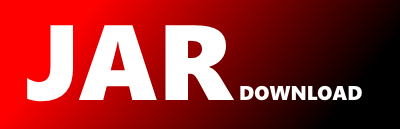
com.onevizion.uitest.api.helper.Checkbox Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ui-test-api Show documentation
Show all versions of ui-test-api Show documentation
An API for easily write tests for OneVizion platform
The newest version!
package com.onevizion.uitest.api.helper;
import java.util.ArrayList;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.onevizion.uitest.api.SeleniumSettings;
@Component
public class Checkbox {
@Autowired
private Js js;
@Autowired
private SeleniumSettings seleniumSettings;
public void clickByName(String checkboxName) {
WebElement checkbox = seleniumSettings.getWebDriver().findElement(By.name(checkboxName));
WebElement label = js.getParentElement(checkbox);
label.click();
}
public void clickById(String checkboxId) {
WebElement checkbox = seleniumSettings.getWebDriver().findElement(By.id(checkboxId));
WebElement label = js.getParentElement(checkbox);
if (label.isDisplayed()) {
label.click();
}
}
public void clickByElement(WebElement checkbox) {
WebElement label = js.getParentElement(checkbox);
label.click();
}
public List findCheckboxesByName(String checkboxname) {
return seleniumSettings.getWebDriver().findElements(By.name(checkboxname));
}
public List findLabelsByName(String checkboxname) {
List checkboxes = seleniumSettings.getWebDriver().findElements(By.name(checkboxname));
List labels = new ArrayList<>();
for (WebElement checkbox : checkboxes) {
WebElement label = js.getParentElement(checkbox);
labels.add(label);
}
return labels;
}
public WebElement findLabelByName(String checkboxname) {
WebElement checkbox = seleniumSettings.getWebDriver().findElement(By.name(checkboxname));
return js.getParentElement(checkbox);
}
public WebElement findLabelByElement(WebElement checkbox) {
return js.getParentElement(checkbox);
}
public boolean isElementChecked(WebElement webElement) {
String checked = webElement.getAttribute("checked");
return checked != null && checked.equals("true");
}
public boolean isCheckedByName(String checkboxName) {
String checked = seleniumSettings.getWebDriver().findElement(By.name(checkboxName)).getAttribute("checked");
return checked != null && checked.equals("true");
}
public boolean isCheckedById(String checkboxId) {
String checked = seleniumSettings.getWebDriver().findElement(By.id(checkboxId)).getAttribute("checked");
return checked != null && checked.equals("true");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy