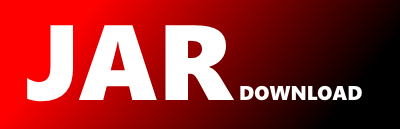
com.onfido.api.client.MultipartLiveVideoRequestBuilder.kt Maven / Gradle / Ivy
package com.onfido.api.client
import com.onfido.api.client.data.LiveVideoChallenges
import com.onfido.api.client.data.LiveVideoLanguage
import com.onfido.api.client.data.PayloadIntegrity
import com.onfido.api.client.data.SdkUploadMetaData
import kotlinx.serialization.encodeToString
import okhttp3.MultipartBody
internal class MultipartLiveVideoRequestBuilder(sdkSource: String, sdkVersion: String) :
MultiPartRequestBuilder(sdkSource, sdkVersion) {
@Suppress("LongParameterList")
fun setMultipartRequestBody(
fileName: String,
fileType: String,
data: ByteArray,
challengeId: String,
challenges: List,
challengeSwitchTimestamp: Long,
languages: List,
sdkUploadMetaData: SdkUploadMetaData?,
payloadIntegrity: PayloadIntegrity?
): MultipartBody.Builder {
setChallengeId(challengeId)
setChallenges(getJsonParserInstance().encodeToString(challenges))
setFile(fileName, fileType, data)
setChallengeSwitch(challengeSwitchTimestamp)
setLanguages(getJsonParserInstance().encodeToString(languages))
setSdkMetadata(sdkUploadMetaData)
if (payloadIntegrity != null && !payloadIntegrity.isEmpty()) {
// these two won't be null guaranteed by the isEmpty call above
setSignature(payloadIntegrity.signatureBase64!!)
setClientNonce(payloadIntegrity.clientNonce!!)
}
return super.getBuilder()
}
private fun setChallenges(challengesRepresentation: String) {
setFormData(CHALLENGES_KEY, challengesRepresentation)
}
private fun setChallengeSwitch(challengeSwitchTimestamp: Long) {
setFormData(CHALLENGE_SWITCH_KEY, challengeSwitchTimestamp.toString())
}
private fun setChallengeId(id: String) {
setFormData(CHALLENGES_ID_KEY, id)
}
private fun setLanguages(localeCode: String) {
setFormData(LANGUAGE_KEY, localeCode)
}
companion object {
private const val CHALLENGES_ID_KEY = "challenge_id"
private const val CHALLENGES_KEY = "challenge"
private const val CHALLENGE_SWITCH_KEY = "challenge_switch_at"
private const val LANGUAGE_KEY = "languages"
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy