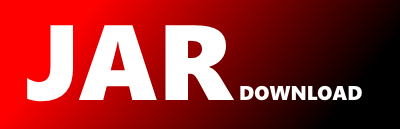
com.onthegomap.planetiler.util.Try Maven / Gradle / Ivy
package com.onthegomap.planetiler.util;
import static com.onthegomap.planetiler.util.Exceptions.throwFatalException;
/**
* A container for the result of an operation that may succeed or fail.
*
* @param Type of the result value, if success
*/
public interface Try {
/**
* Calls {@code supplier} and wraps the result in {@link Success} if successful, or {@link Failure} if it throws an
* exception.
*/
static Try apply(SupplierThatThrows supplier) {
try {
return success(supplier.get());
} catch (Exception e) {
return failure(e);
}
}
static Success success(T item) {
return new Success<>(item);
}
static Failure failure(Exception throwable) {
return new Failure<>(throwable);
}
/**
* Returns the result if success, or throws an exception if failure.
*
* @throws IllegalStateException wrapping the exception on failure
*/
T get();
default boolean isSuccess() {
return !isFailure();
}
default boolean isFailure() {
return exception() != null;
}
default Exception exception() {
return null;
}
/** If success, then tries to cast the result to {@code clazz}, turning into a failure if not possible. */
default Try cast(Class clazz) {
return map(clazz::cast);
}
/**
* If this is a success, then maps the value through {@code fn}, returning the new value in a {@link Success} if
* successful, or {@link Failure} if the mapping function threw an exception.
*/
Try map(FunctionThatThrows fn);
record Success (T get) implements Try {
@Override
public Try map(FunctionThatThrows fn) {
return Try.apply(() -> fn.apply(get));
}
}
record Failure (@Override Exception exception) implements Try {
@Override
public T get() {
return throwFatalException(exception);
}
@Override
@SuppressWarnings("unchecked")
public Try map(FunctionThatThrows fn) {
return (Try) this;
}
}
@FunctionalInterface
interface SupplierThatThrows {
@SuppressWarnings("java:S112")
T get() throws Exception;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy