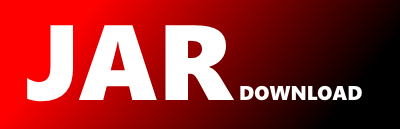
com.onfido.api.client.OnfidoService Maven / Gradle / Ivy
package com.onfido.api.client;
import com.onfido.api.client.data.DocumentMediaUploadResponse;
import com.onfido.api.client.data.DocumentUpload;
import com.onfido.api.client.data.LivePhotoUpload;
import com.onfido.api.client.data.LiveVideoChallenges;
import com.onfido.api.client.data.LiveVideoUpload;
import com.onfido.api.client.data.NfcProperties;
import com.onfido.api.client.data.NfcPropertiesRequest;
import com.onfido.api.client.data.PoaDocumentUpload;
import com.onfido.api.client.data.SdkConfiguration;
import com.onfido.api.client.data.SdkConfigurationRequestBody;
import com.onfido.api.client.data.SupportedDocuments;
import com.onfido.api.client.interceptor.API;
import io.reactivex.rxjava3.core.Completable;
import io.reactivex.rxjava3.core.Single;
import okhttp3.RequestBody;
import retrofit2.Call;
import retrofit2.http.Body;
import retrofit2.http.GET;
import retrofit2.http.Header;
import retrofit2.http.POST;
interface OnfidoService {
// API V3
@API(version = "v3.6")
@POST(Url.DOCUMENT_UPLOAD)
Call upload(
@Body RequestBody body
);
@API(version = "v3.6")
@POST(Url.DOCUMENT_UPLOAD)
Single uploadSingle(@Body RequestBody body);
@API(version = "v3.6")
@POST(Url.DOCUMENT_UPLOAD)
Single uploadPoa(@Body RequestBody body);
@API(version = "v3.6")
@POST(Url.DOCUMENT_UPLOAD)
Single uploadDocumentVideo(
@Header(HeaderKey.MEDIA_SIGNATURE) String mediaSignature,
@Header(HeaderKey.CLIENT_NONCE) String clientNonce,
@Body RequestBody body
);
@API(version = "v3.6")
@POST(Url.LIVE_PHOTO_UPLOAD)
Call uploadLivePhoto(@Body RequestBody body);
@API(version = "v3.6")
@POST(Url.LIVE_VIDEO_UPLOAD)
Single uploadLiveVideo(@Body RequestBody body);
@API(version = "v3.6")
@POST(Url.LIVE_VIDEO_CHALLENGE)
Single getLiveVideoChallenges();
// API V4
@API(version = "v4")
@POST(Url.DOCUMENT_MEDIA_UPLOAD)
Single uploadMediaFile(@Header(HeaderKey.VIDEO_AUTH) String videoAuth,
@Body RequestBody body);
@API(version = "v3.5")
@POST(Url.SDK_CONFIGURATION)
Single getSdkConfiguration(@Body SdkConfigurationRequestBody body);
@API(version = "v3.6")
@GET(Url.ALL_SUPPORTED_DOCUMENTS)
Single getSupportedDocuments();
@API(version = "v4")
@POST(Url.NFC_PROPERTIES)
Single getNfcProperties(@Body NfcPropertiesRequest nfcPropertiesRequest);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy