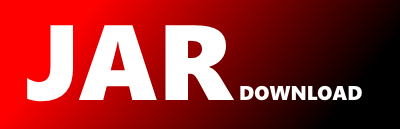
com.opengamma.strata.product.Attributes Maven / Gradle / Ivy
Show all versions of strata-product Show documentation
/*
* Copyright (C) 2018 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.product;
import java.util.Optional;
import com.google.common.collect.ImmutableSet;
import com.opengamma.strata.collect.Messages;
/**
* Additional attributes that can be associated with a model object.
*/
public interface Attributes {
/**
* Obtains an empty instance.
*
* The {@link #withAttribute(AttributeType, Object)} method can be used on
* the instance to add attributes.
*
* @return the empty instance
*/
public static Attributes empty() {
return SimpleAttributes.empty();
}
/**
* Obtains an instance with a single attribute.
*
* The {@link #withAttribute(AttributeType, Object)} method can be used on
* the instance to add more attributes.
*
* @param the type of the attribute value
* @param type the type providing meaning to the value
* @param value the value
* @return the instance
*/
public static Attributes of(AttributeType type, T value) {
return SimpleAttributes.of(type, value);
}
//-------------------------------------------------------------------------
/**
* Gets the attribute types that are available.
*
* See {@link AttributeType#captureWildcard()} for a way to capture the wildcard type.
*
* The default implementation returns an empty set (backwards compatibility prevents an abstract method for now).
*
* @return the attribute types
*/
public default ImmutableSet> getAttributeTypes() {
return ImmutableSet.of(); // TODO: Remove default in Strata v3
}
/**
* Gets the attribute associated with the specified type.
*
* This method obtains the specified attribute.
* This allows an attribute to be obtained if available.
*
* If the attribute is not found, an exception is thrown.
*
* @param the type of the attribute value
* @param type the type to find
* @return the attribute value
* @throws IllegalArgumentException if the attribute is not found
*/
public default T getAttribute(AttributeType type) {
return findAttribute(type).orElseThrow(() -> new IllegalArgumentException(
Messages.format("Attribute not found for type '{}'", type)));
}
/**
* Determines if an attribute associated with the specified type is present.
*
* @param the type of the attribute value
* @param type the type to find
* @return true if a matching attribute is present
*/
public default boolean containsAttribute(AttributeType type) {
return findAttribute(type).isPresent();
}
/**
* Determines if an attribute associated with the specified type is present and its value is {@code equal}
* to the supplied value.
*
* @param the type of the attribute value
* @param type the type to find
* @param attributeValue the value to match against
* @return true if a matching attribute is present
*/
public default boolean containsAttribute(AttributeType type, T attributeValue) {
return findAttribute(type).map(attribute -> attribute.equals(attributeValue)).orElse(false);
}
/**
* Finds the attribute associated with the specified type.
*
* This method obtains the specified attribute.
* This allows an attribute to be obtained if available.
*
* If the attribute is not found, optional empty is returned.
*
* @param the type of the result
* @param type the type to find
* @return the attribute value
*/
public abstract Optional findAttribute(AttributeType type);
/**
* Returns a copy of this instance with the attribute added.
*
* This returns a new instance with the specified attribute added.
* The attribute is added using {@code Map.put(type, value)} semantics.
*
* @param the type of the attribute value
* @param type the type providing meaning to the value
* @param value the value
* @return a new instance based on this one with the attribute added
*/
public abstract Attributes withAttribute(AttributeType type, T value);
/**
* Returns a copy of this instance with the attributes added.
*
* This returns a new instance with the specified attributes added.
* The attributes are added using {@code Map.putAll(type, value)} semantics.
*
* @param other the other instance to copy from
* @return an instance based on this one with the attributes from the other instance
*/
public default Attributes withAttributes(Attributes other) {
Attributes combined = this;
for (AttributeType> type : other.getAttributeTypes()) {
combined = combined.withAttribute(type.captureWildcard(), other.getAttribute(type));
}
return combined;
}
}