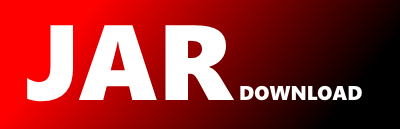
com.opengamma.strata.product.ItemInfo Maven / Gradle / Ivy
Show all versions of strata-product Show documentation
/*
* Copyright (C) 2018 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.product;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Optional;
import org.joda.beans.ImmutableBean;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.TypedMetaBean;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectPrivateBeanBuilder;
import org.joda.beans.impl.direct.MinimalMetaBean;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import com.opengamma.strata.basics.StandardId;
/**
* Additional information about a portfolio item instance.
*
* This allows additional information about an item to be associated.
* It is kept in a separate object as the information is optional for pricing.
*/
@BeanDefinition(style = "minimal", builderScope = "private", constructorScope = "package")
final class ItemInfo
implements PortfolioItemInfo, ImmutableBean, Serializable {
/**
* An empty instance of {@code ItemInfo}.
*/
private static final ItemInfo EMPTY = new ItemInfo(null, ImmutableMap.of());
/**
* The primary identifier for the item, optional.
*
* The identifier is used to identify the item.
* It will typically be an identifier in an external data system.
*
* An item may have multiple active identifiers. Any identifier may be chosen here.
* Certain uses of the identifier, such as storage in a database, require that the
* identifier does not change over time, and this should be considered best practice.
*/
@PropertyDefinition(get = "optional", overrideGet = true)
private final StandardId id;
/**
* The item attributes.
*
* Trade attributes provide the ability to associate arbitrary information in a key-value map.
*/
@PropertyDefinition(validate = "notNull")
private final ImmutableMap, Object> attributes;
//-------------------------------------------------------------------------
/**
* Obtains an empty instance, with no values or attributes.
*
* @return the empty instance
*/
static ItemInfo empty() {
return EMPTY;
}
//-------------------------------------------------------------------------
@Override
public ItemInfo withId(StandardId identifier) {
return new ItemInfo(identifier, attributes);
}
//-------------------------------------------------------------------------
@Override
public ImmutableSet> getAttributeTypes() {
return attributes.keySet();
}
@Override
public Optional findAttribute(AttributeType type) {
return Optional.ofNullable(type.fromStoredForm(attributes.get(type)));
}
@Override
public ItemInfo withAttribute(AttributeType type, T value) {
// ImmutableMap.Builder would not provide Map.put semantics
Map, Object> updatedAttributes = new HashMap<>(attributes);
if (value == null) {
updatedAttributes.remove(type);
} else {
updatedAttributes.put(type, type.toStoredForm(value));
}
return new ItemInfo(id, updatedAttributes);
}
@Override
public ItemInfo withAttributes(Attributes other) {
// ImmutableMap.Builder would not provide Map.put semantics
Map, Object> updatedAttributes = new HashMap<>(attributes);
for (AttributeType> type : other.getAttributeTypes()) {
updatedAttributes.put(type, type.captureWildcard().toStoredForm(other.getAttribute(type)));
}
return new ItemInfo(id, updatedAttributes);
}
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code ItemInfo}.
*/
private static final TypedMetaBean META_BEAN =
MinimalMetaBean.of(
ItemInfo.class,
new String[] {
"id",
"attributes"},
() -> new ItemInfo.Builder(),
b -> b.id,
b -> b.getAttributes());
/**
* The meta-bean for {@code ItemInfo}.
* @return the meta-bean, not null
*/
public static TypedMetaBean meta() {
return META_BEAN;
}
static {
MetaBean.register(META_BEAN);
}
/**
* The serialization version id.
*/
private static final long serialVersionUID = 1L;
/**
* Creates an instance.
* @param id the value of the property
* @param attributes the value of the property, not null
*/
ItemInfo(
StandardId id,
Map, Object> attributes) {
JodaBeanUtils.notNull(attributes, "attributes");
this.id = id;
this.attributes = ImmutableMap.copyOf(attributes);
}
@Override
public TypedMetaBean metaBean() {
return META_BEAN;
}
//-----------------------------------------------------------------------
/**
* Gets the primary identifier for the item, optional.
*
* The identifier is used to identify the item.
* It will typically be an identifier in an external data system.
*
* An item may have multiple active identifiers. Any identifier may be chosen here.
* Certain uses of the identifier, such as storage in a database, require that the
* identifier does not change over time, and this should be considered best practice.
* @return the optional value of the property, not null
*/
@Override
public Optional getId() {
return Optional.ofNullable(id);
}
//-----------------------------------------------------------------------
/**
* Gets the item attributes.
*
* Trade attributes provide the ability to associate arbitrary information in a key-value map.
* @return the value of the property, not null
*/
public ImmutableMap, Object> getAttributes() {
return attributes;
}
//-----------------------------------------------------------------------
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
ItemInfo other = (ItemInfo) obj;
return JodaBeanUtils.equal(id, other.id) &&
JodaBeanUtils.equal(attributes, other.attributes);
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(id);
hash = hash * 31 + JodaBeanUtils.hashCode(attributes);
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(96);
buf.append("ItemInfo{");
buf.append("id").append('=').append(JodaBeanUtils.toString(id)).append(',').append(' ');
buf.append("attributes").append('=').append(JodaBeanUtils.toString(attributes));
buf.append('}');
return buf.toString();
}
//-----------------------------------------------------------------------
/**
* The bean-builder for {@code ItemInfo}.
*/
private static final class Builder extends DirectPrivateBeanBuilder {
private StandardId id;
private Map, Object> attributes = ImmutableMap.of();
/**
* Restricted constructor.
*/
private Builder() {
}
//-----------------------------------------------------------------------
@Override
public Object get(String propertyName) {
switch (propertyName.hashCode()) {
case 3355: // id
return id;
case 405645655: // attributes
return attributes;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
}
@SuppressWarnings("unchecked")
@Override
public Builder set(String propertyName, Object newValue) {
switch (propertyName.hashCode()) {
case 3355: // id
this.id = (StandardId) newValue;
break;
case 405645655: // attributes
this.attributes = (Map, Object>) newValue;
break;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
return this;
}
@Override
public ItemInfo build() {
return new ItemInfo(
id,
attributes);
}
//-----------------------------------------------------------------------
@Override
public String toString() {
StringBuilder buf = new StringBuilder(96);
buf.append("ItemInfo.Builder{");
buf.append("id").append('=').append(JodaBeanUtils.toString(id)).append(',').append(' ');
buf.append("attributes").append('=').append(JodaBeanUtils.toString(attributes));
buf.append('}');
return buf.toString();
}
}
//-------------------------- AUTOGENERATED END --------------------------
}