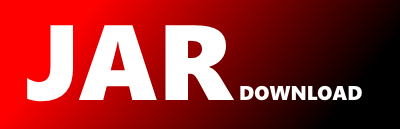
com.opengamma.strata.product.PortfolioItemSummary Maven / Gradle / Ivy
Show all versions of strata-product Show documentation
/*
* Copyright (C) 2017 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.product;
import java.io.Serializable;
import java.util.NoSuchElementException;
import java.util.Optional;
import java.util.Set;
import org.joda.beans.ImmutableBean;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.TypedMetaBean;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectFieldsBeanBuilder;
import org.joda.beans.impl.direct.MinimalMetaBean;
import com.google.common.collect.ImmutableSet;
import com.opengamma.strata.basics.StandardId;
import com.opengamma.strata.basics.currency.Currency;
import com.opengamma.strata.collect.named.Described;
/**
* A summary of a portfolio item.
*
* This can be used to display a summary of a portfolio to a user.
*/
@BeanDefinition(style = "minimal", metaScope = "private", factoryName = "of")
public final class PortfolioItemSummary
implements Described, ImmutableBean, Serializable {
/**
* The identifier of the item, optional.
*/
@PropertyDefinition(get = "optional")
private final StandardId id;
/**
* The type of the item.
*/
@PropertyDefinition(validate = "notNull")
private final PortfolioItemType portfolioItemType;
/**
* The type of the product.
*/
@PropertyDefinition(validate = "notNull")
private final ProductType productType;
/**
* The currencies of the item.
*
* This should include the primary currencies the item is based on, not just the payment currencies.
*/
@PropertyDefinition(validate = "notNull")
private final ImmutableSet currencies;
/**
* The description of the item.
*/
@PropertyDefinition(validate = "notBlank", overrideGet = true)
private final String description;
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code PortfolioItemSummary}.
*/
private static final TypedMetaBean META_BEAN =
MinimalMetaBean.of(
PortfolioItemSummary.class,
new String[] {
"id",
"portfolioItemType",
"productType",
"currencies",
"description"},
() -> new PortfolioItemSummary.Builder(),
b -> b.id,
b -> b.getPortfolioItemType(),
b -> b.getProductType(),
b -> b.getCurrencies(),
b -> b.getDescription());
/**
* The meta-bean for {@code PortfolioItemSummary}.
* @return the meta-bean, not null
*/
public static TypedMetaBean meta() {
return META_BEAN;
}
static {
MetaBean.register(META_BEAN);
}
/**
* The serialization version id.
*/
private static final long serialVersionUID = 1L;
/**
* Obtains an instance.
* @param id the value of the property
* @param portfolioItemType the value of the property, not null
* @param productType the value of the property, not null
* @param currencies the value of the property, not null
* @param description the value of the property, not blank
* @return the instance
*/
public static PortfolioItemSummary of(
StandardId id,
PortfolioItemType portfolioItemType,
ProductType productType,
Set currencies,
String description) {
return new PortfolioItemSummary(
id,
portfolioItemType,
productType,
currencies,
description);
}
/**
* Returns a builder used to create an instance of the bean.
* @return the builder, not null
*/
public static PortfolioItemSummary.Builder builder() {
return new PortfolioItemSummary.Builder();
}
private PortfolioItemSummary(
StandardId id,
PortfolioItemType portfolioItemType,
ProductType productType,
Set currencies,
String description) {
JodaBeanUtils.notNull(portfolioItemType, "portfolioItemType");
JodaBeanUtils.notNull(productType, "productType");
JodaBeanUtils.notNull(currencies, "currencies");
JodaBeanUtils.notBlank(description, "description");
this.id = id;
this.portfolioItemType = portfolioItemType;
this.productType = productType;
this.currencies = ImmutableSet.copyOf(currencies);
this.description = description;
}
@Override
public TypedMetaBean metaBean() {
return META_BEAN;
}
//-----------------------------------------------------------------------
/**
* Gets the identifier of the item, optional.
* @return the optional value of the property, not null
*/
public Optional getId() {
return Optional.ofNullable(id);
}
//-----------------------------------------------------------------------
/**
* Gets the type of the item.
* @return the value of the property, not null
*/
public PortfolioItemType getPortfolioItemType() {
return portfolioItemType;
}
//-----------------------------------------------------------------------
/**
* Gets the type of the product.
* @return the value of the property, not null
*/
public ProductType getProductType() {
return productType;
}
//-----------------------------------------------------------------------
/**
* Gets the currencies of the item.
*
* This should include the primary currencies the item is based on, not just the payment currencies.
* @return the value of the property, not null
*/
public ImmutableSet getCurrencies() {
return currencies;
}
//-----------------------------------------------------------------------
/**
* Gets the description of the item.
* @return the value of the property, not blank
*/
@Override
public String getDescription() {
return description;
}
//-----------------------------------------------------------------------
/**
* Returns a builder that allows this bean to be mutated.
* @return the mutable builder, not null
*/
public Builder toBuilder() {
return new Builder(this);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
PortfolioItemSummary other = (PortfolioItemSummary) obj;
return JodaBeanUtils.equal(id, other.id) &&
JodaBeanUtils.equal(portfolioItemType, other.portfolioItemType) &&
JodaBeanUtils.equal(productType, other.productType) &&
JodaBeanUtils.equal(currencies, other.currencies) &&
JodaBeanUtils.equal(description, other.description);
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(id);
hash = hash * 31 + JodaBeanUtils.hashCode(portfolioItemType);
hash = hash * 31 + JodaBeanUtils.hashCode(productType);
hash = hash * 31 + JodaBeanUtils.hashCode(currencies);
hash = hash * 31 + JodaBeanUtils.hashCode(description);
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(192);
buf.append("PortfolioItemSummary{");
buf.append("id").append('=').append(JodaBeanUtils.toString(id)).append(',').append(' ');
buf.append("portfolioItemType").append('=').append(JodaBeanUtils.toString(portfolioItemType)).append(',').append(' ');
buf.append("productType").append('=').append(JodaBeanUtils.toString(productType)).append(',').append(' ');
buf.append("currencies").append('=').append(JodaBeanUtils.toString(currencies)).append(',').append(' ');
buf.append("description").append('=').append(JodaBeanUtils.toString(description));
buf.append('}');
return buf.toString();
}
//-----------------------------------------------------------------------
/**
* The bean-builder for {@code PortfolioItemSummary}.
*/
public static final class Builder extends DirectFieldsBeanBuilder {
private StandardId id;
private PortfolioItemType portfolioItemType;
private ProductType productType;
private Set currencies = ImmutableSet.of();
private String description;
/**
* Restricted constructor.
*/
private Builder() {
}
/**
* Restricted copy constructor.
* @param beanToCopy the bean to copy from, not null
*/
private Builder(PortfolioItemSummary beanToCopy) {
this.id = beanToCopy.id;
this.portfolioItemType = beanToCopy.getPortfolioItemType();
this.productType = beanToCopy.getProductType();
this.currencies = beanToCopy.getCurrencies();
this.description = beanToCopy.getDescription();
}
//-----------------------------------------------------------------------
@Override
public Object get(String propertyName) {
switch (propertyName.hashCode()) {
case 3355: // id
return id;
case 1766940245: // portfolioItemType
return portfolioItemType;
case -1491615543: // productType
return productType;
case -1089470353: // currencies
return currencies;
case -1724546052: // description
return description;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
}
@SuppressWarnings("unchecked")
@Override
public Builder set(String propertyName, Object newValue) {
switch (propertyName.hashCode()) {
case 3355: // id
this.id = (StandardId) newValue;
break;
case 1766940245: // portfolioItemType
this.portfolioItemType = (PortfolioItemType) newValue;
break;
case -1491615543: // productType
this.productType = (ProductType) newValue;
break;
case -1089470353: // currencies
this.currencies = (Set) newValue;
break;
case -1724546052: // description
this.description = (String) newValue;
break;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
return this;
}
@Override
public Builder set(MetaProperty> property, Object value) {
super.set(property, value);
return this;
}
@Override
public PortfolioItemSummary build() {
return new PortfolioItemSummary(
id,
portfolioItemType,
productType,
currencies,
description);
}
//-----------------------------------------------------------------------
/**
* Sets the identifier of the item, optional.
* @param id the new value
* @return this, for chaining, not null
*/
public Builder id(StandardId id) {
this.id = id;
return this;
}
/**
* Sets the type of the item.
* @param portfolioItemType the new value, not null
* @return this, for chaining, not null
*/
public Builder portfolioItemType(PortfolioItemType portfolioItemType) {
JodaBeanUtils.notNull(portfolioItemType, "portfolioItemType");
this.portfolioItemType = portfolioItemType;
return this;
}
/**
* Sets the type of the product.
* @param productType the new value, not null
* @return this, for chaining, not null
*/
public Builder productType(ProductType productType) {
JodaBeanUtils.notNull(productType, "productType");
this.productType = productType;
return this;
}
/**
* Sets the currencies of the item.
*
* This should include the primary currencies the item is based on, not just the payment currencies.
* @param currencies the new value, not null
* @return this, for chaining, not null
*/
public Builder currencies(Set currencies) {
JodaBeanUtils.notNull(currencies, "currencies");
this.currencies = currencies;
return this;
}
/**
* Sets the {@code currencies} property in the builder
* from an array of objects.
* @param currencies the new value, not null
* @return this, for chaining, not null
*/
public Builder currencies(Currency... currencies) {
return currencies(ImmutableSet.copyOf(currencies));
}
/**
* Sets the description of the item.
* @param description the new value, not blank
* @return this, for chaining, not null
*/
public Builder description(String description) {
JodaBeanUtils.notBlank(description, "description");
this.description = description;
return this;
}
//-----------------------------------------------------------------------
@Override
public String toString() {
StringBuilder buf = new StringBuilder(192);
buf.append("PortfolioItemSummary.Builder{");
buf.append("id").append('=').append(JodaBeanUtils.toString(id)).append(',').append(' ');
buf.append("portfolioItemType").append('=').append(JodaBeanUtils.toString(portfolioItemType)).append(',').append(' ');
buf.append("productType").append('=').append(JodaBeanUtils.toString(productType)).append(',').append(' ');
buf.append("currencies").append('=').append(JodaBeanUtils.toString(currencies)).append(',').append(' ');
buf.append("description").append('=').append(JodaBeanUtils.toString(description));
buf.append('}');
return buf.toString();
}
}
//-------------------------- AUTOGENERATED END --------------------------
}