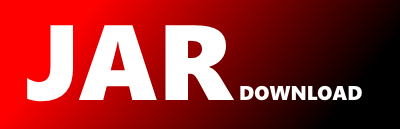
com.opengamma.strata.market.amount.LegAmounts Maven / Gradle / Ivy
Show all versions of strata-market Show documentation
/*
* Copyright (C) 2015 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.market.amount;
import static com.opengamma.strata.collect.Guavate.toImmutableList;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import org.joda.beans.Bean;
import org.joda.beans.BeanBuilder;
import org.joda.beans.ImmutableBean;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectMetaBean;
import org.joda.beans.impl.direct.DirectMetaProperty;
import org.joda.beans.impl.direct.DirectMetaPropertyMap;
import org.joda.beans.impl.direct.DirectPrivateBeanBuilder;
import com.google.common.collect.ImmutableList;
import com.opengamma.strata.basics.currency.Currency;
import com.opengamma.strata.basics.currency.FxConvertible;
import com.opengamma.strata.basics.currency.FxRateProvider;
/**
* A collection of leg amounts.
*
* Contains a list of individual {@linkplain LegAmount leg amount} objects, each representing an
* amount associated with one leg of an instrument. The order of the list is expected to be the
* same as the order in which the legs are defined on the instrument.
*/
@BeanDefinition(builderScope = "private")
public final class LegAmounts
implements FxConvertible, ImmutableBean, Serializable {
/**
* The leg amounts.
*/
@PropertyDefinition(validate = "notNull")
private final ImmutableList amounts;
//-------------------------------------------------------------------------
/**
* Returns an instance containing the specified leg amounts.
*
* @param amounts the individual leg amounts
* @return an instance containing the specified leg amounts
*/
public static LegAmounts of(List amounts) {
return new LegAmounts(amounts);
}
/**
* Returns an instance containing the specified leg amounts.
*
* @param amounts the individual leg amounts
* @return an instance containing the specified leg amounts
*/
public static LegAmounts of(LegAmount... amounts) {
return new LegAmounts(ImmutableList.copyOf(amounts));
}
@Override
public LegAmounts convertedTo(Currency resultCurrency, FxRateProvider rateProvider) {
List convertedAmounts = amounts.stream()
.map(amount -> amount.convertedTo(resultCurrency, rateProvider))
.collect(toImmutableList());
return of(convertedAmounts);
}
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code LegAmounts}.
* @return the meta-bean, not null
*/
public static LegAmounts.Meta meta() {
return LegAmounts.Meta.INSTANCE;
}
static {
MetaBean.register(LegAmounts.Meta.INSTANCE);
}
/**
* The serialization version id.
*/
private static final long serialVersionUID = 1L;
private LegAmounts(
List amounts) {
JodaBeanUtils.notNull(amounts, "amounts");
this.amounts = ImmutableList.copyOf(amounts);
}
@Override
public LegAmounts.Meta metaBean() {
return LegAmounts.Meta.INSTANCE;
}
//-----------------------------------------------------------------------
/**
* Gets the leg amounts.
* @return the value of the property, not null
*/
public ImmutableList getAmounts() {
return amounts;
}
//-----------------------------------------------------------------------
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
LegAmounts other = (LegAmounts) obj;
return JodaBeanUtils.equal(amounts, other.amounts);
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(amounts);
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(64);
buf.append("LegAmounts{");
buf.append("amounts").append('=').append(JodaBeanUtils.toString(amounts));
buf.append('}');
return buf.toString();
}
//-----------------------------------------------------------------------
/**
* The meta-bean for {@code LegAmounts}.
*/
public static final class Meta extends DirectMetaBean {
/**
* The singleton instance of the meta-bean.
*/
static final Meta INSTANCE = new Meta();
/**
* The meta-property for the {@code amounts} property.
*/
@SuppressWarnings({"unchecked", "rawtypes" })
private final MetaProperty> amounts = DirectMetaProperty.ofImmutable(
this, "amounts", LegAmounts.class, (Class) ImmutableList.class);
/**
* The meta-properties.
*/
private final Map> metaPropertyMap$ = new DirectMetaPropertyMap(
this, null,
"amounts");
/**
* Restricted constructor.
*/
private Meta() {
}
@Override
protected MetaProperty> metaPropertyGet(String propertyName) {
switch (propertyName.hashCode()) {
case -879772901: // amounts
return amounts;
}
return super.metaPropertyGet(propertyName);
}
@Override
public BeanBuilder extends LegAmounts> builder() {
return new LegAmounts.Builder();
}
@Override
public Class extends LegAmounts> beanType() {
return LegAmounts.class;
}
@Override
public Map> metaPropertyMap() {
return metaPropertyMap$;
}
//-----------------------------------------------------------------------
/**
* The meta-property for the {@code amounts} property.
* @return the meta-property, not null
*/
public MetaProperty> amounts() {
return amounts;
}
//-----------------------------------------------------------------------
@Override
protected Object propertyGet(Bean bean, String propertyName, boolean quiet) {
switch (propertyName.hashCode()) {
case -879772901: // amounts
return ((LegAmounts) bean).getAmounts();
}
return super.propertyGet(bean, propertyName, quiet);
}
@Override
protected void propertySet(Bean bean, String propertyName, Object newValue, boolean quiet) {
metaProperty(propertyName);
if (quiet) {
return;
}
throw new UnsupportedOperationException("Property cannot be written: " + propertyName);
}
}
//-----------------------------------------------------------------------
/**
* The bean-builder for {@code LegAmounts}.
*/
private static final class Builder extends DirectPrivateBeanBuilder {
private List amounts = ImmutableList.of();
/**
* Restricted constructor.
*/
private Builder() {
}
//-----------------------------------------------------------------------
@Override
public Object get(String propertyName) {
switch (propertyName.hashCode()) {
case -879772901: // amounts
return amounts;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
}
@SuppressWarnings("unchecked")
@Override
public Builder set(String propertyName, Object newValue) {
switch (propertyName.hashCode()) {
case -879772901: // amounts
this.amounts = (List) newValue;
break;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
return this;
}
@Override
public LegAmounts build() {
return new LegAmounts(
amounts);
}
//-----------------------------------------------------------------------
@Override
public String toString() {
StringBuilder buf = new StringBuilder(64);
buf.append("LegAmounts.Builder{");
buf.append("amounts").append('=').append(JodaBeanUtils.toString(amounts));
buf.append('}');
return buf.toString();
}
}
//-------------------------- AUTOGENERATED END --------------------------
}