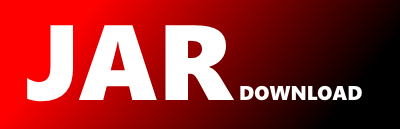
com.onfido.model.DocumentProperties Maven / Gradle / Ivy
/*
* Onfido API v3.6
* The Onfido API (v3.6)
*
* The version of the OpenAPI document: v3.6
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.onfido.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.onfido.model.DocumentPropertiesAddressLines;
import com.onfido.model.DocumentPropertiesBarcodeInner;
import com.onfido.model.DocumentPropertiesDocumentClassification;
import com.onfido.model.DocumentPropertiesDocumentNumbersInner;
import com.onfido.model.DocumentPropertiesDrivingLicenceInformation;
import com.onfido.model.DocumentPropertiesExtractedData;
import com.onfido.model.DocumentPropertiesNfc;
import java.io.IOException;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.onfido.JSON;
/**
* DocumentProperties
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.9.0")
public class DocumentProperties {
public static final String SERIALIZED_NAME_DATE_OF_BIRTH = "date_of_birth";
@SerializedName(SERIALIZED_NAME_DATE_OF_BIRTH)
private LocalDate dateOfBirth;
public static final String SERIALIZED_NAME_DATE_OF_EXPIRY = "date_of_expiry";
@SerializedName(SERIALIZED_NAME_DATE_OF_EXPIRY)
private LocalDate dateOfExpiry;
public static final String SERIALIZED_NAME_PERSONAL_NUMBER = "personal_number";
@SerializedName(SERIALIZED_NAME_PERSONAL_NUMBER)
private String personalNumber;
public static final String SERIALIZED_NAME_DOCUMENT_NUMBERS = "document_numbers";
@SerializedName(SERIALIZED_NAME_DOCUMENT_NUMBERS)
private List documentNumbers = new ArrayList<>();
public static final String SERIALIZED_NAME_DOCUMENT_TYPE = "document_type";
@SerializedName(SERIALIZED_NAME_DOCUMENT_TYPE)
private String documentType;
public static final String SERIALIZED_NAME_FIRST_NAME = "first_name";
@SerializedName(SERIALIZED_NAME_FIRST_NAME)
private String firstName;
public static final String SERIALIZED_NAME_MIDDLE_NAME = "middle_name";
@SerializedName(SERIALIZED_NAME_MIDDLE_NAME)
private String middleName;
public static final String SERIALIZED_NAME_LAST_NAME = "last_name";
@SerializedName(SERIALIZED_NAME_LAST_NAME)
private String lastName;
public static final String SERIALIZED_NAME_GENDER = "gender";
@SerializedName(SERIALIZED_NAME_GENDER)
private String gender;
public static final String SERIALIZED_NAME_ISSUING_COUNTRY = "issuing_country";
@SerializedName(SERIALIZED_NAME_ISSUING_COUNTRY)
private String issuingCountry;
public static final String SERIALIZED_NAME_NATIONALITY = "nationality";
@SerializedName(SERIALIZED_NAME_NATIONALITY)
private String nationality;
public static final String SERIALIZED_NAME_ISSUING_STATE = "issuing_state";
@SerializedName(SERIALIZED_NAME_ISSUING_STATE)
private String issuingState;
public static final String SERIALIZED_NAME_ISSUING_DATE = "issuing_date";
@SerializedName(SERIALIZED_NAME_ISSUING_DATE)
private LocalDate issuingDate;
public static final String SERIALIZED_NAME_CATEGORISATION = "categorisation";
@SerializedName(SERIALIZED_NAME_CATEGORISATION)
private String categorisation;
public static final String SERIALIZED_NAME_MRZ_LINE1 = "mrz_line1";
@SerializedName(SERIALIZED_NAME_MRZ_LINE1)
private String mrzLine1;
public static final String SERIALIZED_NAME_MRZ_LINE2 = "mrz_line2";
@SerializedName(SERIALIZED_NAME_MRZ_LINE2)
private String mrzLine2;
public static final String SERIALIZED_NAME_MRZ_LINE3 = "mrz_line3";
@SerializedName(SERIALIZED_NAME_MRZ_LINE3)
private String mrzLine3;
public static final String SERIALIZED_NAME_ADDRESS = "address";
@SerializedName(SERIALIZED_NAME_ADDRESS)
private String address;
public static final String SERIALIZED_NAME_PLACE_OF_BIRTH = "place_of_birth";
@SerializedName(SERIALIZED_NAME_PLACE_OF_BIRTH)
private String placeOfBirth;
public static final String SERIALIZED_NAME_SPOUSE_NAME = "spouse_name";
@SerializedName(SERIALIZED_NAME_SPOUSE_NAME)
private String spouseName;
public static final String SERIALIZED_NAME_WIDOW_NAME = "widow_name";
@SerializedName(SERIALIZED_NAME_WIDOW_NAME)
private String widowName;
public static final String SERIALIZED_NAME_ALIAS_NAME = "alias_name";
@SerializedName(SERIALIZED_NAME_ALIAS_NAME)
private String aliasName;
public static final String SERIALIZED_NAME_ISSUING_AUTHORITY = "issuing_authority";
@SerializedName(SERIALIZED_NAME_ISSUING_AUTHORITY)
private String issuingAuthority;
public static final String SERIALIZED_NAME_REMARKS = "remarks";
@SerializedName(SERIALIZED_NAME_REMARKS)
private String remarks;
public static final String SERIALIZED_NAME_CIVIL_STATE = "civil_state";
@SerializedName(SERIALIZED_NAME_CIVIL_STATE)
private String civilState;
public static final String SERIALIZED_NAME_EXPATRIATION = "expatriation";
@SerializedName(SERIALIZED_NAME_EXPATRIATION)
private String expatriation;
public static final String SERIALIZED_NAME_FATHER_NAME = "father_name";
@SerializedName(SERIALIZED_NAME_FATHER_NAME)
private String fatherName;
public static final String SERIALIZED_NAME_MOTHER_NAME = "mother_name";
@SerializedName(SERIALIZED_NAME_MOTHER_NAME)
private String motherName;
public static final String SERIALIZED_NAME_RELIGION = "religion";
@SerializedName(SERIALIZED_NAME_RELIGION)
private String religion;
public static final String SERIALIZED_NAME_TYPE_OF_PERMIT = "type_of_permit";
@SerializedName(SERIALIZED_NAME_TYPE_OF_PERMIT)
private String typeOfPermit;
public static final String SERIALIZED_NAME_VERSION_NUMBER = "version_number";
@SerializedName(SERIALIZED_NAME_VERSION_NUMBER)
private String versionNumber;
public static final String SERIALIZED_NAME_DOCUMENT_SUBTYPE = "document_subtype";
@SerializedName(SERIALIZED_NAME_DOCUMENT_SUBTYPE)
private String documentSubtype;
public static final String SERIALIZED_NAME_PROFESSION = "profession";
@SerializedName(SERIALIZED_NAME_PROFESSION)
private String profession;
public static final String SERIALIZED_NAME_SECURITY_DOCUMENT_NUMBER = "security_document_number";
@SerializedName(SERIALIZED_NAME_SECURITY_DOCUMENT_NUMBER)
private String securityDocumentNumber;
public static final String SERIALIZED_NAME_TAX_NUMBER = "tax_number";
@SerializedName(SERIALIZED_NAME_TAX_NUMBER)
private String taxNumber;
/**
* Gets or Sets nistIdentityEvidenceStrength
*/
@JsonAdapter(NistIdentityEvidenceStrengthEnum.Adapter.class)
public enum NistIdentityEvidenceStrengthEnum {
SUPERIOR("superior"),
STRONG("strong"),
FAIR("fair"),
WEAK("weak"),
UNACCEPTABLE("unacceptable"),
UNSPECIFIED_IDENTITY_EVIDENCE_STRENGTH("unspecified_identity_evidence_strength"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
NistIdentityEvidenceStrengthEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static NistIdentityEvidenceStrengthEnum fromValue(String value) {
for (NistIdentityEvidenceStrengthEnum b : NistIdentityEvidenceStrengthEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final NistIdentityEvidenceStrengthEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public NistIdentityEvidenceStrengthEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return NistIdentityEvidenceStrengthEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
NistIdentityEvidenceStrengthEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_NIST_IDENTITY_EVIDENCE_STRENGTH = "nist_identity_evidence_strength";
@SerializedName(SERIALIZED_NAME_NIST_IDENTITY_EVIDENCE_STRENGTH)
private NistIdentityEvidenceStrengthEnum nistIdentityEvidenceStrength;
/**
* Gets or Sets hasIssuanceConfirmation
*/
@JsonAdapter(HasIssuanceConfirmationEnum.Adapter.class)
public enum HasIssuanceConfirmationEnum {
TRUE("true"),
FALSE("false"),
UNSPECIFIED("unspecified"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
HasIssuanceConfirmationEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static HasIssuanceConfirmationEnum fromValue(String value) {
for (HasIssuanceConfirmationEnum b : HasIssuanceConfirmationEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final HasIssuanceConfirmationEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public HasIssuanceConfirmationEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return HasIssuanceConfirmationEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
HasIssuanceConfirmationEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_HAS_ISSUANCE_CONFIRMATION = "has_issuance_confirmation";
@SerializedName(SERIALIZED_NAME_HAS_ISSUANCE_CONFIRMATION)
private HasIssuanceConfirmationEnum hasIssuanceConfirmation;
public static final String SERIALIZED_NAME_REAL_ID_COMPLIANCE = "real_id_compliance";
@SerializedName(SERIALIZED_NAME_REAL_ID_COMPLIANCE)
private Boolean realIdCompliance;
/**
* Gets or Sets securityTier
*/
@JsonAdapter(SecurityTierEnum.Adapter.class)
public enum SecurityTierEnum {
TIER_1("tier_1"),
TIER_2("tier_2"),
TIER_3("tier_3"),
TIER_4("tier_4"),
TIER_5("tier_5"),
UNSPECIFIED_SECURITY_TIER("unspecified_security_tier"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
SecurityTierEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static SecurityTierEnum fromValue(String value) {
for (SecurityTierEnum b : SecurityTierEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final SecurityTierEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public SecurityTierEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return SecurityTierEnum.fromValue(value);
}
}
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
String value = jsonElement.getAsString();
SecurityTierEnum.fromValue(value);
}
}
public static final String SERIALIZED_NAME_SECURITY_TIER = "security_tier";
@SerializedName(SERIALIZED_NAME_SECURITY_TIER)
private SecurityTierEnum securityTier;
public static final String SERIALIZED_NAME_ADDRESS_LINES = "address_lines";
@SerializedName(SERIALIZED_NAME_ADDRESS_LINES)
private DocumentPropertiesAddressLines addressLines;
public static final String SERIALIZED_NAME_BARCODE = "barcode";
@SerializedName(SERIALIZED_NAME_BARCODE)
private List barcode = new ArrayList<>();
public static final String SERIALIZED_NAME_NFC = "nfc";
@SerializedName(SERIALIZED_NAME_NFC)
private DocumentPropertiesNfc nfc;
public static final String SERIALIZED_NAME_DRIVING_LICENCE_INFORMATION = "driving_licence_information";
@SerializedName(SERIALIZED_NAME_DRIVING_LICENCE_INFORMATION)
private DocumentPropertiesDrivingLicenceInformation drivingLicenceInformation;
public static final String SERIALIZED_NAME_DOCUMENT_CLASSIFICATION = "document_classification";
@SerializedName(SERIALIZED_NAME_DOCUMENT_CLASSIFICATION)
private DocumentPropertiesDocumentClassification documentClassification;
public static final String SERIALIZED_NAME_EXTRACTED_DATA = "extracted_data";
@SerializedName(SERIALIZED_NAME_EXTRACTED_DATA)
private DocumentPropertiesExtractedData extractedData;
public DocumentProperties() {
}
public DocumentProperties dateOfBirth(LocalDate dateOfBirth) {
this.dateOfBirth = dateOfBirth;
return this;
}
/**
* Get dateOfBirth
* @return dateOfBirth
*/
@javax.annotation.Nullable
public LocalDate getDateOfBirth() {
return dateOfBirth;
}
public void setDateOfBirth(LocalDate dateOfBirth) {
this.dateOfBirth = dateOfBirth;
}
public DocumentProperties dateOfExpiry(LocalDate dateOfExpiry) {
this.dateOfExpiry = dateOfExpiry;
return this;
}
/**
* Get dateOfExpiry
* @return dateOfExpiry
*/
@javax.annotation.Nullable
public LocalDate getDateOfExpiry() {
return dateOfExpiry;
}
public void setDateOfExpiry(LocalDate dateOfExpiry) {
this.dateOfExpiry = dateOfExpiry;
}
public DocumentProperties personalNumber(String personalNumber) {
this.personalNumber = personalNumber;
return this;
}
/**
* Get personalNumber
* @return personalNumber
*/
@javax.annotation.Nullable
public String getPersonalNumber() {
return personalNumber;
}
public void setPersonalNumber(String personalNumber) {
this.personalNumber = personalNumber;
}
public DocumentProperties documentNumbers(List documentNumbers) {
this.documentNumbers = documentNumbers;
return this;
}
public DocumentProperties addDocumentNumbersItem(DocumentPropertiesDocumentNumbersInner documentNumbersItem) {
if (this.documentNumbers == null) {
this.documentNumbers = new ArrayList<>();
}
this.documentNumbers.add(documentNumbersItem);
return this;
}
/**
* Get documentNumbers
* @return documentNumbers
*/
@javax.annotation.Nullable
public List getDocumentNumbers() {
return documentNumbers;
}
public void setDocumentNumbers(List documentNumbers) {
this.documentNumbers = documentNumbers;
}
public DocumentProperties documentType(String documentType) {
this.documentType = documentType;
return this;
}
/**
* Get documentType
* @return documentType
*/
@javax.annotation.Nullable
public String getDocumentType() {
return documentType;
}
public void setDocumentType(String documentType) {
this.documentType = documentType;
}
public DocumentProperties firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* Get firstName
* @return firstName
*/
@javax.annotation.Nullable
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public DocumentProperties middleName(String middleName) {
this.middleName = middleName;
return this;
}
/**
* Get middleName
* @return middleName
*/
@javax.annotation.Nullable
public String getMiddleName() {
return middleName;
}
public void setMiddleName(String middleName) {
this.middleName = middleName;
}
public DocumentProperties lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* Get lastName
* @return lastName
*/
@javax.annotation.Nullable
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public DocumentProperties gender(String gender) {
this.gender = gender;
return this;
}
/**
* Get gender
* @return gender
*/
@javax.annotation.Nullable
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public DocumentProperties issuingCountry(String issuingCountry) {
this.issuingCountry = issuingCountry;
return this;
}
/**
* Get issuingCountry
* @return issuingCountry
*/
@javax.annotation.Nullable
public String getIssuingCountry() {
return issuingCountry;
}
public void setIssuingCountry(String issuingCountry) {
this.issuingCountry = issuingCountry;
}
public DocumentProperties nationality(String nationality) {
this.nationality = nationality;
return this;
}
/**
* Get nationality
* @return nationality
*/
@javax.annotation.Nullable
public String getNationality() {
return nationality;
}
public void setNationality(String nationality) {
this.nationality = nationality;
}
public DocumentProperties issuingState(String issuingState) {
this.issuingState = issuingState;
return this;
}
/**
* Get issuingState
* @return issuingState
*/
@javax.annotation.Nullable
public String getIssuingState() {
return issuingState;
}
public void setIssuingState(String issuingState) {
this.issuingState = issuingState;
}
public DocumentProperties issuingDate(LocalDate issuingDate) {
this.issuingDate = issuingDate;
return this;
}
/**
* Get issuingDate
* @return issuingDate
*/
@javax.annotation.Nullable
public LocalDate getIssuingDate() {
return issuingDate;
}
public void setIssuingDate(LocalDate issuingDate) {
this.issuingDate = issuingDate;
}
public DocumentProperties categorisation(String categorisation) {
this.categorisation = categorisation;
return this;
}
/**
* Get categorisation
* @return categorisation
*/
@javax.annotation.Nullable
public String getCategorisation() {
return categorisation;
}
public void setCategorisation(String categorisation) {
this.categorisation = categorisation;
}
public DocumentProperties mrzLine1(String mrzLine1) {
this.mrzLine1 = mrzLine1;
return this;
}
/**
* Get mrzLine1
* @return mrzLine1
*/
@javax.annotation.Nullable
public String getMrzLine1() {
return mrzLine1;
}
public void setMrzLine1(String mrzLine1) {
this.mrzLine1 = mrzLine1;
}
public DocumentProperties mrzLine2(String mrzLine2) {
this.mrzLine2 = mrzLine2;
return this;
}
/**
* Get mrzLine2
* @return mrzLine2
*/
@javax.annotation.Nullable
public String getMrzLine2() {
return mrzLine2;
}
public void setMrzLine2(String mrzLine2) {
this.mrzLine2 = mrzLine2;
}
public DocumentProperties mrzLine3(String mrzLine3) {
this.mrzLine3 = mrzLine3;
return this;
}
/**
* Get mrzLine3
* @return mrzLine3
*/
@javax.annotation.Nullable
public String getMrzLine3() {
return mrzLine3;
}
public void setMrzLine3(String mrzLine3) {
this.mrzLine3 = mrzLine3;
}
public DocumentProperties address(String address) {
this.address = address;
return this;
}
/**
* Get address
* @return address
*/
@javax.annotation.Nullable
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public DocumentProperties placeOfBirth(String placeOfBirth) {
this.placeOfBirth = placeOfBirth;
return this;
}
/**
* Get placeOfBirth
* @return placeOfBirth
*/
@javax.annotation.Nullable
public String getPlaceOfBirth() {
return placeOfBirth;
}
public void setPlaceOfBirth(String placeOfBirth) {
this.placeOfBirth = placeOfBirth;
}
public DocumentProperties spouseName(String spouseName) {
this.spouseName = spouseName;
return this;
}
/**
* Get spouseName
* @return spouseName
*/
@javax.annotation.Nullable
public String getSpouseName() {
return spouseName;
}
public void setSpouseName(String spouseName) {
this.spouseName = spouseName;
}
public DocumentProperties widowName(String widowName) {
this.widowName = widowName;
return this;
}
/**
* Get widowName
* @return widowName
*/
@javax.annotation.Nullable
public String getWidowName() {
return widowName;
}
public void setWidowName(String widowName) {
this.widowName = widowName;
}
public DocumentProperties aliasName(String aliasName) {
this.aliasName = aliasName;
return this;
}
/**
* Get aliasName
* @return aliasName
*/
@javax.annotation.Nullable
public String getAliasName() {
return aliasName;
}
public void setAliasName(String aliasName) {
this.aliasName = aliasName;
}
public DocumentProperties issuingAuthority(String issuingAuthority) {
this.issuingAuthority = issuingAuthority;
return this;
}
/**
* Get issuingAuthority
* @return issuingAuthority
*/
@javax.annotation.Nullable
public String getIssuingAuthority() {
return issuingAuthority;
}
public void setIssuingAuthority(String issuingAuthority) {
this.issuingAuthority = issuingAuthority;
}
public DocumentProperties remarks(String remarks) {
this.remarks = remarks;
return this;
}
/**
* Get remarks
* @return remarks
*/
@javax.annotation.Nullable
public String getRemarks() {
return remarks;
}
public void setRemarks(String remarks) {
this.remarks = remarks;
}
public DocumentProperties civilState(String civilState) {
this.civilState = civilState;
return this;
}
/**
* Get civilState
* @return civilState
*/
@javax.annotation.Nullable
public String getCivilState() {
return civilState;
}
public void setCivilState(String civilState) {
this.civilState = civilState;
}
public DocumentProperties expatriation(String expatriation) {
this.expatriation = expatriation;
return this;
}
/**
* Get expatriation
* @return expatriation
*/
@javax.annotation.Nullable
public String getExpatriation() {
return expatriation;
}
public void setExpatriation(String expatriation) {
this.expatriation = expatriation;
}
public DocumentProperties fatherName(String fatherName) {
this.fatherName = fatherName;
return this;
}
/**
* Get fatherName
* @return fatherName
*/
@javax.annotation.Nullable
public String getFatherName() {
return fatherName;
}
public void setFatherName(String fatherName) {
this.fatherName = fatherName;
}
public DocumentProperties motherName(String motherName) {
this.motherName = motherName;
return this;
}
/**
* Get motherName
* @return motherName
*/
@javax.annotation.Nullable
public String getMotherName() {
return motherName;
}
public void setMotherName(String motherName) {
this.motherName = motherName;
}
public DocumentProperties religion(String religion) {
this.religion = religion;
return this;
}
/**
* Get religion
* @return religion
*/
@javax.annotation.Nullable
public String getReligion() {
return religion;
}
public void setReligion(String religion) {
this.religion = religion;
}
public DocumentProperties typeOfPermit(String typeOfPermit) {
this.typeOfPermit = typeOfPermit;
return this;
}
/**
* Get typeOfPermit
* @return typeOfPermit
*/
@javax.annotation.Nullable
public String getTypeOfPermit() {
return typeOfPermit;
}
public void setTypeOfPermit(String typeOfPermit) {
this.typeOfPermit = typeOfPermit;
}
public DocumentProperties versionNumber(String versionNumber) {
this.versionNumber = versionNumber;
return this;
}
/**
* Get versionNumber
* @return versionNumber
*/
@javax.annotation.Nullable
public String getVersionNumber() {
return versionNumber;
}
public void setVersionNumber(String versionNumber) {
this.versionNumber = versionNumber;
}
public DocumentProperties documentSubtype(String documentSubtype) {
this.documentSubtype = documentSubtype;
return this;
}
/**
* Get documentSubtype
* @return documentSubtype
*/
@javax.annotation.Nullable
public String getDocumentSubtype() {
return documentSubtype;
}
public void setDocumentSubtype(String documentSubtype) {
this.documentSubtype = documentSubtype;
}
public DocumentProperties profession(String profession) {
this.profession = profession;
return this;
}
/**
* Get profession
* @return profession
*/
@javax.annotation.Nullable
public String getProfession() {
return profession;
}
public void setProfession(String profession) {
this.profession = profession;
}
public DocumentProperties securityDocumentNumber(String securityDocumentNumber) {
this.securityDocumentNumber = securityDocumentNumber;
return this;
}
/**
* Get securityDocumentNumber
* @return securityDocumentNumber
*/
@javax.annotation.Nullable
public String getSecurityDocumentNumber() {
return securityDocumentNumber;
}
public void setSecurityDocumentNumber(String securityDocumentNumber) {
this.securityDocumentNumber = securityDocumentNumber;
}
public DocumentProperties taxNumber(String taxNumber) {
this.taxNumber = taxNumber;
return this;
}
/**
* Get taxNumber
* @return taxNumber
*/
@javax.annotation.Nullable
public String getTaxNumber() {
return taxNumber;
}
public void setTaxNumber(String taxNumber) {
this.taxNumber = taxNumber;
}
public DocumentProperties nistIdentityEvidenceStrength(NistIdentityEvidenceStrengthEnum nistIdentityEvidenceStrength) {
this.nistIdentityEvidenceStrength = nistIdentityEvidenceStrength;
return this;
}
/**
* Get nistIdentityEvidenceStrength
* @return nistIdentityEvidenceStrength
*/
@javax.annotation.Nullable
public NistIdentityEvidenceStrengthEnum getNistIdentityEvidenceStrength() {
return nistIdentityEvidenceStrength;
}
public void setNistIdentityEvidenceStrength(NistIdentityEvidenceStrengthEnum nistIdentityEvidenceStrength) {
this.nistIdentityEvidenceStrength = nistIdentityEvidenceStrength;
}
public DocumentProperties hasIssuanceConfirmation(HasIssuanceConfirmationEnum hasIssuanceConfirmation) {
this.hasIssuanceConfirmation = hasIssuanceConfirmation;
return this;
}
/**
* Get hasIssuanceConfirmation
* @return hasIssuanceConfirmation
*/
@javax.annotation.Nullable
public HasIssuanceConfirmationEnum getHasIssuanceConfirmation() {
return hasIssuanceConfirmation;
}
public void setHasIssuanceConfirmation(HasIssuanceConfirmationEnum hasIssuanceConfirmation) {
this.hasIssuanceConfirmation = hasIssuanceConfirmation;
}
public DocumentProperties realIdCompliance(Boolean realIdCompliance) {
this.realIdCompliance = realIdCompliance;
return this;
}
/**
* Get realIdCompliance
* @return realIdCompliance
*/
@javax.annotation.Nullable
public Boolean getRealIdCompliance() {
return realIdCompliance;
}
public void setRealIdCompliance(Boolean realIdCompliance) {
this.realIdCompliance = realIdCompliance;
}
public DocumentProperties securityTier(SecurityTierEnum securityTier) {
this.securityTier = securityTier;
return this;
}
/**
* Get securityTier
* @return securityTier
*/
@javax.annotation.Nullable
public SecurityTierEnum getSecurityTier() {
return securityTier;
}
public void setSecurityTier(SecurityTierEnum securityTier) {
this.securityTier = securityTier;
}
public DocumentProperties addressLines(DocumentPropertiesAddressLines addressLines) {
this.addressLines = addressLines;
return this;
}
/**
* Get addressLines
* @return addressLines
*/
@javax.annotation.Nullable
public DocumentPropertiesAddressLines getAddressLines() {
return addressLines;
}
public void setAddressLines(DocumentPropertiesAddressLines addressLines) {
this.addressLines = addressLines;
}
public DocumentProperties barcode(List barcode) {
this.barcode = barcode;
return this;
}
public DocumentProperties addBarcodeItem(DocumentPropertiesBarcodeInner barcodeItem) {
if (this.barcode == null) {
this.barcode = new ArrayList<>();
}
this.barcode.add(barcodeItem);
return this;
}
/**
* Get barcode
* @return barcode
*/
@javax.annotation.Nullable
public List getBarcode() {
return barcode;
}
public void setBarcode(List barcode) {
this.barcode = barcode;
}
public DocumentProperties nfc(DocumentPropertiesNfc nfc) {
this.nfc = nfc;
return this;
}
/**
* Get nfc
* @return nfc
*/
@javax.annotation.Nullable
public DocumentPropertiesNfc getNfc() {
return nfc;
}
public void setNfc(DocumentPropertiesNfc nfc) {
this.nfc = nfc;
}
public DocumentProperties drivingLicenceInformation(DocumentPropertiesDrivingLicenceInformation drivingLicenceInformation) {
this.drivingLicenceInformation = drivingLicenceInformation;
return this;
}
/**
* Get drivingLicenceInformation
* @return drivingLicenceInformation
*/
@javax.annotation.Nullable
public DocumentPropertiesDrivingLicenceInformation getDrivingLicenceInformation() {
return drivingLicenceInformation;
}
public void setDrivingLicenceInformation(DocumentPropertiesDrivingLicenceInformation drivingLicenceInformation) {
this.drivingLicenceInformation = drivingLicenceInformation;
}
public DocumentProperties documentClassification(DocumentPropertiesDocumentClassification documentClassification) {
this.documentClassification = documentClassification;
return this;
}
/**
* Get documentClassification
* @return documentClassification
*/
@javax.annotation.Nullable
public DocumentPropertiesDocumentClassification getDocumentClassification() {
return documentClassification;
}
public void setDocumentClassification(DocumentPropertiesDocumentClassification documentClassification) {
this.documentClassification = documentClassification;
}
public DocumentProperties extractedData(DocumentPropertiesExtractedData extractedData) {
this.extractedData = extractedData;
return this;
}
/**
* Get extractedData
* @return extractedData
*/
@javax.annotation.Nullable
public DocumentPropertiesExtractedData getExtractedData() {
return extractedData;
}
public void setExtractedData(DocumentPropertiesExtractedData extractedData) {
this.extractedData = extractedData;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the DocumentProperties instance itself
*/
public DocumentProperties putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DocumentProperties documentProperties = (DocumentProperties) o;
return Objects.equals(this.dateOfBirth, documentProperties.dateOfBirth) &&
Objects.equals(this.dateOfExpiry, documentProperties.dateOfExpiry) &&
Objects.equals(this.personalNumber, documentProperties.personalNumber) &&
Objects.equals(this.documentNumbers, documentProperties.documentNumbers) &&
Objects.equals(this.documentType, documentProperties.documentType) &&
Objects.equals(this.firstName, documentProperties.firstName) &&
Objects.equals(this.middleName, documentProperties.middleName) &&
Objects.equals(this.lastName, documentProperties.lastName) &&
Objects.equals(this.gender, documentProperties.gender) &&
Objects.equals(this.issuingCountry, documentProperties.issuingCountry) &&
Objects.equals(this.nationality, documentProperties.nationality) &&
Objects.equals(this.issuingState, documentProperties.issuingState) &&
Objects.equals(this.issuingDate, documentProperties.issuingDate) &&
Objects.equals(this.categorisation, documentProperties.categorisation) &&
Objects.equals(this.mrzLine1, documentProperties.mrzLine1) &&
Objects.equals(this.mrzLine2, documentProperties.mrzLine2) &&
Objects.equals(this.mrzLine3, documentProperties.mrzLine3) &&
Objects.equals(this.address, documentProperties.address) &&
Objects.equals(this.placeOfBirth, documentProperties.placeOfBirth) &&
Objects.equals(this.spouseName, documentProperties.spouseName) &&
Objects.equals(this.widowName, documentProperties.widowName) &&
Objects.equals(this.aliasName, documentProperties.aliasName) &&
Objects.equals(this.issuingAuthority, documentProperties.issuingAuthority) &&
Objects.equals(this.remarks, documentProperties.remarks) &&
Objects.equals(this.civilState, documentProperties.civilState) &&
Objects.equals(this.expatriation, documentProperties.expatriation) &&
Objects.equals(this.fatherName, documentProperties.fatherName) &&
Objects.equals(this.motherName, documentProperties.motherName) &&
Objects.equals(this.religion, documentProperties.religion) &&
Objects.equals(this.typeOfPermit, documentProperties.typeOfPermit) &&
Objects.equals(this.versionNumber, documentProperties.versionNumber) &&
Objects.equals(this.documentSubtype, documentProperties.documentSubtype) &&
Objects.equals(this.profession, documentProperties.profession) &&
Objects.equals(this.securityDocumentNumber, documentProperties.securityDocumentNumber) &&
Objects.equals(this.taxNumber, documentProperties.taxNumber) &&
Objects.equals(this.nistIdentityEvidenceStrength, documentProperties.nistIdentityEvidenceStrength) &&
Objects.equals(this.hasIssuanceConfirmation, documentProperties.hasIssuanceConfirmation) &&
Objects.equals(this.realIdCompliance, documentProperties.realIdCompliance) &&
Objects.equals(this.securityTier, documentProperties.securityTier) &&
Objects.equals(this.addressLines, documentProperties.addressLines) &&
Objects.equals(this.barcode, documentProperties.barcode) &&
Objects.equals(this.nfc, documentProperties.nfc) &&
Objects.equals(this.drivingLicenceInformation, documentProperties.drivingLicenceInformation) &&
Objects.equals(this.documentClassification, documentProperties.documentClassification) &&
Objects.equals(this.extractedData, documentProperties.extractedData)&&
Objects.equals(this.additionalProperties, documentProperties.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(dateOfBirth, dateOfExpiry, personalNumber, documentNumbers, documentType, firstName, middleName, lastName, gender, issuingCountry, nationality, issuingState, issuingDate, categorisation, mrzLine1, mrzLine2, mrzLine3, address, placeOfBirth, spouseName, widowName, aliasName, issuingAuthority, remarks, civilState, expatriation, fatherName, motherName, religion, typeOfPermit, versionNumber, documentSubtype, profession, securityDocumentNumber, taxNumber, nistIdentityEvidenceStrength, hasIssuanceConfirmation, realIdCompliance, securityTier, addressLines, barcode, nfc, drivingLicenceInformation, documentClassification, extractedData, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DocumentProperties {\n");
sb.append(" dateOfBirth: ").append(toIndentedString(dateOfBirth)).append("\n");
sb.append(" dateOfExpiry: ").append(toIndentedString(dateOfExpiry)).append("\n");
sb.append(" personalNumber: ").append(toIndentedString(personalNumber)).append("\n");
sb.append(" documentNumbers: ").append(toIndentedString(documentNumbers)).append("\n");
sb.append(" documentType: ").append(toIndentedString(documentType)).append("\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" middleName: ").append(toIndentedString(middleName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" gender: ").append(toIndentedString(gender)).append("\n");
sb.append(" issuingCountry: ").append(toIndentedString(issuingCountry)).append("\n");
sb.append(" nationality: ").append(toIndentedString(nationality)).append("\n");
sb.append(" issuingState: ").append(toIndentedString(issuingState)).append("\n");
sb.append(" issuingDate: ").append(toIndentedString(issuingDate)).append("\n");
sb.append(" categorisation: ").append(toIndentedString(categorisation)).append("\n");
sb.append(" mrzLine1: ").append(toIndentedString(mrzLine1)).append("\n");
sb.append(" mrzLine2: ").append(toIndentedString(mrzLine2)).append("\n");
sb.append(" mrzLine3: ").append(toIndentedString(mrzLine3)).append("\n");
sb.append(" address: ").append(toIndentedString(address)).append("\n");
sb.append(" placeOfBirth: ").append(toIndentedString(placeOfBirth)).append("\n");
sb.append(" spouseName: ").append(toIndentedString(spouseName)).append("\n");
sb.append(" widowName: ").append(toIndentedString(widowName)).append("\n");
sb.append(" aliasName: ").append(toIndentedString(aliasName)).append("\n");
sb.append(" issuingAuthority: ").append(toIndentedString(issuingAuthority)).append("\n");
sb.append(" remarks: ").append(toIndentedString(remarks)).append("\n");
sb.append(" civilState: ").append(toIndentedString(civilState)).append("\n");
sb.append(" expatriation: ").append(toIndentedString(expatriation)).append("\n");
sb.append(" fatherName: ").append(toIndentedString(fatherName)).append("\n");
sb.append(" motherName: ").append(toIndentedString(motherName)).append("\n");
sb.append(" religion: ").append(toIndentedString(religion)).append("\n");
sb.append(" typeOfPermit: ").append(toIndentedString(typeOfPermit)).append("\n");
sb.append(" versionNumber: ").append(toIndentedString(versionNumber)).append("\n");
sb.append(" documentSubtype: ").append(toIndentedString(documentSubtype)).append("\n");
sb.append(" profession: ").append(toIndentedString(profession)).append("\n");
sb.append(" securityDocumentNumber: ").append(toIndentedString(securityDocumentNumber)).append("\n");
sb.append(" taxNumber: ").append(toIndentedString(taxNumber)).append("\n");
sb.append(" nistIdentityEvidenceStrength: ").append(toIndentedString(nistIdentityEvidenceStrength)).append("\n");
sb.append(" hasIssuanceConfirmation: ").append(toIndentedString(hasIssuanceConfirmation)).append("\n");
sb.append(" realIdCompliance: ").append(toIndentedString(realIdCompliance)).append("\n");
sb.append(" securityTier: ").append(toIndentedString(securityTier)).append("\n");
sb.append(" addressLines: ").append(toIndentedString(addressLines)).append("\n");
sb.append(" barcode: ").append(toIndentedString(barcode)).append("\n");
sb.append(" nfc: ").append(toIndentedString(nfc)).append("\n");
sb.append(" drivingLicenceInformation: ").append(toIndentedString(drivingLicenceInformation)).append("\n");
sb.append(" documentClassification: ").append(toIndentedString(documentClassification)).append("\n");
sb.append(" extractedData: ").append(toIndentedString(extractedData)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("date_of_birth");
openapiFields.add("date_of_expiry");
openapiFields.add("personal_number");
openapiFields.add("document_numbers");
openapiFields.add("document_type");
openapiFields.add("first_name");
openapiFields.add("middle_name");
openapiFields.add("last_name");
openapiFields.add("gender");
openapiFields.add("issuing_country");
openapiFields.add("nationality");
openapiFields.add("issuing_state");
openapiFields.add("issuing_date");
openapiFields.add("categorisation");
openapiFields.add("mrz_line1");
openapiFields.add("mrz_line2");
openapiFields.add("mrz_line3");
openapiFields.add("address");
openapiFields.add("place_of_birth");
openapiFields.add("spouse_name");
openapiFields.add("widow_name");
openapiFields.add("alias_name");
openapiFields.add("issuing_authority");
openapiFields.add("remarks");
openapiFields.add("civil_state");
openapiFields.add("expatriation");
openapiFields.add("father_name");
openapiFields.add("mother_name");
openapiFields.add("religion");
openapiFields.add("type_of_permit");
openapiFields.add("version_number");
openapiFields.add("document_subtype");
openapiFields.add("profession");
openapiFields.add("security_document_number");
openapiFields.add("tax_number");
openapiFields.add("nist_identity_evidence_strength");
openapiFields.add("has_issuance_confirmation");
openapiFields.add("real_id_compliance");
openapiFields.add("security_tier");
openapiFields.add("address_lines");
openapiFields.add("barcode");
openapiFields.add("nfc");
openapiFields.add("driving_licence_information");
openapiFields.add("document_classification");
openapiFields.add("extracted_data");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to DocumentProperties
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!DocumentProperties.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in DocumentProperties is not found in the empty JSON string", DocumentProperties.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("personal_number") != null && !jsonObj.get("personal_number").isJsonNull()) && !jsonObj.get("personal_number").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `personal_number` to be a primitive type in the JSON string but got `%s`", jsonObj.get("personal_number").toString()));
}
if (jsonObj.get("document_numbers") != null && !jsonObj.get("document_numbers").isJsonNull()) {
JsonArray jsonArraydocumentNumbers = jsonObj.getAsJsonArray("document_numbers");
if (jsonArraydocumentNumbers != null) {
// ensure the json data is an array
if (!jsonObj.get("document_numbers").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `document_numbers` to be an array in the JSON string but got `%s`", jsonObj.get("document_numbers").toString()));
}
// validate the optional field `document_numbers` (array)
for (int i = 0; i < jsonArraydocumentNumbers.size(); i++) {
DocumentPropertiesDocumentNumbersInner.validateJsonElement(jsonArraydocumentNumbers.get(i));
};
}
}
if ((jsonObj.get("document_type") != null && !jsonObj.get("document_type").isJsonNull()) && !jsonObj.get("document_type").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `document_type` to be a primitive type in the JSON string but got `%s`", jsonObj.get("document_type").toString()));
}
if ((jsonObj.get("first_name") != null && !jsonObj.get("first_name").isJsonNull()) && !jsonObj.get("first_name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `first_name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("first_name").toString()));
}
if ((jsonObj.get("middle_name") != null && !jsonObj.get("middle_name").isJsonNull()) && !jsonObj.get("middle_name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `middle_name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("middle_name").toString()));
}
if ((jsonObj.get("last_name") != null && !jsonObj.get("last_name").isJsonNull()) && !jsonObj.get("last_name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `last_name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("last_name").toString()));
}
if ((jsonObj.get("gender") != null && !jsonObj.get("gender").isJsonNull()) && !jsonObj.get("gender").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `gender` to be a primitive type in the JSON string but got `%s`", jsonObj.get("gender").toString()));
}
if ((jsonObj.get("issuing_country") != null && !jsonObj.get("issuing_country").isJsonNull()) && !jsonObj.get("issuing_country").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `issuing_country` to be a primitive type in the JSON string but got `%s`", jsonObj.get("issuing_country").toString()));
}
if ((jsonObj.get("nationality") != null && !jsonObj.get("nationality").isJsonNull()) && !jsonObj.get("nationality").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `nationality` to be a primitive type in the JSON string but got `%s`", jsonObj.get("nationality").toString()));
}
if ((jsonObj.get("issuing_state") != null && !jsonObj.get("issuing_state").isJsonNull()) && !jsonObj.get("issuing_state").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `issuing_state` to be a primitive type in the JSON string but got `%s`", jsonObj.get("issuing_state").toString()));
}
if ((jsonObj.get("categorisation") != null && !jsonObj.get("categorisation").isJsonNull()) && !jsonObj.get("categorisation").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `categorisation` to be a primitive type in the JSON string but got `%s`", jsonObj.get("categorisation").toString()));
}
if ((jsonObj.get("mrz_line1") != null && !jsonObj.get("mrz_line1").isJsonNull()) && !jsonObj.get("mrz_line1").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mrz_line1` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mrz_line1").toString()));
}
if ((jsonObj.get("mrz_line2") != null && !jsonObj.get("mrz_line2").isJsonNull()) && !jsonObj.get("mrz_line2").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mrz_line2` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mrz_line2").toString()));
}
if ((jsonObj.get("mrz_line3") != null && !jsonObj.get("mrz_line3").isJsonNull()) && !jsonObj.get("mrz_line3").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mrz_line3` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mrz_line3").toString()));
}
if ((jsonObj.get("address") != null && !jsonObj.get("address").isJsonNull()) && !jsonObj.get("address").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `address` to be a primitive type in the JSON string but got `%s`", jsonObj.get("address").toString()));
}
if ((jsonObj.get("place_of_birth") != null && !jsonObj.get("place_of_birth").isJsonNull()) && !jsonObj.get("place_of_birth").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `place_of_birth` to be a primitive type in the JSON string but got `%s`", jsonObj.get("place_of_birth").toString()));
}
if ((jsonObj.get("spouse_name") != null && !jsonObj.get("spouse_name").isJsonNull()) && !jsonObj.get("spouse_name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `spouse_name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("spouse_name").toString()));
}
if ((jsonObj.get("widow_name") != null && !jsonObj.get("widow_name").isJsonNull()) && !jsonObj.get("widow_name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `widow_name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("widow_name").toString()));
}
if ((jsonObj.get("alias_name") != null && !jsonObj.get("alias_name").isJsonNull()) && !jsonObj.get("alias_name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `alias_name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("alias_name").toString()));
}
if ((jsonObj.get("issuing_authority") != null && !jsonObj.get("issuing_authority").isJsonNull()) && !jsonObj.get("issuing_authority").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `issuing_authority` to be a primitive type in the JSON string but got `%s`", jsonObj.get("issuing_authority").toString()));
}
if ((jsonObj.get("remarks") != null && !jsonObj.get("remarks").isJsonNull()) && !jsonObj.get("remarks").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `remarks` to be a primitive type in the JSON string but got `%s`", jsonObj.get("remarks").toString()));
}
if ((jsonObj.get("civil_state") != null && !jsonObj.get("civil_state").isJsonNull()) && !jsonObj.get("civil_state").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `civil_state` to be a primitive type in the JSON string but got `%s`", jsonObj.get("civil_state").toString()));
}
if ((jsonObj.get("expatriation") != null && !jsonObj.get("expatriation").isJsonNull()) && !jsonObj.get("expatriation").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `expatriation` to be a primitive type in the JSON string but got `%s`", jsonObj.get("expatriation").toString()));
}
if ((jsonObj.get("father_name") != null && !jsonObj.get("father_name").isJsonNull()) && !jsonObj.get("father_name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `father_name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("father_name").toString()));
}
if ((jsonObj.get("mother_name") != null && !jsonObj.get("mother_name").isJsonNull()) && !jsonObj.get("mother_name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `mother_name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("mother_name").toString()));
}
if ((jsonObj.get("religion") != null && !jsonObj.get("religion").isJsonNull()) && !jsonObj.get("religion").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `religion` to be a primitive type in the JSON string but got `%s`", jsonObj.get("religion").toString()));
}
if ((jsonObj.get("type_of_permit") != null && !jsonObj.get("type_of_permit").isJsonNull()) && !jsonObj.get("type_of_permit").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `type_of_permit` to be a primitive type in the JSON string but got `%s`", jsonObj.get("type_of_permit").toString()));
}
if ((jsonObj.get("version_number") != null && !jsonObj.get("version_number").isJsonNull()) && !jsonObj.get("version_number").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `version_number` to be a primitive type in the JSON string but got `%s`", jsonObj.get("version_number").toString()));
}
if ((jsonObj.get("document_subtype") != null && !jsonObj.get("document_subtype").isJsonNull()) && !jsonObj.get("document_subtype").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `document_subtype` to be a primitive type in the JSON string but got `%s`", jsonObj.get("document_subtype").toString()));
}
if ((jsonObj.get("profession") != null && !jsonObj.get("profession").isJsonNull()) && !jsonObj.get("profession").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `profession` to be a primitive type in the JSON string but got `%s`", jsonObj.get("profession").toString()));
}
if ((jsonObj.get("security_document_number") != null && !jsonObj.get("security_document_number").isJsonNull()) && !jsonObj.get("security_document_number").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `security_document_number` to be a primitive type in the JSON string but got `%s`", jsonObj.get("security_document_number").toString()));
}
if ((jsonObj.get("tax_number") != null && !jsonObj.get("tax_number").isJsonNull()) && !jsonObj.get("tax_number").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `tax_number` to be a primitive type in the JSON string but got `%s`", jsonObj.get("tax_number").toString()));
}
if ((jsonObj.get("nist_identity_evidence_strength") != null && !jsonObj.get("nist_identity_evidence_strength").isJsonNull()) && !jsonObj.get("nist_identity_evidence_strength").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `nist_identity_evidence_strength` to be a primitive type in the JSON string but got `%s`", jsonObj.get("nist_identity_evidence_strength").toString()));
}
// validate the optional field `nist_identity_evidence_strength`
if (jsonObj.get("nist_identity_evidence_strength") != null && !jsonObj.get("nist_identity_evidence_strength").isJsonNull()) {
NistIdentityEvidenceStrengthEnum.validateJsonElement(jsonObj.get("nist_identity_evidence_strength"));
}
if ((jsonObj.get("has_issuance_confirmation") != null && !jsonObj.get("has_issuance_confirmation").isJsonNull()) && !jsonObj.get("has_issuance_confirmation").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `has_issuance_confirmation` to be a primitive type in the JSON string but got `%s`", jsonObj.get("has_issuance_confirmation").toString()));
}
// validate the optional field `has_issuance_confirmation`
if (jsonObj.get("has_issuance_confirmation") != null && !jsonObj.get("has_issuance_confirmation").isJsonNull()) {
HasIssuanceConfirmationEnum.validateJsonElement(jsonObj.get("has_issuance_confirmation"));
}
if ((jsonObj.get("security_tier") != null && !jsonObj.get("security_tier").isJsonNull()) && !jsonObj.get("security_tier").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `security_tier` to be a primitive type in the JSON string but got `%s`", jsonObj.get("security_tier").toString()));
}
// validate the optional field `security_tier`
if (jsonObj.get("security_tier") != null && !jsonObj.get("security_tier").isJsonNull()) {
SecurityTierEnum.validateJsonElement(jsonObj.get("security_tier"));
}
// validate the optional field `address_lines`
if (jsonObj.get("address_lines") != null && !jsonObj.get("address_lines").isJsonNull()) {
DocumentPropertiesAddressLines.validateJsonElement(jsonObj.get("address_lines"));
}
if (jsonObj.get("barcode") != null && !jsonObj.get("barcode").isJsonNull()) {
JsonArray jsonArraybarcode = jsonObj.getAsJsonArray("barcode");
if (jsonArraybarcode != null) {
// ensure the json data is an array
if (!jsonObj.get("barcode").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `barcode` to be an array in the JSON string but got `%s`", jsonObj.get("barcode").toString()));
}
// validate the optional field `barcode` (array)
for (int i = 0; i < jsonArraybarcode.size(); i++) {
DocumentPropertiesBarcodeInner.validateJsonElement(jsonArraybarcode.get(i));
};
}
}
// validate the optional field `nfc`
if (jsonObj.get("nfc") != null && !jsonObj.get("nfc").isJsonNull()) {
DocumentPropertiesNfc.validateJsonElement(jsonObj.get("nfc"));
}
// validate the optional field `driving_licence_information`
if (jsonObj.get("driving_licence_information") != null && !jsonObj.get("driving_licence_information").isJsonNull()) {
DocumentPropertiesDrivingLicenceInformation.validateJsonElement(jsonObj.get("driving_licence_information"));
}
// validate the optional field `document_classification`
if (jsonObj.get("document_classification") != null && !jsonObj.get("document_classification").isJsonNull()) {
DocumentPropertiesDocumentClassification.validateJsonElement(jsonObj.get("document_classification"));
}
// validate the optional field `extracted_data`
if (jsonObj.get("extracted_data") != null && !jsonObj.get("extracted_data").isJsonNull()) {
DocumentPropertiesExtractedData.validateJsonElement(jsonObj.get("extracted_data"));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!DocumentProperties.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'DocumentProperties' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(DocumentProperties.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, DocumentProperties value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// serialize additional properties
if (value.getAdditionalProperties() != null) {
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else if (jsonElement.isJsonObject()) {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
}
}
elementAdapter.write(out, obj);
}
@Override
public DocumentProperties read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
DocumentProperties instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of DocumentProperties given an JSON string
*
* @param jsonString JSON string
* @return An instance of DocumentProperties
* @throws IOException if the JSON string is invalid with respect to DocumentProperties
*/
public static DocumentProperties fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, DocumentProperties.class);
}
/**
* Convert an instance of DocumentProperties to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy