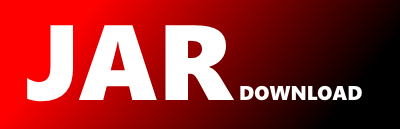
com.oneops.infoblox.curl.CurlLoggingInterceptor Maven / Gradle / Ivy
package com.oneops.infoblox.curl;
import java.io.IOException;
import java.nio.charset.Charset;
import okhttp3.Headers;
import okhttp3.Interceptor;
import okhttp3.MediaType;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
import okhttp3.logging.HttpLoggingInterceptor.Logger;
import okio.Buffer;
/**
* An OkHttp interceptor that logs requests as curl shell commands. They can then be copied, pasted
* and executed inside a terminal environment. This might be useful for troubleshooting
* client/server API interaction during development, making it easy to isolate and share requests
* made by the app.
*
* Warning: The logs generated by this interceptor have the potential to leak sensitive
* information. It should only be used in a controlled manner or in a non-production environment.
*
* @author Jeff Gilfelt
* @see Gist Link
*/
public class CurlLoggingInterceptor implements Interceptor {
private static final Charset UTF8 = Charset.forName("UTF-8");
private final Logger logger;
private String curlOptions;
public CurlLoggingInterceptor() {
this(Logger.DEFAULT);
}
public CurlLoggingInterceptor(Logger logger) {
this.logger = logger;
}
/** Set any additional curl command options (see 'curl --help'). */
public void curlOptions(String curlOptions) {
this.curlOptions = curlOptions;
}
@Override
public Response intercept(Chain chain) throws IOException {
Request request = chain.request();
StringBuilder curlCmd = new StringBuilder("curl");
if (curlOptions != null) {
curlCmd.append(" ").append(curlOptions);
}
curlCmd.append(" -X ").append(request.method());
boolean compressed = false;
Headers headers = request.headers();
for (int i = 0; i < headers.size(); i++) {
String name = headers.name(i);
String value = headers.value(i);
if ("Accept-Encoding".equalsIgnoreCase(name) && "gzip".equalsIgnoreCase(value)) {
compressed = true;
}
// Exclude the Http Auth and Cookie headers from logging.
if (name.toLowerCase().contains("authorization") || "Cookie".equalsIgnoreCase(name)) {
value = "██";
}
curlCmd.append(" -H " + "\"").append(name).append(": ").append(value).append("\"");
}
RequestBody requestBody = request.body();
if (requestBody != null) {
Buffer buffer = new Buffer();
requestBody.writeTo(buffer);
Charset charset = UTF8;
MediaType contentType = requestBody.contentType();
if (contentType != null) {
charset = contentType.charset(UTF8);
}
// Try to keep to a single line and use a subshell to preserve any line breaks.
curlCmd
.append(" --data $'")
.append(buffer.readString(charset).replace("\n", "\\n"))
.append("'");
}
curlCmd.append((compressed) ? " --compressed " : " ").append(request.url());
logger.log(String.format("(cURL) --> %s", curlCmd.toString()));
return chain.proceed(request);
}
}