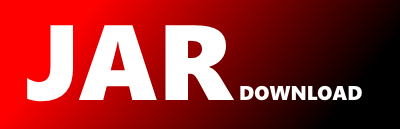
com.opencredo.concourse.domain.time.TimeRange Maven / Gradle / Ivy
package com.opencredo.concourse.domain.time;
import java.time.Instant;
import java.util.Optional;
public final class TimeRange {
private static final TimeRange UNBOUNDED = new TimeRange(Optional.empty(), Optional.empty());
public static TimeRange unbounded() {
return UNBOUNDED;
}
public interface UpperBoundCapture {
TimeRange to(Optional upper);
default TimeRange toInclusive(Instant upper) {
return to(Optional.of(TimeRangeBound.inclusive(upper)));
}
default TimeRange toExclusive(Instant upper) {
return to(Optional.of(TimeRangeBound.exclusive(upper)));
}
default TimeRange toUnbounded() {
return to(Optional.empty());
}
}
public static UpperBoundCapture from(Optional lower) {
return upper -> new TimeRange(lower, upper);
}
public static UpperBoundCapture fromInclusive(Instant lower) {
return from(Optional.of(TimeRangeBound.inclusive(lower)));
}
public static UpperBoundCapture fromExclusive(Instant upper) {
return from(Optional.of(TimeRangeBound.exclusive(upper)));
}
public static UpperBoundCapture fromUnbounded() {
return from(Optional.empty());
}
private final Optional lowerBound;
private final Optional upperBound;
private TimeRange(Optional lowerBound, Optional upperBound) {
this.lowerBound = lowerBound;
this.upperBound = upperBound;
}
public boolean contains(Instant instant) {
return lowerBound.map(lower -> lower.containsLower(instant)).orElse(true)
&& upperBound.map(upper -> upper.containsUpper(instant)).orElse(true);
}
public boolean isUnbounded() {
return !lowerBound.isPresent() && !upperBound.isPresent();
}
public Optional getLowerBound() {
return lowerBound;
}
public Optional getUpperBound() {
return upperBound;
}
@Override
public String toString() {
return "TimeRange from " + lowerBound.map(TimeRangeBound::toString).orElse("unbounded") + " to "
+ upperBound.map(TimeRangeBound::toString).orElse("unbounded");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy