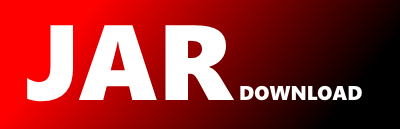
com.opengamma.strata.loader.csv.FxRatesCsvLoader Maven / Gradle / Ivy
Show all versions of strata-loader Show documentation
/*
* Copyright (C) 2015 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.loader.csv;
import static java.util.stream.Collectors.toList;
import java.time.LocalDate;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.function.Predicate;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableMap.Builder;
import com.google.common.io.CharSource;
import com.opengamma.strata.basics.currency.CurrencyPair;
import com.opengamma.strata.basics.currency.FxRate;
import com.opengamma.strata.collect.io.CharSources;
import com.opengamma.strata.collect.io.CsvFile;
import com.opengamma.strata.collect.io.CsvRow;
import com.opengamma.strata.collect.io.ResourceLocator;
import com.opengamma.strata.collect.io.UnicodeBom;
import com.opengamma.strata.collect.result.ParseFailureException;
import com.opengamma.strata.data.FxRateId;
import com.opengamma.strata.loader.LoaderUtils;
/**
* Loads a set of FX rates into memory from CSV resources.
*
* The rates are expected to be in a CSV format, with the following header row:
* {@code Valuation Date, Currency Pair, Value}.
*
* - The 'Valuation Date' column provides the valuation date, allowing data from different
* days to be stored in the same file
*
- The 'Currency Pair' column is the currency pair in the format 'EUR/USD'.
*
- The 'Value' column is the value of the rate.
*
*
* Each file may contain entries for many different dates.
*
* For example:
*
* Valuation Date, Currency Pair, Value
* 2014-01-22, EUR/USD, 1.10
* 2014-01-22, GBP/USD, 1.50
* 2014-01-23, EUR/USD, 1.11
*
* Note that Microsoft Excel prefers the CSV file to have no space after the comma.
*
* CSV files sometimes contain a Unicode Byte Order Mark.
* Callers are responsible for handling this, such as by using {@link UnicodeBom}.
*/
public final class FxRatesCsvLoader {
// CSV column headers
private static final String DATE_FIELD = "Valuation Date";
private static final String CURRENCY_PAIR_FIELD = "Currency Pair";
private static final String VALUE_FIELD = "Value";
//-------------------------------------------------------------------------
/**
* Loads one or more CSV format FX rate files for a specific date.
*
* Only those rates that match the specified date will be loaded.
*
* If the files contain a duplicate entry an exception will be thrown.
*
* @param marketDataDate the date to load
* @param resources the CSV resources
* @return the loaded FX rates, mapped by {@linkplain FxRateId rate ID}
* @throws IllegalArgumentException if the files contain a duplicate entry
*/
public static ImmutableMap load(LocalDate marketDataDate, ResourceLocator... resources) {
return load(marketDataDate, Arrays.asList(resources));
}
/**
* Loads one or more CSV format FX rate files for a specific date.
*
* Only those rates that match the specified date will be loaded.
*
* If the files contain a duplicate entry an exception will be thrown.
*
* @param marketDataDate the date to load
* @param resources the CSV resources
* @return the loaded FX rates, mapped by {@linkplain FxRateId rate ID}
* @throws IllegalArgumentException if the files contain a duplicate entry
*/
public static ImmutableMap load(LocalDate marketDataDate, Collection resources) {
Collection charSources = resources.stream().map(r -> r.getCharSource()).collect(toList());
return parse(d -> marketDataDate.equals(d), charSources).getOrDefault(marketDataDate, ImmutableMap.of());
}
//-------------------------------------------------------------------------
/**
* Loads one or more CSV format FX rate files for a set of dates.
*
* Only those rates that match one of the specified dates will be loaded.
*
* If the files contain a duplicate entry an exception will be thrown.
*
* @param marketDataDates the set of dates to load
* @param resources the CSV resources
* @return the loaded FX rates, mapped by {@link LocalDate} and {@linkplain FxRateId rate ID}
* @throws IllegalArgumentException if the files contain a duplicate entry
*/
public static ImmutableMap> load(
Set marketDataDates,
ResourceLocator... resources) {
return load(marketDataDates, Arrays.asList(resources));
}
/**
* Loads one or more CSV format FX rate files for a set of dates.
*
* Only those rates that match one of the specified dates will be loaded.
*
* If the files contain a duplicate entry an exception will be thrown.
*
* @param marketDataDates the dates to load
* @param resources the CSV resources
* @return the loaded FX rates, mapped by {@link LocalDate} and {@linkplain FxRateId rate ID}
* @throws IllegalArgumentException if the files contain a duplicate entry
*/
public static ImmutableMap> load(
Set marketDataDates,
Collection resources) {
Collection charSources = resources.stream().map(r -> r.getCharSource()).collect(toList());
return parse(d -> marketDataDates.contains(d), charSources);
}
//-------------------------------------------------------------------------
/**
* Loads one or more CSV format FX rate files.
*
* All dates that are found will be returned.
*
* If the files contain a duplicate entry an exception will be thrown.
*
* @param resources the CSV resources
* @return the loaded FX rates, mapped by {@link LocalDate} and {@linkplain FxRateId rate ID}
* @throws IllegalArgumentException if the files contain a duplicate entry
*/
public static ImmutableMap> loadAllDates(ResourceLocator... resources) {
return loadAllDates(Arrays.asList(resources));
}
/**
* Loads one or more CSV format FX rate files.
*
* All dates that are found will be returned.
*
* If the files contain a duplicate entry an exception will be thrown.
*
* @param resources the CSV resources
* @return the loaded FX rates, mapped by {@link LocalDate} and {@linkplain FxRateId rate ID}
* @throws IllegalArgumentException if the files contain a duplicate entry
*/
public static ImmutableMap> loadAllDates(
Collection resources) {
Collection charSources = resources.stream().map(r -> r.getCharSource()).collect(toList());
return parse(d -> true, charSources);
}
//-------------------------------------------------------------------------
/**
* Parses one or more CSV format FX rate files.
*
* A predicate is specified that is used to filter the dates that are returned.
* This could match a single date, a set of dates or all dates.
*
* If the files contain a duplicate entry an exception will be thrown.
*
* @param datePredicate the predicate used to select the dates
* @param charSources the CSV character sources
* @return the loaded FX rates, mapped by {@link LocalDate} and {@linkplain FxRateId rate ID}
* @throws IllegalArgumentException if the files contain a duplicate entry
*/
public static ImmutableMap> parse(
Predicate datePredicate,
Collection charSources) {
try {
// builder ensures keys can only be seen once
Map> mutableMap = new HashMap<>();
for (CharSource charSource : charSources) {
parseSingle(datePredicate, charSource, mutableMap);
}
ImmutableMap.Builder> builder = ImmutableMap.builder();
for (Entry> entry : mutableMap.entrySet()) {
builder.put(entry.getKey(), entry.getValue().build());
}
return builder.build();
} catch (ParseFailureException ex) {
throw ex;
} catch (RuntimeException ex) {
throw new ParseFailureException(ex, "Error parsing FX rates CSV files: {exceptionMessage}", ex.getMessage());
}
}
// loads a single CSV file, filtering by date
private static void parseSingle(
Predicate datePredicate,
CharSource resource,
Map> mutableMap) {
try {
CsvFile csv = CsvFile.of(resource, true);
for (CsvRow row : csv.rows()) {
String dateText = row.getField(DATE_FIELD);
LocalDate date = LoaderUtils.parseDate(dateText);
if (datePredicate.test(date)) {
String currencyPairStr = row.getField(CURRENCY_PAIR_FIELD);
String valueStr = row.getField(VALUE_FIELD);
CurrencyPair currencyPair = CurrencyPair.parse(currencyPairStr);
double value = Double.valueOf(valueStr);
ImmutableMap.Builder builderForDate = mutableMap.computeIfAbsent(date, k -> ImmutableMap.builder());
builderForDate.put(FxRateId.of(currencyPair), FxRate.of(currencyPair, value));
}
}
} catch (RuntimeException ex) {
throw new ParseFailureException(
ex, "Error parsing CSV file '{fileName}': {exceptionMessage}", CharSources.extractFileName(resource), ex.getMessage());
}
}
//-------------------------------------------------------------------------
/**
* Restricted constructor.
*/
private FxRatesCsvLoader() {
}
}