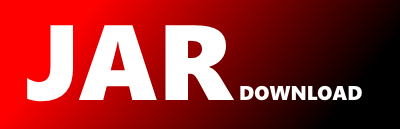
com.opengamma.strata.market.explain.ExplainMapBuilder Maven / Gradle / Ivy
Show all versions of strata-market Show documentation
/*
* Copyright (C) 2015 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.market.explain;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Consumer;
import com.opengamma.strata.collect.ArgChecker;
/**
* A builder for the map of explanatory values.
*
* This is a mutable builder for {@link ExplainMap} that must be used from a single thread.
*/
public final class ExplainMapBuilder {
/**
* The parent builder.
*/
private final ExplainMapBuilder parent;
/**
* The map of explanatory values.
*/
private final Map, Object> map = new LinkedHashMap<>();
/**
* Creates a new instance.
*/
ExplainMapBuilder() {
this.parent = null;
}
/**
* Creates a new instance.
*
* @param parent the parent builder
*/
ExplainMapBuilder(ExplainMapBuilder parent) {
this.parent = parent;
}
//-------------------------------------------------------------------------
/**
* Opens a list entry to be populated.
*
* This returns the builder for the new list entry.
* If the list does not exist, it is created and the first entry added.
* If the list has already been created, the entry is appended.
*
* Once opened, the child builder resulting from this method must be used.
* The method {@link #closeListEntry(ExplainKey)} must be used to close the
* child and receive an instance of the parent back again.
*
* @param the type of the value
* @param key the list key to open
* @return the child builder
*/
@SuppressWarnings("unchecked")
public > ExplainMapBuilder openListEntry(ExplainKey key) {
// list entry is a ExplainMapBuilder, making use of erasure in generics
// builder is converted to ExplainMap when entry is closed
ExplainMapBuilder child = new ExplainMapBuilder(this);
Object value = map.get(key);
ArrayList