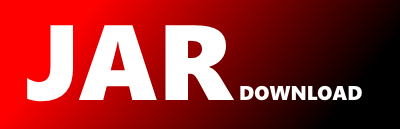
com.opengamma.strata.math.impl.rootfinding.RidderSingleRootFinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of strata-math Show documentation
Show all versions of strata-math Show documentation
Mathematic support for Strata
/*
* Copyright (C) 2009 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.math.impl.rootfinding;
import java.util.function.Function;
import org.apache.commons.math3.analysis.UnivariateFunction;
import org.apache.commons.math3.analysis.solvers.RiddersSolver;
import org.apache.commons.math3.exception.NoBracketingException;
import org.apache.commons.math3.exception.TooManyEvaluationsException;
import com.opengamma.strata.math.MathException;
import com.opengamma.strata.math.impl.util.CommonsMathWrapper;
/**
* Finds a single root of a function using Ridder's method. This class is a wrapper for the
* Commons Math library implementation
* of Ridder's method.
*/
public class RidderSingleRootFinder extends RealSingleRootFinder {
private static final int MAX_ITER = 100000;
private final RiddersSolver _ridder;
/**
* Sets the accuracy to 10-15.
*/
public RidderSingleRootFinder() {
this(1e-15);
}
/**
* @param functionValueAccuracy The accuracy of the function evaluations.
*/
public RidderSingleRootFinder(double functionValueAccuracy) {
_ridder = new RiddersSolver(functionValueAccuracy);
}
/**
* @param functionValueAccuracy The accuracy of the function evaluations.
* @param absoluteAccurary The maximum absolute error of the variable.
*/
public RidderSingleRootFinder(double functionValueAccuracy, double absoluteAccurary) {
_ridder = new RiddersSolver(functionValueAccuracy, absoluteAccurary);
}
/**
* {@inheritDoc}
* @throws MathException If the Commons method could not evaluate the function;
* if the Commons method could not converge.
*/
@Override
public Double getRoot(Function function, Double xLow, Double xHigh) {
checkInputs(function, xLow, xHigh);
UnivariateFunction wrapped = CommonsMathWrapper.wrapUnivariate(function);
try {
return _ridder.solve(MAX_ITER, wrapped, xLow, xHigh);
} catch (TooManyEvaluationsException | NoBracketingException e) {
throw new MathException(e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy