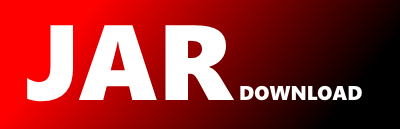
com.onevizion.uitest.api.helper.wiki.FckEditor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ui-test-api Show documentation
Show all versions of ui-test-api Show documentation
An API for easily write tests for OneVizion platform
The newest version!
package com.onevizion.uitest.api.helper.wiki;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.interactions.Actions;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.testng.Assert;
import com.onevizion.uitest.api.AbstractSeleniumCore;
import com.onevizion.uitest.api.SeleniumScreenshot;
import com.onevizion.uitest.api.SeleniumSettings;
import com.onevizion.uitest.api.helper.Element;
import com.onevizion.uitest.api.helper.Wait;
import com.onevizion.uitest.api.helper.clipboard.Clipboard;
@Component
public class FckEditor {
@Autowired
private SeleniumSettings seleniumSettings;
@Autowired
private Wait wait;
@Autowired
private Element element;
@Autowired
private FckEditorWait fckEditorWait;
@Autowired
private Clipboard clipboard;
@Autowired
private SeleniumScreenshot seleniumScreenshot;
public void setValue(String name, String value) {
if (value.startsWith("") && value.endsWith("
")) {
value = value.substring(3, value.length() - 4);
}
wait.waitWebElement(By.id(name));
wait.waitWebElement(By.id("cke_" + name));
element.moveToElementById("cke_" + name);
fckEditorWait.waitReady(name);
WebElement div = seleniumSettings.getWebDriver().findElement(By.id("cke_" + name));
WebElement iframe = div.findElement(By.tagName("iframe"));
seleniumSettings.getWebDriver().switchTo().frame(iframe);
WebElement body = seleniumSettings.getWebDriver().findElement(By.tagName("body"));
body.click();
String prevVal = body.getAttribute("innerHTML");
Actions actionObject = new Actions(seleniumSettings.getWebDriver());
for (int i = 0; i < prevVal.length(); i++) {
body.click();
actionObject.sendKeys(Keys.ARROW_RIGHT).perform();
actionObject.sendKeys(Keys.BACK_SPACE).perform();
}
if (value.length() > 0) {
actionObject.sendKeys(value).perform();
actionObject.sendKeys(" ").perform();
actionObject.sendKeys(Keys.BACK_SPACE).perform();
}
seleniumSettings.getWebDriver().switchTo().parentFrame();
}
public void setValueFromClipboard(String name, String value) {
clipboard.pasteTextToClipboard(value);
wait.waitWebElement(By.id(name));
wait.waitWebElement(By.id("cke_" + name));
element.moveToElementById("cke_" + name);
fckEditorWait.waitReady(name);
WebElement div = seleniumSettings.getWebDriver().findElement(By.id("cke_" + name));
WebElement iframe = div.findElement(By.tagName("iframe"));
seleniumSettings.getWebDriver().switchTo().frame(iframe);
AbstractSeleniumCore.sleep(1000L);
seleniumSettings.getWebDriver().findElement(By.tagName("body")).click();
AbstractSeleniumCore.sleep(1000L);
Actions action = new Actions(seleniumSettings.getWebDriver());
seleniumScreenshot.getScreenshot();
action.keyDown(Keys.CONTROL).sendKeys("a").keyUp(Keys.CONTROL).perform();
seleniumScreenshot.getScreenshot();
action.sendKeys(Keys.BACK_SPACE).perform();
seleniumScreenshot.getScreenshot();
action.keyDown(Keys.CONTROL).sendKeys("v").keyUp(Keys.CONTROL).perform();
seleniumScreenshot.getScreenshot();
seleniumSettings.getWebDriver().switchTo().parentFrame();
}
public void checkValue(String name, String expectedValue) {
if (expectedValue.startsWith("") && expectedValue.endsWith("
")) {
expectedValue = expectedValue.substring(3, expectedValue.length() - 4);
}
wait.waitWebElement(By.id(name));
wait.waitWebElement(By.id("cke_" + name));
element.moveToElementById("cke_" + name);
fckEditorWait.waitReady(name);
WebElement div = seleniumSettings.getWebDriver().findElement(By.id("cke_" + name));
WebElement iframe = div.findElement(By.tagName("iframe"));
seleniumSettings.getWebDriver().switchTo().frame(iframe);
String actualValue = seleniumSettings.getWebDriver().findElement(By.tagName("body")).getAttribute("innerHTML").trim();
seleniumSettings.getWebDriver().switchTo().parentFrame();
actualValue = actualValue.replaceAll(AbstractSeleniumCore.SPECIAL_CHARACTERS_ENCODED_1, AbstractSeleniumCore.SPECIAL_CHARACTERS_1);
actualValue = actualValue.replaceAll(AbstractSeleniumCore.SPECIAL_CHARACTERS_ENCODED_2, AbstractSeleniumCore.SPECIAL_CHARACTERS_2);
actualValue = actualValue.replaceAll(AbstractSeleniumCore.SPECIAL_CHARACTERS_ENCODED_3, AbstractSeleniumCore.SPECIAL_CHARACTERS_3);
actualValue = actualValue.replaceAll(AbstractSeleniumCore.SPECIAL_CHARACTERS_ENCODED_4, AbstractSeleniumCore.SPECIAL_CHARACTERS_4);
if ("
".equals(actualValue) || "
".equals(actualValue)) {
actualValue = "";
}
if (actualValue.startsWith("") && actualValue.endsWith("
")) {
actualValue = actualValue.substring(3, actualValue.length() - 8);
}
if (actualValue.startsWith("") && actualValue.endsWith("
")) {
actualValue = actualValue.substring(3, actualValue.length() - 4);
}
Assert.assertEquals(actualValue, expectedValue, "Element with name=[" + name + "] has wrong value");
}
public void checkValueReadOnly(String name, String expectedValue) {
seleniumSettings.getWebDriver().switchTo().frame(name);
String actualValue = seleniumSettings.getWebDriver().findElement(By.tagName("body")).getAttribute("innerHTML").trim();
seleniumSettings.getWebDriver().switchTo().parentFrame();
actualValue = actualValue.replaceAll(AbstractSeleniumCore.SPECIAL_CHARACTERS_ENCODED_1, AbstractSeleniumCore.SPECIAL_CHARACTERS_1);
actualValue = actualValue.replaceAll(AbstractSeleniumCore.SPECIAL_CHARACTERS_ENCODED_2, AbstractSeleniumCore.SPECIAL_CHARACTERS_2);
actualValue = actualValue.replaceAll(AbstractSeleniumCore.SPECIAL_CHARACTERS_ENCODED_3, AbstractSeleniumCore.SPECIAL_CHARACTERS_3);
actualValue = actualValue.replaceAll(AbstractSeleniumCore.SPECIAL_CHARACTERS_ENCODED_4, AbstractSeleniumCore.SPECIAL_CHARACTERS_4);
Assert.assertEquals(actualValue, expectedValue, "Element with name=[" + name + "] has wrong value");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy