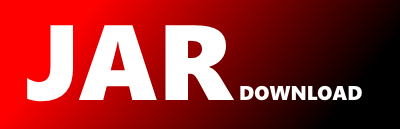
com.opengamma.strata.market.cube.SimpleCubeParameterMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of strata-market Show documentation
Show all versions of strata-market Show documentation
Entities describing the financial market
The newest version!
/*
* Copyright (C) 2024 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.market.cube;
import java.io.Serializable;
import java.util.Map;
import java.util.NoSuchElementException;
import org.joda.beans.Bean;
import org.joda.beans.BeanBuilder;
import org.joda.beans.ImmutableBean;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectMetaBean;
import org.joda.beans.impl.direct.DirectMetaProperty;
import org.joda.beans.impl.direct.DirectMetaPropertyMap;
import org.joda.beans.impl.direct.DirectPrivateBeanBuilder;
import com.opengamma.strata.market.ValueType;
import com.opengamma.strata.market.param.ParameterMetadata;
/**
* Simple parameter metadata containing the x, y, z values and type.
*/
@BeanDefinition(builderScope = "private")
public final class SimpleCubeParameterMetadata
implements ParameterMetadata, ImmutableBean, Serializable {
/**
* The type of the x-value.
*/
@PropertyDefinition(validate = "notNull")
private final ValueType xValueType;
/**
* The x-value.
*/
@PropertyDefinition
private final double xValue;
/**
* The type of the y-value.
*/
@PropertyDefinition(validate = "notNull")
private final ValueType yValueType;
/**
* The y-value.
*/
@PropertyDefinition
private final double yValue;
/**
* The type of the z-value.
*/
@PropertyDefinition(validate = "notNull")
private final ValueType zValueType;
/**
* The z-value.
*/
@PropertyDefinition
private final double zValue;
//-------------------------------------------------------------------------
/**
* Obtains an instance specifying information.
*
* @param xValueType the x-value type
* @param xValue the x-value
* @param yValueType the x-value type
* @param yValue the x-value
* @param zValueType the z-value type
* @param zValue the z-value
* @return the parameter metadata based on the date and label
*/
public static SimpleCubeParameterMetadata of(
ValueType xValueType,
double xValue,
ValueType yValueType,
double yValue,
ValueType zValueType,
double zValue) {
return new SimpleCubeParameterMetadata(xValueType, xValue, yValueType, yValue, zValueType, zValue);
}
//-------------------------------------------------------------------------
@Override
public String getLabel() {
return xValueType + "=" + xValue + ", " + yValueType + "=" + yValue + ", " + zValueType + "=" + zValue;
}
@Override
public String getIdentifier() {
return getLabel();
}
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code SimpleCubeParameterMetadata}.
* @return the meta-bean, not null
*/
public static SimpleCubeParameterMetadata.Meta meta() {
return SimpleCubeParameterMetadata.Meta.INSTANCE;
}
static {
MetaBean.register(SimpleCubeParameterMetadata.Meta.INSTANCE);
}
/**
* The serialization version id.
*/
private static final long serialVersionUID = 1L;
private SimpleCubeParameterMetadata(
ValueType xValueType,
double xValue,
ValueType yValueType,
double yValue,
ValueType zValueType,
double zValue) {
JodaBeanUtils.notNull(xValueType, "xValueType");
JodaBeanUtils.notNull(yValueType, "yValueType");
JodaBeanUtils.notNull(zValueType, "zValueType");
this.xValueType = xValueType;
this.xValue = xValue;
this.yValueType = yValueType;
this.yValue = yValue;
this.zValueType = zValueType;
this.zValue = zValue;
}
@Override
public SimpleCubeParameterMetadata.Meta metaBean() {
return SimpleCubeParameterMetadata.Meta.INSTANCE;
}
//-----------------------------------------------------------------------
/**
* Gets the type of the x-value.
* @return the value of the property, not null
*/
public ValueType getXValueType() {
return xValueType;
}
//-----------------------------------------------------------------------
/**
* Gets the x-value.
* @return the value of the property
*/
public double getXValue() {
return xValue;
}
//-----------------------------------------------------------------------
/**
* Gets the type of the y-value.
* @return the value of the property, not null
*/
public ValueType getYValueType() {
return yValueType;
}
//-----------------------------------------------------------------------
/**
* Gets the y-value.
* @return the value of the property
*/
public double getYValue() {
return yValue;
}
//-----------------------------------------------------------------------
/**
* Gets the type of the z-value.
* @return the value of the property, not null
*/
public ValueType getZValueType() {
return zValueType;
}
//-----------------------------------------------------------------------
/**
* Gets the z-value.
* @return the value of the property
*/
public double getZValue() {
return zValue;
}
//-----------------------------------------------------------------------
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
SimpleCubeParameterMetadata other = (SimpleCubeParameterMetadata) obj;
return JodaBeanUtils.equal(xValueType, other.xValueType) &&
JodaBeanUtils.equal(xValue, other.xValue) &&
JodaBeanUtils.equal(yValueType, other.yValueType) &&
JodaBeanUtils.equal(yValue, other.yValue) &&
JodaBeanUtils.equal(zValueType, other.zValueType) &&
JodaBeanUtils.equal(zValue, other.zValue);
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(xValueType);
hash = hash * 31 + JodaBeanUtils.hashCode(xValue);
hash = hash * 31 + JodaBeanUtils.hashCode(yValueType);
hash = hash * 31 + JodaBeanUtils.hashCode(yValue);
hash = hash * 31 + JodaBeanUtils.hashCode(zValueType);
hash = hash * 31 + JodaBeanUtils.hashCode(zValue);
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(224);
buf.append("SimpleCubeParameterMetadata{");
buf.append("xValueType").append('=').append(JodaBeanUtils.toString(xValueType)).append(',').append(' ');
buf.append("xValue").append('=').append(JodaBeanUtils.toString(xValue)).append(',').append(' ');
buf.append("yValueType").append('=').append(JodaBeanUtils.toString(yValueType)).append(',').append(' ');
buf.append("yValue").append('=').append(JodaBeanUtils.toString(yValue)).append(',').append(' ');
buf.append("zValueType").append('=').append(JodaBeanUtils.toString(zValueType)).append(',').append(' ');
buf.append("zValue").append('=').append(JodaBeanUtils.toString(zValue));
buf.append('}');
return buf.toString();
}
//-----------------------------------------------------------------------
/**
* The meta-bean for {@code SimpleCubeParameterMetadata}.
*/
public static final class Meta extends DirectMetaBean {
/**
* The singleton instance of the meta-bean.
*/
static final Meta INSTANCE = new Meta();
/**
* The meta-property for the {@code xValueType} property.
*/
private final MetaProperty xValueType = DirectMetaProperty.ofImmutable(
this, "xValueType", SimpleCubeParameterMetadata.class, ValueType.class);
/**
* The meta-property for the {@code xValue} property.
*/
private final MetaProperty xValue = DirectMetaProperty.ofImmutable(
this, "xValue", SimpleCubeParameterMetadata.class, Double.TYPE);
/**
* The meta-property for the {@code yValueType} property.
*/
private final MetaProperty yValueType = DirectMetaProperty.ofImmutable(
this, "yValueType", SimpleCubeParameterMetadata.class, ValueType.class);
/**
* The meta-property for the {@code yValue} property.
*/
private final MetaProperty yValue = DirectMetaProperty.ofImmutable(
this, "yValue", SimpleCubeParameterMetadata.class, Double.TYPE);
/**
* The meta-property for the {@code zValueType} property.
*/
private final MetaProperty zValueType = DirectMetaProperty.ofImmutable(
this, "zValueType", SimpleCubeParameterMetadata.class, ValueType.class);
/**
* The meta-property for the {@code zValue} property.
*/
private final MetaProperty zValue = DirectMetaProperty.ofImmutable(
this, "zValue", SimpleCubeParameterMetadata.class, Double.TYPE);
/**
* The meta-properties.
*/
private final Map> metaPropertyMap$ = new DirectMetaPropertyMap(
this, null,
"xValueType",
"xValue",
"yValueType",
"yValue",
"zValueType",
"zValue");
/**
* Restricted constructor.
*/
private Meta() {
}
@Override
protected MetaProperty> metaPropertyGet(String propertyName) {
switch (propertyName.hashCode()) {
case -868509005: // xValueType
return xValueType;
case -777049127: // xValue
return xValue;
case -1065022510: // yValueType
return yValueType;
case -748419976: // yValue
return yValue;
case -1261536015: // zValueType
return zValueType;
case -719790825: // zValue
return zValue;
}
return super.metaPropertyGet(propertyName);
}
@Override
public BeanBuilder extends SimpleCubeParameterMetadata> builder() {
return new SimpleCubeParameterMetadata.Builder();
}
@Override
public Class extends SimpleCubeParameterMetadata> beanType() {
return SimpleCubeParameterMetadata.class;
}
@Override
public Map> metaPropertyMap() {
return metaPropertyMap$;
}
//-----------------------------------------------------------------------
/**
* The meta-property for the {@code xValueType} property.
* @return the meta-property, not null
*/
public MetaProperty xValueType() {
return xValueType;
}
/**
* The meta-property for the {@code xValue} property.
* @return the meta-property, not null
*/
public MetaProperty xValue() {
return xValue;
}
/**
* The meta-property for the {@code yValueType} property.
* @return the meta-property, not null
*/
public MetaProperty yValueType() {
return yValueType;
}
/**
* The meta-property for the {@code yValue} property.
* @return the meta-property, not null
*/
public MetaProperty yValue() {
return yValue;
}
/**
* The meta-property for the {@code zValueType} property.
* @return the meta-property, not null
*/
public MetaProperty zValueType() {
return zValueType;
}
/**
* The meta-property for the {@code zValue} property.
* @return the meta-property, not null
*/
public MetaProperty zValue() {
return zValue;
}
//-----------------------------------------------------------------------
@Override
protected Object propertyGet(Bean bean, String propertyName, boolean quiet) {
switch (propertyName.hashCode()) {
case -868509005: // xValueType
return ((SimpleCubeParameterMetadata) bean).getXValueType();
case -777049127: // xValue
return ((SimpleCubeParameterMetadata) bean).getXValue();
case -1065022510: // yValueType
return ((SimpleCubeParameterMetadata) bean).getYValueType();
case -748419976: // yValue
return ((SimpleCubeParameterMetadata) bean).getYValue();
case -1261536015: // zValueType
return ((SimpleCubeParameterMetadata) bean).getZValueType();
case -719790825: // zValue
return ((SimpleCubeParameterMetadata) bean).getZValue();
}
return super.propertyGet(bean, propertyName, quiet);
}
@Override
protected void propertySet(Bean bean, String propertyName, Object newValue, boolean quiet) {
metaProperty(propertyName);
if (quiet) {
return;
}
throw new UnsupportedOperationException("Property cannot be written: " + propertyName);
}
}
//-----------------------------------------------------------------------
/**
* The bean-builder for {@code SimpleCubeParameterMetadata}.
*/
private static final class Builder extends DirectPrivateBeanBuilder {
private ValueType xValueType;
private double xValue;
private ValueType yValueType;
private double yValue;
private ValueType zValueType;
private double zValue;
/**
* Restricted constructor.
*/
private Builder() {
}
//-----------------------------------------------------------------------
@Override
public Object get(String propertyName) {
switch (propertyName.hashCode()) {
case -868509005: // xValueType
return xValueType;
case -777049127: // xValue
return xValue;
case -1065022510: // yValueType
return yValueType;
case -748419976: // yValue
return yValue;
case -1261536015: // zValueType
return zValueType;
case -719790825: // zValue
return zValue;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
}
@Override
public Builder set(String propertyName, Object newValue) {
switch (propertyName.hashCode()) {
case -868509005: // xValueType
this.xValueType = (ValueType) newValue;
break;
case -777049127: // xValue
this.xValue = (Double) newValue;
break;
case -1065022510: // yValueType
this.yValueType = (ValueType) newValue;
break;
case -748419976: // yValue
this.yValue = (Double) newValue;
break;
case -1261536015: // zValueType
this.zValueType = (ValueType) newValue;
break;
case -719790825: // zValue
this.zValue = (Double) newValue;
break;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
return this;
}
@Override
public SimpleCubeParameterMetadata build() {
return new SimpleCubeParameterMetadata(
xValueType,
xValue,
yValueType,
yValue,
zValueType,
zValue);
}
//-----------------------------------------------------------------------
@Override
public String toString() {
StringBuilder buf = new StringBuilder(224);
buf.append("SimpleCubeParameterMetadata.Builder{");
buf.append("xValueType").append('=').append(JodaBeanUtils.toString(xValueType)).append(',').append(' ');
buf.append("xValue").append('=').append(JodaBeanUtils.toString(xValue)).append(',').append(' ');
buf.append("yValueType").append('=').append(JodaBeanUtils.toString(yValueType)).append(',').append(' ');
buf.append("yValue").append('=').append(JodaBeanUtils.toString(yValue)).append(',').append(' ');
buf.append("zValueType").append('=').append(JodaBeanUtils.toString(zValueType)).append(',').append(' ');
buf.append("zValue").append('=').append(JodaBeanUtils.toString(zValue));
buf.append('}');
return buf.toString();
}
}
//-------------------------- AUTOGENERATED END --------------------------
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy