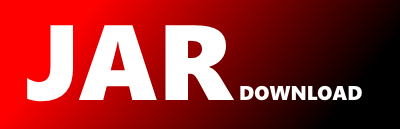
com.opengamma.strata.math.impl.minimization.NonLinearParameterTransforms Maven / Gradle / Ivy
Show all versions of strata-math Show documentation
/*
* Copyright (C) 2011 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.math.impl.minimization;
import com.opengamma.strata.collect.array.DoubleArray;
import com.opengamma.strata.collect.array.DoubleMatrix;
/**
* Describes the transformation (and its inverse) from a set of n variables (e.g. model parameters) to a set of m variables
* (e.g. fitting parameters), where m <= n.The principle use is in constrained optimisation, where the valid values of the parameters
* of a model live in some hyper-volume in R^n, but we wish to work with unconstrained variables in R^m.
* The model parameters are denoted as y and the unconstrained variables as y*, which are related by the vector function
* y* = f(y), and its inverse y = f-1(y*). The i,j element of the Jacobian is the rate of change of
* the ith element of y* with respect to the j th element of y, which is a (matrix) function
* of y, i.e. J(y). The inverse Jacobian is the rate of change of y with respect to
* y*, i.e. J-1(y*).
* These four functions must be provided by implementations of this interface.
*/
//CSOFF: JavadocMethod
public interface NonLinearParameterTransforms {
public abstract int getNumberOfModelParameters();
public abstract int getNumberOfFittingParameters();
/**
* Transforms from a set of model parameters to a (possibly smaller) set of unconstrained fitting parameters.
* @param modelParameters the model parameters
* @return The fitting parameters
*/
public abstract DoubleArray transform(DoubleArray modelParameters);
/**
* Transforms from a set of unconstrained fitting parameters to a (possibly larger) set of function parameters.
* @param fittingParameters The fitting parameters
* @return The model parameters
*/
public abstract DoubleArray inverseTransform(DoubleArray fittingParameters);
/**
* Calculates the Jacobian - the rate of change of the fitting parameters WRT the model parameters.
* @param modelParameters The model parameters
* @return The Jacobian
*/
public abstract DoubleMatrix jacobian(DoubleArray modelParameters);
/**
* Calculates the inverse Jacobian - the rate of change of the model parameters WRT the fitting parameters.
* @param fittingParameters The fitting parameters
* @return the inverse Jacobian
*/
public abstract DoubleMatrix inverseJacobian(DoubleArray fittingParameters);
}