=" + wapiVersion + ", "
+ "userName=" + userName + ", "
+ "password=" + password + ", "
+ "dnsView=" + dnsView + ", "
+ "tlsVerify=" + tlsVerify + ", "
+ "debug=" + debug + ", "
+ "timeout=" + timeout
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof InfobloxClient) {
InfobloxClient that = (InfobloxClient) o;
return (this.endPoint.equals(that.endPoint()))
&& (this.wapiVersion.equals(that.wapiVersion()))
&& (this.userName.equals(that.userName()))
&& (this.password.equals(that.password()))
&& (this.dnsView.equals(that.dnsView()))
&& (this.tlsVerify == that.tlsVerify())
&& (this.debug == that.debug())
&& (this.timeout == that.timeout());
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.endPoint.hashCode();
h *= 1000003;
h ^= this.wapiVersion.hashCode();
h *= 1000003;
h ^= this.userName.hashCode();
h *= 1000003;
h ^= this.password.hashCode();
h *= 1000003;
h ^= this.dnsView.hashCode();
h *= 1000003;
h ^= this.tlsVerify ? 1231 : 1237;
h *= 1000003;
h ^= this.debug ? 1231 : 1237;
h *= 1000003;
h ^= this.timeout;
return h;
}
static final class Builder extends InfobloxClient.Builder {
private String endPoint;
private String wapiVersion;
private String userName;
private String password;
private String dnsView;
private Boolean tlsVerify;
private Boolean debug;
private Integer timeout;
Builder() {
}
@Override
public InfobloxClient.Builder endPoint(String endPoint) {
if (endPoint == null) {
throw new NullPointerException("Null endPoint");
}
this.endPoint = endPoint;
return this;
}
@Override
public InfobloxClient.Builder wapiVersion(String wapiVersion) {
if (wapiVersion == null) {
throw new NullPointerException("Null wapiVersion");
}
this.wapiVersion = wapiVersion;
return this;
}
@Override
public InfobloxClient.Builder userName(String userName) {
if (userName == null) {
throw new NullPointerException("Null userName");
}
this.userName = userName;
return this;
}
@Override
public InfobloxClient.Builder password(String password) {
if (password == null) {
throw new NullPointerException("Null password");
}
this.password = password;
return this;
}
@Override
public InfobloxClient.Builder dnsView(String dnsView) {
if (dnsView == null) {
throw new NullPointerException("Null dnsView");
}
this.dnsView = dnsView;
return this;
}
@Override
public InfobloxClient.Builder tlsVerify(boolean tlsVerify) {
this.tlsVerify = tlsVerify;
return this;
}
@Override
public InfobloxClient.Builder debug(boolean debug) {
this.debug = debug;
return this;
}
@Override
public InfobloxClient.Builder timeout(int timeout) {
this.timeout = timeout;
return this;
}
@Override
InfobloxClient autoBuild() {
String missing = "";
if (this.endPoint == null) {
missing += " endPoint";
}
if (this.wapiVersion == null) {
missing += " wapiVersion";
}
if (this.userName == null) {
missing += " userName";
}
if (this.password == null) {
missing += " password";
}
if (this.dnsView == null) {
missing += " dnsView";
}
if (this.tlsVerify == null) {
missing += " tlsVerify";
}
if (this.debug == null) {
missing += " debug";
}
if (this.timeout == null) {
missing += " timeout";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_InfobloxClient(
this.endPoint,
this.wapiVersion,
this.userName,
this.password,
this.dnsView,
this.tlsVerify,
this.debug,
this.timeout);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy