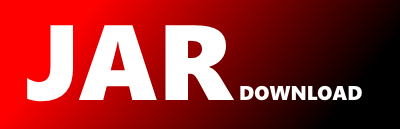
com.opengamma.strata.measure.ValuationZoneTimeDefinition Maven / Gradle / Ivy
/*
* Copyright (C) 2017 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.measure;
import java.io.Serializable;
import java.time.LocalDate;
import java.time.LocalTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import org.joda.beans.Bean;
import org.joda.beans.BeanBuilder;
import org.joda.beans.ImmutableBean;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectMetaBean;
import org.joda.beans.impl.direct.DirectMetaProperty;
import org.joda.beans.impl.direct.DirectMetaPropertyMap;
import org.joda.beans.impl.direct.DirectPrivateBeanBuilder;
import com.google.common.collect.ImmutableList;
import com.opengamma.strata.data.scenario.MarketDataBox;
/**
* Definition of valuation zone and time.
*
* This contains {@code ZoneId} and a set of {@code LocalTime} to create {@code ZonedDateTime} from {@code LocalDate}.
*/
@BeanDefinition(builderScope = "private")
public final class ValuationZoneTimeDefinition
implements ImmutableBean, Serializable {
/**
* The default local time.
*
* The default local time will be used if the input date is not scenario value or
* if the scenario size of the input date exceeds the size of {@code localTimes}.
*/
@PropertyDefinition(validate = "notNull")
private final LocalTime defaultLocalTime;
/**
* The zone ID.
*/
@PropertyDefinition(validate = "notNull")
private final ZoneId zoneId;
/**
* The local time.
*
* The local time in {@code zoneId}.
* The size is not necessarily the same as the scenario size.
* {@code defaultLocalTime} will be used if extra {@code LocalTime} is required.
*/
@PropertyDefinition(validate = "notNull")
private final ImmutableList localTimes;
//-------------------------------------------------------------------------
/**
* Obtains an instance.
*
* @param defaultLocalTime default local time
* @param zoneId the zone ID
* @param localTimes the local time
* @return the instance
*/
public static ValuationZoneTimeDefinition of(LocalTime defaultLocalTime, ZoneId zoneId, LocalTime... localTimes) {
return new ValuationZoneTimeDefinition(defaultLocalTime, zoneId, ImmutableList.copyOf(localTimes));
}
/**
* Creates zoned date time.
*
* If the scenario size of {@code dates} is greater than the size of {@code localTimes},
* {@code defaultLocalTime} is used.
* If {@code dates} is single value, {@code defaultLocalTime} is used.
*
* @param dates the local date
* @return the zoned date time
*/
public MarketDataBox toZonedDateTime(MarketDataBox dates) {
if (dates.isScenarioValue()) {
int nScenarios = dates.getScenarioCount();
int nTimes = localTimes.size();
List zonedDateTimes = IntStream.range(0, nScenarios)
.mapToObj(scenarioIndex -> zonedDateTime(dates.getValue(scenarioIndex), nTimes, scenarioIndex))
.collect(Collectors.toList());
return MarketDataBox.ofScenarioValues(zonedDateTimes);
}
ZonedDateTime zonedDateTime = dates.getSingleValue().atTime(defaultLocalTime).atZone(zoneId);
return MarketDataBox.ofSingleValue(zonedDateTime);
}
private ZonedDateTime zonedDateTime(LocalDate date, int nTimes, int scenarioIndex) {
return date.atTime(scenarioIndex < nTimes ? localTimes.get(scenarioIndex) : defaultLocalTime).atZone(zoneId);
}
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code ValuationZoneTimeDefinition}.
* @return the meta-bean, not null
*/
public static ValuationZoneTimeDefinition.Meta meta() {
return ValuationZoneTimeDefinition.Meta.INSTANCE;
}
static {
MetaBean.register(ValuationZoneTimeDefinition.Meta.INSTANCE);
}
/**
* The serialization version id.
*/
private static final long serialVersionUID = 1L;
private ValuationZoneTimeDefinition(
LocalTime defaultLocalTime,
ZoneId zoneId,
List localTimes) {
JodaBeanUtils.notNull(defaultLocalTime, "defaultLocalTime");
JodaBeanUtils.notNull(zoneId, "zoneId");
JodaBeanUtils.notNull(localTimes, "localTimes");
this.defaultLocalTime = defaultLocalTime;
this.zoneId = zoneId;
this.localTimes = ImmutableList.copyOf(localTimes);
}
@Override
public ValuationZoneTimeDefinition.Meta metaBean() {
return ValuationZoneTimeDefinition.Meta.INSTANCE;
}
//-----------------------------------------------------------------------
/**
* Gets the default local time.
*
* The default local time will be used if the input date is not scenario value or
* if the scenario size of the input date exceeds the size of {@code localTimes}.
* @return the value of the property, not null
*/
public LocalTime getDefaultLocalTime() {
return defaultLocalTime;
}
//-----------------------------------------------------------------------
/**
* Gets the zone ID.
* @return the value of the property, not null
*/
public ZoneId getZoneId() {
return zoneId;
}
//-----------------------------------------------------------------------
/**
* Gets the local time.
*
* The local time in {@code zoneId}.
* The size is not necessarily the same as the scenario size.
* {@code defaultLocalTime} will be used if extra {@code LocalTime} is required.
* @return the value of the property, not null
*/
public ImmutableList getLocalTimes() {
return localTimes;
}
//-----------------------------------------------------------------------
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
ValuationZoneTimeDefinition other = (ValuationZoneTimeDefinition) obj;
return JodaBeanUtils.equal(defaultLocalTime, other.defaultLocalTime) &&
JodaBeanUtils.equal(zoneId, other.zoneId) &&
JodaBeanUtils.equal(localTimes, other.localTimes);
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(defaultLocalTime);
hash = hash * 31 + JodaBeanUtils.hashCode(zoneId);
hash = hash * 31 + JodaBeanUtils.hashCode(localTimes);
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(128);
buf.append("ValuationZoneTimeDefinition{");
buf.append("defaultLocalTime").append('=').append(JodaBeanUtils.toString(defaultLocalTime)).append(',').append(' ');
buf.append("zoneId").append('=').append(JodaBeanUtils.toString(zoneId)).append(',').append(' ');
buf.append("localTimes").append('=').append(JodaBeanUtils.toString(localTimes));
buf.append('}');
return buf.toString();
}
//-----------------------------------------------------------------------
/**
* The meta-bean for {@code ValuationZoneTimeDefinition}.
*/
public static final class Meta extends DirectMetaBean {
/**
* The singleton instance of the meta-bean.
*/
static final Meta INSTANCE = new Meta();
/**
* The meta-property for the {@code defaultLocalTime} property.
*/
private final MetaProperty defaultLocalTime = DirectMetaProperty.ofImmutable(
this, "defaultLocalTime", ValuationZoneTimeDefinition.class, LocalTime.class);
/**
* The meta-property for the {@code zoneId} property.
*/
private final MetaProperty zoneId = DirectMetaProperty.ofImmutable(
this, "zoneId", ValuationZoneTimeDefinition.class, ZoneId.class);
/**
* The meta-property for the {@code localTimes} property.
*/
@SuppressWarnings({"unchecked", "rawtypes" })
private final MetaProperty> localTimes = DirectMetaProperty.ofImmutable(
this, "localTimes", ValuationZoneTimeDefinition.class, (Class) ImmutableList.class);
/**
* The meta-properties.
*/
private final Map> metaPropertyMap$ = new DirectMetaPropertyMap(
this, null,
"defaultLocalTime",
"zoneId",
"localTimes");
/**
* Restricted constructor.
*/
private Meta() {
}
@Override
protected MetaProperty> metaPropertyGet(String propertyName) {
switch (propertyName.hashCode()) {
case 605993879: // defaultLocalTime
return defaultLocalTime;
case -696323609: // zoneId
return zoneId;
case 1293230747: // localTimes
return localTimes;
}
return super.metaPropertyGet(propertyName);
}
@Override
public BeanBuilder extends ValuationZoneTimeDefinition> builder() {
return new ValuationZoneTimeDefinition.Builder();
}
@Override
public Class extends ValuationZoneTimeDefinition> beanType() {
return ValuationZoneTimeDefinition.class;
}
@Override
public Map> metaPropertyMap() {
return metaPropertyMap$;
}
//-----------------------------------------------------------------------
/**
* The meta-property for the {@code defaultLocalTime} property.
* @return the meta-property, not null
*/
public MetaProperty defaultLocalTime() {
return defaultLocalTime;
}
/**
* The meta-property for the {@code zoneId} property.
* @return the meta-property, not null
*/
public MetaProperty zoneId() {
return zoneId;
}
/**
* The meta-property for the {@code localTimes} property.
* @return the meta-property, not null
*/
public MetaProperty> localTimes() {
return localTimes;
}
//-----------------------------------------------------------------------
@Override
protected Object propertyGet(Bean bean, String propertyName, boolean quiet) {
switch (propertyName.hashCode()) {
case 605993879: // defaultLocalTime
return ((ValuationZoneTimeDefinition) bean).getDefaultLocalTime();
case -696323609: // zoneId
return ((ValuationZoneTimeDefinition) bean).getZoneId();
case 1293230747: // localTimes
return ((ValuationZoneTimeDefinition) bean).getLocalTimes();
}
return super.propertyGet(bean, propertyName, quiet);
}
@Override
protected void propertySet(Bean bean, String propertyName, Object newValue, boolean quiet) {
metaProperty(propertyName);
if (quiet) {
return;
}
throw new UnsupportedOperationException("Property cannot be written: " + propertyName);
}
}
//-----------------------------------------------------------------------
/**
* The bean-builder for {@code ValuationZoneTimeDefinition}.
*/
private static final class Builder extends DirectPrivateBeanBuilder {
private LocalTime defaultLocalTime;
private ZoneId zoneId;
private List localTimes = ImmutableList.of();
/**
* Restricted constructor.
*/
private Builder() {
}
//-----------------------------------------------------------------------
@Override
public Object get(String propertyName) {
switch (propertyName.hashCode()) {
case 605993879: // defaultLocalTime
return defaultLocalTime;
case -696323609: // zoneId
return zoneId;
case 1293230747: // localTimes
return localTimes;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
}
@SuppressWarnings("unchecked")
@Override
public Builder set(String propertyName, Object newValue) {
switch (propertyName.hashCode()) {
case 605993879: // defaultLocalTime
this.defaultLocalTime = (LocalTime) newValue;
break;
case -696323609: // zoneId
this.zoneId = (ZoneId) newValue;
break;
case 1293230747: // localTimes
this.localTimes = (List) newValue;
break;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
return this;
}
@Override
public ValuationZoneTimeDefinition build() {
return new ValuationZoneTimeDefinition(
defaultLocalTime,
zoneId,
localTimes);
}
//-----------------------------------------------------------------------
@Override
public String toString() {
StringBuilder buf = new StringBuilder(128);
buf.append("ValuationZoneTimeDefinition.Builder{");
buf.append("defaultLocalTime").append('=').append(JodaBeanUtils.toString(defaultLocalTime)).append(',').append(' ');
buf.append("zoneId").append('=').append(JodaBeanUtils.toString(zoneId)).append(',').append(' ');
buf.append("localTimes").append('=').append(JodaBeanUtils.toString(localTimes));
buf.append('}');
return buf.toString();
}
}
//-------------------------- AUTOGENERATED END --------------------------
}