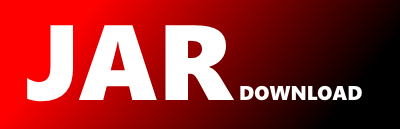
com.opengamma.strata.market.curve.DepositIsdaCreditCurveNode Maven / Gradle / Ivy
Show all versions of strata-market Show documentation
/*
* Copyright (C) 2016 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.market.curve;
import java.io.Serializable;
import java.time.LocalDate;
import java.util.Map;
import java.util.NoSuchElementException;
import org.joda.beans.Bean;
import org.joda.beans.ImmutableBean;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.ImmutablePreBuild;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectFieldsBeanBuilder;
import org.joda.beans.impl.direct.DirectMetaBean;
import org.joda.beans.impl.direct.DirectMetaProperty;
import org.joda.beans.impl.direct.DirectMetaPropertyMap;
import com.opengamma.strata.basics.ReferenceData;
import com.opengamma.strata.basics.date.BusinessDayAdjustment;
import com.opengamma.strata.basics.date.DayCount;
import com.opengamma.strata.basics.date.DaysAdjustment;
import com.opengamma.strata.basics.date.Tenor;
import com.opengamma.strata.data.ObservableId;
import com.opengamma.strata.market.param.TenorDateParameterMetadata;
/**
* An ISDA compliant curve node whose instrument is a term deposit.
*
* A term deposit is a financial instrument that provides a fixed rate of interest on an amount for a specific term.
* {@code observableId} is used to access the market data value of this fixed rate.
*/
@BeanDefinition
public final class DepositIsdaCreditCurveNode
implements IsdaCreditCurveNode, ImmutableBean, Serializable {
/**
* The label to use for the node, defaulted.
*
* When building, this will default based on the tenor if not specified.
*/
@PropertyDefinition(validate = "notEmpty", overrideGet = true)
private final String label;
/**
* The identifier of the market data value that provides the rate.
*/
@PropertyDefinition(validate = "notNull", overrideGet = true)
private final ObservableId observableId;
/**
* The period between the start date and the end date.
*/
@PropertyDefinition(validate = "notNull")
private final Tenor tenor;
/**
* The offset of the start date from the trade date.
*
* The offset is applied to the trade date and is typically plus 2 business days.
*/
@PropertyDefinition(validate = "notNull")
private final DaysAdjustment spotDateOffset;
/**
* The business day adjustment to apply to the start and end date.
*
* The start and end date will be adjusted as defined here.
*/
@PropertyDefinition(validate = "notNull")
private final BusinessDayAdjustment businessDayAdjustment;
/**
* The day count convention.
*
* This defines the term year fraction.
*/
@PropertyDefinition(validate = "notNull")
private final DayCount dayCount;
//-------------------------------------------------------------------------
/**
* Returns a curve node for a term deposit.
*
* The label will be created using {@code tenor}.
*
* @param observableId the observable ID
* @param spotDateOffset the spot date offset
* @param businessDayAdjustment the business day adjustment
* @param tenor the tenor
* @param dayCount the day count
* @return the curve node
*/
public static DepositIsdaCreditCurveNode of(
ObservableId observableId,
DaysAdjustment spotDateOffset,
BusinessDayAdjustment businessDayAdjustment,
Tenor tenor,
DayCount dayCount) {
return DepositIsdaCreditCurveNode.builder()
.observableId(observableId)
.tenor(tenor)
.businessDayAdjustment(businessDayAdjustment)
.dayCount(dayCount)
.spotDateOffset(spotDateOffset)
.build();
}
@ImmutablePreBuild
private static void preBuild(Builder builder) {
if (builder.label == null && builder.tenor != null) {
builder.label = builder.tenor.toString();
}
}
//-------------------------------------------------------------------------
@Override
public LocalDate date(LocalDate tradeDate, ReferenceData refData) {
LocalDate startDate = spotDateOffset.adjust(tradeDate, refData);
LocalDate endDate = startDate.plus(tenor);
return businessDayAdjustment.adjust(endDate, refData);
}
@Override
public TenorDateParameterMetadata metadata(LocalDate nodeDate) {
return TenorDateParameterMetadata.of(nodeDate, tenor);
}
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code DepositIsdaCreditCurveNode}.
* @return the meta-bean, not null
*/
public static DepositIsdaCreditCurveNode.Meta meta() {
return DepositIsdaCreditCurveNode.Meta.INSTANCE;
}
static {
MetaBean.register(DepositIsdaCreditCurveNode.Meta.INSTANCE);
}
/**
* The serialization version id.
*/
private static final long serialVersionUID = 1L;
/**
* Returns a builder used to create an instance of the bean.
* @return the builder, not null
*/
public static DepositIsdaCreditCurveNode.Builder builder() {
return new DepositIsdaCreditCurveNode.Builder();
}
private DepositIsdaCreditCurveNode(
String label,
ObservableId observableId,
Tenor tenor,
DaysAdjustment spotDateOffset,
BusinessDayAdjustment businessDayAdjustment,
DayCount dayCount) {
JodaBeanUtils.notEmpty(label, "label");
JodaBeanUtils.notNull(observableId, "observableId");
JodaBeanUtils.notNull(tenor, "tenor");
JodaBeanUtils.notNull(spotDateOffset, "spotDateOffset");
JodaBeanUtils.notNull(businessDayAdjustment, "businessDayAdjustment");
JodaBeanUtils.notNull(dayCount, "dayCount");
this.label = label;
this.observableId = observableId;
this.tenor = tenor;
this.spotDateOffset = spotDateOffset;
this.businessDayAdjustment = businessDayAdjustment;
this.dayCount = dayCount;
}
@Override
public DepositIsdaCreditCurveNode.Meta metaBean() {
return DepositIsdaCreditCurveNode.Meta.INSTANCE;
}
//-----------------------------------------------------------------------
/**
* Gets the label to use for the node, defaulted.
*
* When building, this will default based on the tenor if not specified.
* @return the value of the property, not empty
*/
@Override
public String getLabel() {
return label;
}
//-----------------------------------------------------------------------
/**
* Gets the identifier of the market data value that provides the rate.
* @return the value of the property, not null
*/
@Override
public ObservableId getObservableId() {
return observableId;
}
//-----------------------------------------------------------------------
/**
* Gets the period between the start date and the end date.
* @return the value of the property, not null
*/
public Tenor getTenor() {
return tenor;
}
//-----------------------------------------------------------------------
/**
* Gets the offset of the start date from the trade date.
*
* The offset is applied to the trade date and is typically plus 2 business days.
* @return the value of the property, not null
*/
public DaysAdjustment getSpotDateOffset() {
return spotDateOffset;
}
//-----------------------------------------------------------------------
/**
* Gets the business day adjustment to apply to the start and end date.
*
* The start and end date will be adjusted as defined here.
* @return the value of the property, not null
*/
public BusinessDayAdjustment getBusinessDayAdjustment() {
return businessDayAdjustment;
}
//-----------------------------------------------------------------------
/**
* Gets the day count convention.
*
* This defines the term year fraction.
* @return the value of the property, not null
*/
public DayCount getDayCount() {
return dayCount;
}
//-----------------------------------------------------------------------
/**
* Returns a builder that allows this bean to be mutated.
* @return the mutable builder, not null
*/
public Builder toBuilder() {
return new Builder(this);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
DepositIsdaCreditCurveNode other = (DepositIsdaCreditCurveNode) obj;
return JodaBeanUtils.equal(label, other.label) &&
JodaBeanUtils.equal(observableId, other.observableId) &&
JodaBeanUtils.equal(tenor, other.tenor) &&
JodaBeanUtils.equal(spotDateOffset, other.spotDateOffset) &&
JodaBeanUtils.equal(businessDayAdjustment, other.businessDayAdjustment) &&
JodaBeanUtils.equal(dayCount, other.dayCount);
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(label);
hash = hash * 31 + JodaBeanUtils.hashCode(observableId);
hash = hash * 31 + JodaBeanUtils.hashCode(tenor);
hash = hash * 31 + JodaBeanUtils.hashCode(spotDateOffset);
hash = hash * 31 + JodaBeanUtils.hashCode(businessDayAdjustment);
hash = hash * 31 + JodaBeanUtils.hashCode(dayCount);
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(224);
buf.append("DepositIsdaCreditCurveNode{");
buf.append("label").append('=').append(JodaBeanUtils.toString(label)).append(',').append(' ');
buf.append("observableId").append('=').append(JodaBeanUtils.toString(observableId)).append(',').append(' ');
buf.append("tenor").append('=').append(JodaBeanUtils.toString(tenor)).append(',').append(' ');
buf.append("spotDateOffset").append('=').append(JodaBeanUtils.toString(spotDateOffset)).append(',').append(' ');
buf.append("businessDayAdjustment").append('=').append(JodaBeanUtils.toString(businessDayAdjustment)).append(',').append(' ');
buf.append("dayCount").append('=').append(JodaBeanUtils.toString(dayCount));
buf.append('}');
return buf.toString();
}
//-----------------------------------------------------------------------
/**
* The meta-bean for {@code DepositIsdaCreditCurveNode}.
*/
public static final class Meta extends DirectMetaBean {
/**
* The singleton instance of the meta-bean.
*/
static final Meta INSTANCE = new Meta();
/**
* The meta-property for the {@code label} property.
*/
private final MetaProperty label = DirectMetaProperty.ofImmutable(
this, "label", DepositIsdaCreditCurveNode.class, String.class);
/**
* The meta-property for the {@code observableId} property.
*/
private final MetaProperty observableId = DirectMetaProperty.ofImmutable(
this, "observableId", DepositIsdaCreditCurveNode.class, ObservableId.class);
/**
* The meta-property for the {@code tenor} property.
*/
private final MetaProperty tenor = DirectMetaProperty.ofImmutable(
this, "tenor", DepositIsdaCreditCurveNode.class, Tenor.class);
/**
* The meta-property for the {@code spotDateOffset} property.
*/
private final MetaProperty spotDateOffset = DirectMetaProperty.ofImmutable(
this, "spotDateOffset", DepositIsdaCreditCurveNode.class, DaysAdjustment.class);
/**
* The meta-property for the {@code businessDayAdjustment} property.
*/
private final MetaProperty businessDayAdjustment = DirectMetaProperty.ofImmutable(
this, "businessDayAdjustment", DepositIsdaCreditCurveNode.class, BusinessDayAdjustment.class);
/**
* The meta-property for the {@code dayCount} property.
*/
private final MetaProperty dayCount = DirectMetaProperty.ofImmutable(
this, "dayCount", DepositIsdaCreditCurveNode.class, DayCount.class);
/**
* The meta-properties.
*/
private final Map> metaPropertyMap$ = new DirectMetaPropertyMap(
this, null,
"label",
"observableId",
"tenor",
"spotDateOffset",
"businessDayAdjustment",
"dayCount");
/**
* Restricted constructor.
*/
private Meta() {
}
@Override
protected MetaProperty> metaPropertyGet(String propertyName) {
switch (propertyName.hashCode()) {
case 102727412: // label
return label;
case -518800962: // observableId
return observableId;
case 110246592: // tenor
return tenor;
case 746995843: // spotDateOffset
return spotDateOffset;
case -1065319863: // businessDayAdjustment
return businessDayAdjustment;
case 1905311443: // dayCount
return dayCount;
}
return super.metaPropertyGet(propertyName);
}
@Override
public DepositIsdaCreditCurveNode.Builder builder() {
return new DepositIsdaCreditCurveNode.Builder();
}
@Override
public Class extends DepositIsdaCreditCurveNode> beanType() {
return DepositIsdaCreditCurveNode.class;
}
@Override
public Map> metaPropertyMap() {
return metaPropertyMap$;
}
//-----------------------------------------------------------------------
/**
* The meta-property for the {@code label} property.
* @return the meta-property, not null
*/
public MetaProperty label() {
return label;
}
/**
* The meta-property for the {@code observableId} property.
* @return the meta-property, not null
*/
public MetaProperty observableId() {
return observableId;
}
/**
* The meta-property for the {@code tenor} property.
* @return the meta-property, not null
*/
public MetaProperty tenor() {
return tenor;
}
/**
* The meta-property for the {@code spotDateOffset} property.
* @return the meta-property, not null
*/
public MetaProperty spotDateOffset() {
return spotDateOffset;
}
/**
* The meta-property for the {@code businessDayAdjustment} property.
* @return the meta-property, not null
*/
public MetaProperty businessDayAdjustment() {
return businessDayAdjustment;
}
/**
* The meta-property for the {@code dayCount} property.
* @return the meta-property, not null
*/
public MetaProperty dayCount() {
return dayCount;
}
//-----------------------------------------------------------------------
@Override
protected Object propertyGet(Bean bean, String propertyName, boolean quiet) {
switch (propertyName.hashCode()) {
case 102727412: // label
return ((DepositIsdaCreditCurveNode) bean).getLabel();
case -518800962: // observableId
return ((DepositIsdaCreditCurveNode) bean).getObservableId();
case 110246592: // tenor
return ((DepositIsdaCreditCurveNode) bean).getTenor();
case 746995843: // spotDateOffset
return ((DepositIsdaCreditCurveNode) bean).getSpotDateOffset();
case -1065319863: // businessDayAdjustment
return ((DepositIsdaCreditCurveNode) bean).getBusinessDayAdjustment();
case 1905311443: // dayCount
return ((DepositIsdaCreditCurveNode) bean).getDayCount();
}
return super.propertyGet(bean, propertyName, quiet);
}
@Override
protected void propertySet(Bean bean, String propertyName, Object newValue, boolean quiet) {
metaProperty(propertyName);
if (quiet) {
return;
}
throw new UnsupportedOperationException("Property cannot be written: " + propertyName);
}
}
//-----------------------------------------------------------------------
/**
* The bean-builder for {@code DepositIsdaCreditCurveNode}.
*/
public static final class Builder extends DirectFieldsBeanBuilder {
private String label;
private ObservableId observableId;
private Tenor tenor;
private DaysAdjustment spotDateOffset;
private BusinessDayAdjustment businessDayAdjustment;
private DayCount dayCount;
/**
* Restricted constructor.
*/
private Builder() {
}
/**
* Restricted copy constructor.
* @param beanToCopy the bean to copy from, not null
*/
private Builder(DepositIsdaCreditCurveNode beanToCopy) {
this.label = beanToCopy.getLabel();
this.observableId = beanToCopy.getObservableId();
this.tenor = beanToCopy.getTenor();
this.spotDateOffset = beanToCopy.getSpotDateOffset();
this.businessDayAdjustment = beanToCopy.getBusinessDayAdjustment();
this.dayCount = beanToCopy.getDayCount();
}
//-----------------------------------------------------------------------
@Override
public Object get(String propertyName) {
switch (propertyName.hashCode()) {
case 102727412: // label
return label;
case -518800962: // observableId
return observableId;
case 110246592: // tenor
return tenor;
case 746995843: // spotDateOffset
return spotDateOffset;
case -1065319863: // businessDayAdjustment
return businessDayAdjustment;
case 1905311443: // dayCount
return dayCount;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
}
@Override
public Builder set(String propertyName, Object newValue) {
switch (propertyName.hashCode()) {
case 102727412: // label
this.label = (String) newValue;
break;
case -518800962: // observableId
this.observableId = (ObservableId) newValue;
break;
case 110246592: // tenor
this.tenor = (Tenor) newValue;
break;
case 746995843: // spotDateOffset
this.spotDateOffset = (DaysAdjustment) newValue;
break;
case -1065319863: // businessDayAdjustment
this.businessDayAdjustment = (BusinessDayAdjustment) newValue;
break;
case 1905311443: // dayCount
this.dayCount = (DayCount) newValue;
break;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
return this;
}
@Override
public Builder set(MetaProperty> property, Object value) {
super.set(property, value);
return this;
}
@Override
public DepositIsdaCreditCurveNode build() {
preBuild(this);
return new DepositIsdaCreditCurveNode(
label,
observableId,
tenor,
spotDateOffset,
businessDayAdjustment,
dayCount);
}
//-----------------------------------------------------------------------
/**
* Sets the label to use for the node, defaulted.
*
* When building, this will default based on the tenor if not specified.
* @param label the new value, not empty
* @return this, for chaining, not null
*/
public Builder label(String label) {
JodaBeanUtils.notEmpty(label, "label");
this.label = label;
return this;
}
/**
* Sets the identifier of the market data value that provides the rate.
* @param observableId the new value, not null
* @return this, for chaining, not null
*/
public Builder observableId(ObservableId observableId) {
JodaBeanUtils.notNull(observableId, "observableId");
this.observableId = observableId;
return this;
}
/**
* Sets the period between the start date and the end date.
* @param tenor the new value, not null
* @return this, for chaining, not null
*/
public Builder tenor(Tenor tenor) {
JodaBeanUtils.notNull(tenor, "tenor");
this.tenor = tenor;
return this;
}
/**
* Sets the offset of the start date from the trade date.
*
* The offset is applied to the trade date and is typically plus 2 business days.
* @param spotDateOffset the new value, not null
* @return this, for chaining, not null
*/
public Builder spotDateOffset(DaysAdjustment spotDateOffset) {
JodaBeanUtils.notNull(spotDateOffset, "spotDateOffset");
this.spotDateOffset = spotDateOffset;
return this;
}
/**
* Sets the business day adjustment to apply to the start and end date.
*
* The start and end date will be adjusted as defined here.
* @param businessDayAdjustment the new value, not null
* @return this, for chaining, not null
*/
public Builder businessDayAdjustment(BusinessDayAdjustment businessDayAdjustment) {
JodaBeanUtils.notNull(businessDayAdjustment, "businessDayAdjustment");
this.businessDayAdjustment = businessDayAdjustment;
return this;
}
/**
* Sets the day count convention.
*
* This defines the term year fraction.
* @param dayCount the new value, not null
* @return this, for chaining, not null
*/
public Builder dayCount(DayCount dayCount) {
JodaBeanUtils.notNull(dayCount, "dayCount");
this.dayCount = dayCount;
return this;
}
//-----------------------------------------------------------------------
@Override
public String toString() {
StringBuilder buf = new StringBuilder(224);
buf.append("DepositIsdaCreditCurveNode.Builder{");
buf.append("label").append('=').append(JodaBeanUtils.toString(label)).append(',').append(' ');
buf.append("observableId").append('=').append(JodaBeanUtils.toString(observableId)).append(',').append(' ');
buf.append("tenor").append('=').append(JodaBeanUtils.toString(tenor)).append(',').append(' ');
buf.append("spotDateOffset").append('=').append(JodaBeanUtils.toString(spotDateOffset)).append(',').append(' ');
buf.append("businessDayAdjustment").append('=').append(JodaBeanUtils.toString(businessDayAdjustment)).append(',').append(' ');
buf.append("dayCount").append('=').append(JodaBeanUtils.toString(dayCount));
buf.append('}');
return buf.toString();
}
}
//-------------------------- AUTOGENERATED END --------------------------
}