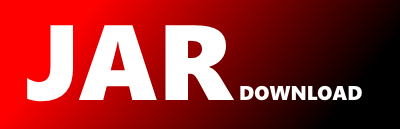
com.opengamma.strata.product.rate.OvernightCompoundedRateComputation Maven / Gradle / Ivy
Show all versions of strata-product Show documentation
/*
* Copyright (C) 2014 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.product.rate;
import java.io.Serializable;
import java.time.LocalDate;
import java.util.Map;
import java.util.NoSuchElementException;
import org.joda.beans.Bean;
import org.joda.beans.ImmutableBean;
import org.joda.beans.JodaBeanUtils;
import org.joda.beans.MetaBean;
import org.joda.beans.MetaProperty;
import org.joda.beans.gen.BeanDefinition;
import org.joda.beans.gen.ImmutableValidator;
import org.joda.beans.gen.PropertyDefinition;
import org.joda.beans.impl.direct.DirectFieldsBeanBuilder;
import org.joda.beans.impl.direct.DirectMetaBean;
import org.joda.beans.impl.direct.DirectMetaProperty;
import org.joda.beans.impl.direct.DirectMetaPropertyMap;
import com.opengamma.strata.basics.ReferenceData;
import com.opengamma.strata.basics.date.HolidayCalendar;
import com.opengamma.strata.basics.index.OvernightIndex;
import com.opengamma.strata.collect.ArgChecker;
/**
* Defines the computation of a rate from a single Overnight index that is compounded daily.
*
* An interest rate determined directly from an Overnight index with daily compounding.
* For example, a rate determined by compounding values from 'GBP-SONIA'.
*/
@BeanDefinition
public final class OvernightCompoundedRateComputation
implements OvernightRateComputation, ImmutableBean, Serializable {
/**
* The Overnight index.
*
* The rate to be paid is based on this index.
* It will be a well known market index such as 'GBP-SONIA'.
*/
@PropertyDefinition(validate = "notNull", overrideGet = true)
private final OvernightIndex index;
/**
* The resolved calendar that the index uses.
*/
@PropertyDefinition(validate = "notNull", overrideGet = true)
private final HolidayCalendar fixingCalendar;
/**
* The fixing date associated with the start date of the accrual period.
*
* This is also the first fixing date.
* The overnight rate is observed from this date onwards.
*
* In general, the fixing dates and accrual dates are the same for an overnight index.
* However, in the case of a Tomorrow/Next index, the fixing period is one business day
* before the accrual period.
*/
@PropertyDefinition(validate = "notNull", overrideGet = true)
private final LocalDate startDate;
/**
* The fixing date associated with the end date of the accrual period.
*
* The overnight rate is observed until this date.
*
* In general, the fixing dates and accrual dates are the same for an overnight index.
* However, in the case of a Tomorrow/Next index, the fixing period is one business day
* before the accrual period.
*/
@PropertyDefinition(validate = "notNull", overrideGet = true)
private final LocalDate endDate;
/**
* The number of business days before the end of the period that the rate is cut off.
*
* When a rate cut-off applies, the final daily rate is determined this number of days
* before the end of the period, with any subsequent days having the same rate.
*
* The amount must be zero or positive.
* A value of zero or one will have no effect on the standard calculation.
* The fixing holiday calendar of the index is used to determine business days.
*
* For example, a value of {@code 3} means that the rate observed on
* {@code (periodEndDate - 3 business days)} is also to be used on
* {@code (periodEndDate - 2 business days)} and {@code (periodEndDate - 1 business day)}.
*
* If there are multiple accrual periods in the payment period, then this
* should typically only be non-zero in the last accrual period.
*/
@PropertyDefinition(validate = "ArgChecker.notNegative")
private final int rateCutOffDays;
//-------------------------------------------------------------------------
/**
* Creates an instance from an index and period dates
*
* No rate cut-off applies.
*
* @param index the index
* @param startDate the first date of the accrual period
* @param endDate the last date of the accrual period
* @param refData the reference data to use when resolving holiday calendars
* @return the rate computation
*/
public static OvernightCompoundedRateComputation of(
OvernightIndex index,
LocalDate startDate,
LocalDate endDate,
ReferenceData refData) {
return of(index, startDate, endDate, 0, refData);
}
/**
* Creates an instance from an index, period dates and rate cut-off.
*
* Rate cut-off applies if the cut-off is 2 or greater.
* A value of 0 or 1 should be used if no cut-off applies.
*
* @param index the index
* @param startDate the first date of the accrual period
* @param endDate the last date of the accrual period
* @param rateCutOffDays the rate cut-off days offset, not negative
* @param refData the reference data to use when resolving holiday calendars
* @return the rate computation
*/
public static OvernightCompoundedRateComputation of(
OvernightIndex index,
LocalDate startDate,
LocalDate endDate,
int rateCutOffDays,
ReferenceData refData) {
return OvernightCompoundedRateComputation.builder()
.index(index)
.fixingCalendar(index.getFixingCalendar().resolve(refData))
.startDate(index.calculateFixingFromEffective(startDate, refData))
.endDate(index.calculateFixingFromEffective(endDate, refData))
.rateCutOffDays(rateCutOffDays)
.build();
}
//-------------------------------------------------------------------------
@ImmutableValidator
private void validate() {
ArgChecker.inOrderNotEqual(startDate, endDate, "startDate", "endDate");
}
//------------------------- AUTOGENERATED START -------------------------
/**
* The meta-bean for {@code OvernightCompoundedRateComputation}.
* @return the meta-bean, not null
*/
public static OvernightCompoundedRateComputation.Meta meta() {
return OvernightCompoundedRateComputation.Meta.INSTANCE;
}
static {
MetaBean.register(OvernightCompoundedRateComputation.Meta.INSTANCE);
}
/**
* The serialization version id.
*/
private static final long serialVersionUID = 1L;
/**
* Returns a builder used to create an instance of the bean.
* @return the builder, not null
*/
public static OvernightCompoundedRateComputation.Builder builder() {
return new OvernightCompoundedRateComputation.Builder();
}
private OvernightCompoundedRateComputation(
OvernightIndex index,
HolidayCalendar fixingCalendar,
LocalDate startDate,
LocalDate endDate,
int rateCutOffDays) {
JodaBeanUtils.notNull(index, "index");
JodaBeanUtils.notNull(fixingCalendar, "fixingCalendar");
JodaBeanUtils.notNull(startDate, "startDate");
JodaBeanUtils.notNull(endDate, "endDate");
ArgChecker.notNegative(rateCutOffDays, "rateCutOffDays");
this.index = index;
this.fixingCalendar = fixingCalendar;
this.startDate = startDate;
this.endDate = endDate;
this.rateCutOffDays = rateCutOffDays;
validate();
}
@Override
public OvernightCompoundedRateComputation.Meta metaBean() {
return OvernightCompoundedRateComputation.Meta.INSTANCE;
}
//-----------------------------------------------------------------------
/**
* Gets the Overnight index.
*
* The rate to be paid is based on this index.
* It will be a well known market index such as 'GBP-SONIA'.
* @return the value of the property, not null
*/
@Override
public OvernightIndex getIndex() {
return index;
}
//-----------------------------------------------------------------------
/**
* Gets the resolved calendar that the index uses.
* @return the value of the property, not null
*/
@Override
public HolidayCalendar getFixingCalendar() {
return fixingCalendar;
}
//-----------------------------------------------------------------------
/**
* Gets the fixing date associated with the start date of the accrual period.
*
* This is also the first fixing date.
* The overnight rate is observed from this date onwards.
*
* In general, the fixing dates and accrual dates are the same for an overnight index.
* However, in the case of a Tomorrow/Next index, the fixing period is one business day
* before the accrual period.
* @return the value of the property, not null
*/
@Override
public LocalDate getStartDate() {
return startDate;
}
//-----------------------------------------------------------------------
/**
* Gets the fixing date associated with the end date of the accrual period.
*
* The overnight rate is observed until this date.
*
* In general, the fixing dates and accrual dates are the same for an overnight index.
* However, in the case of a Tomorrow/Next index, the fixing period is one business day
* before the accrual period.
* @return the value of the property, not null
*/
@Override
public LocalDate getEndDate() {
return endDate;
}
//-----------------------------------------------------------------------
/**
* Gets the number of business days before the end of the period that the rate is cut off.
*
* When a rate cut-off applies, the final daily rate is determined this number of days
* before the end of the period, with any subsequent days having the same rate.
*
* The amount must be zero or positive.
* A value of zero or one will have no effect on the standard calculation.
* The fixing holiday calendar of the index is used to determine business days.
*
* For example, a value of {@code 3} means that the rate observed on
* {@code (periodEndDate - 3 business days)} is also to be used on
* {@code (periodEndDate - 2 business days)} and {@code (periodEndDate - 1 business day)}.
*
* If there are multiple accrual periods in the payment period, then this
* should typically only be non-zero in the last accrual period.
* @return the value of the property
*/
public int getRateCutOffDays() {
return rateCutOffDays;
}
//-----------------------------------------------------------------------
/**
* Returns a builder that allows this bean to be mutated.
* @return the mutable builder, not null
*/
public Builder toBuilder() {
return new Builder(this);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (obj != null && obj.getClass() == this.getClass()) {
OvernightCompoundedRateComputation other = (OvernightCompoundedRateComputation) obj;
return JodaBeanUtils.equal(index, other.index) &&
JodaBeanUtils.equal(fixingCalendar, other.fixingCalendar) &&
JodaBeanUtils.equal(startDate, other.startDate) &&
JodaBeanUtils.equal(endDate, other.endDate) &&
(rateCutOffDays == other.rateCutOffDays);
}
return false;
}
@Override
public int hashCode() {
int hash = getClass().hashCode();
hash = hash * 31 + JodaBeanUtils.hashCode(index);
hash = hash * 31 + JodaBeanUtils.hashCode(fixingCalendar);
hash = hash * 31 + JodaBeanUtils.hashCode(startDate);
hash = hash * 31 + JodaBeanUtils.hashCode(endDate);
hash = hash * 31 + JodaBeanUtils.hashCode(rateCutOffDays);
return hash;
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder(192);
buf.append("OvernightCompoundedRateComputation{");
buf.append("index").append('=').append(JodaBeanUtils.toString(index)).append(',').append(' ');
buf.append("fixingCalendar").append('=').append(JodaBeanUtils.toString(fixingCalendar)).append(',').append(' ');
buf.append("startDate").append('=').append(JodaBeanUtils.toString(startDate)).append(',').append(' ');
buf.append("endDate").append('=').append(JodaBeanUtils.toString(endDate)).append(',').append(' ');
buf.append("rateCutOffDays").append('=').append(JodaBeanUtils.toString(rateCutOffDays));
buf.append('}');
return buf.toString();
}
//-----------------------------------------------------------------------
/**
* The meta-bean for {@code OvernightCompoundedRateComputation}.
*/
public static final class Meta extends DirectMetaBean {
/**
* The singleton instance of the meta-bean.
*/
static final Meta INSTANCE = new Meta();
/**
* The meta-property for the {@code index} property.
*/
private final MetaProperty index = DirectMetaProperty.ofImmutable(
this, "index", OvernightCompoundedRateComputation.class, OvernightIndex.class);
/**
* The meta-property for the {@code fixingCalendar} property.
*/
private final MetaProperty fixingCalendar = DirectMetaProperty.ofImmutable(
this, "fixingCalendar", OvernightCompoundedRateComputation.class, HolidayCalendar.class);
/**
* The meta-property for the {@code startDate} property.
*/
private final MetaProperty startDate = DirectMetaProperty.ofImmutable(
this, "startDate", OvernightCompoundedRateComputation.class, LocalDate.class);
/**
* The meta-property for the {@code endDate} property.
*/
private final MetaProperty endDate = DirectMetaProperty.ofImmutable(
this, "endDate", OvernightCompoundedRateComputation.class, LocalDate.class);
/**
* The meta-property for the {@code rateCutOffDays} property.
*/
private final MetaProperty rateCutOffDays = DirectMetaProperty.ofImmutable(
this, "rateCutOffDays", OvernightCompoundedRateComputation.class, Integer.TYPE);
/**
* The meta-properties.
*/
private final Map> metaPropertyMap$ = new DirectMetaPropertyMap(
this, null,
"index",
"fixingCalendar",
"startDate",
"endDate",
"rateCutOffDays");
/**
* Restricted constructor.
*/
private Meta() {
}
@Override
protected MetaProperty> metaPropertyGet(String propertyName) {
switch (propertyName.hashCode()) {
case 100346066: // index
return index;
case 394230283: // fixingCalendar
return fixingCalendar;
case -2129778896: // startDate
return startDate;
case -1607727319: // endDate
return endDate;
case -92095804: // rateCutOffDays
return rateCutOffDays;
}
return super.metaPropertyGet(propertyName);
}
@Override
public OvernightCompoundedRateComputation.Builder builder() {
return new OvernightCompoundedRateComputation.Builder();
}
@Override
public Class extends OvernightCompoundedRateComputation> beanType() {
return OvernightCompoundedRateComputation.class;
}
@Override
public Map> metaPropertyMap() {
return metaPropertyMap$;
}
//-----------------------------------------------------------------------
/**
* The meta-property for the {@code index} property.
* @return the meta-property, not null
*/
public MetaProperty index() {
return index;
}
/**
* The meta-property for the {@code fixingCalendar} property.
* @return the meta-property, not null
*/
public MetaProperty fixingCalendar() {
return fixingCalendar;
}
/**
* The meta-property for the {@code startDate} property.
* @return the meta-property, not null
*/
public MetaProperty startDate() {
return startDate;
}
/**
* The meta-property for the {@code endDate} property.
* @return the meta-property, not null
*/
public MetaProperty endDate() {
return endDate;
}
/**
* The meta-property for the {@code rateCutOffDays} property.
* @return the meta-property, not null
*/
public MetaProperty rateCutOffDays() {
return rateCutOffDays;
}
//-----------------------------------------------------------------------
@Override
protected Object propertyGet(Bean bean, String propertyName, boolean quiet) {
switch (propertyName.hashCode()) {
case 100346066: // index
return ((OvernightCompoundedRateComputation) bean).getIndex();
case 394230283: // fixingCalendar
return ((OvernightCompoundedRateComputation) bean).getFixingCalendar();
case -2129778896: // startDate
return ((OvernightCompoundedRateComputation) bean).getStartDate();
case -1607727319: // endDate
return ((OvernightCompoundedRateComputation) bean).getEndDate();
case -92095804: // rateCutOffDays
return ((OvernightCompoundedRateComputation) bean).getRateCutOffDays();
}
return super.propertyGet(bean, propertyName, quiet);
}
@Override
protected void propertySet(Bean bean, String propertyName, Object newValue, boolean quiet) {
metaProperty(propertyName);
if (quiet) {
return;
}
throw new UnsupportedOperationException("Property cannot be written: " + propertyName);
}
}
//-----------------------------------------------------------------------
/**
* The bean-builder for {@code OvernightCompoundedRateComputation}.
*/
public static final class Builder extends DirectFieldsBeanBuilder {
private OvernightIndex index;
private HolidayCalendar fixingCalendar;
private LocalDate startDate;
private LocalDate endDate;
private int rateCutOffDays;
/**
* Restricted constructor.
*/
private Builder() {
}
/**
* Restricted copy constructor.
* @param beanToCopy the bean to copy from, not null
*/
private Builder(OvernightCompoundedRateComputation beanToCopy) {
this.index = beanToCopy.getIndex();
this.fixingCalendar = beanToCopy.getFixingCalendar();
this.startDate = beanToCopy.getStartDate();
this.endDate = beanToCopy.getEndDate();
this.rateCutOffDays = beanToCopy.getRateCutOffDays();
}
//-----------------------------------------------------------------------
@Override
public Object get(String propertyName) {
switch (propertyName.hashCode()) {
case 100346066: // index
return index;
case 394230283: // fixingCalendar
return fixingCalendar;
case -2129778896: // startDate
return startDate;
case -1607727319: // endDate
return endDate;
case -92095804: // rateCutOffDays
return rateCutOffDays;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
}
@Override
public Builder set(String propertyName, Object newValue) {
switch (propertyName.hashCode()) {
case 100346066: // index
this.index = (OvernightIndex) newValue;
break;
case 394230283: // fixingCalendar
this.fixingCalendar = (HolidayCalendar) newValue;
break;
case -2129778896: // startDate
this.startDate = (LocalDate) newValue;
break;
case -1607727319: // endDate
this.endDate = (LocalDate) newValue;
break;
case -92095804: // rateCutOffDays
this.rateCutOffDays = (Integer) newValue;
break;
default:
throw new NoSuchElementException("Unknown property: " + propertyName);
}
return this;
}
@Override
public Builder set(MetaProperty> property, Object value) {
super.set(property, value);
return this;
}
@Override
public OvernightCompoundedRateComputation build() {
return new OvernightCompoundedRateComputation(
index,
fixingCalendar,
startDate,
endDate,
rateCutOffDays);
}
//-----------------------------------------------------------------------
/**
* Sets the Overnight index.
*
* The rate to be paid is based on this index.
* It will be a well known market index such as 'GBP-SONIA'.
* @param index the new value, not null
* @return this, for chaining, not null
*/
public Builder index(OvernightIndex index) {
JodaBeanUtils.notNull(index, "index");
this.index = index;
return this;
}
/**
* Sets the resolved calendar that the index uses.
* @param fixingCalendar the new value, not null
* @return this, for chaining, not null
*/
public Builder fixingCalendar(HolidayCalendar fixingCalendar) {
JodaBeanUtils.notNull(fixingCalendar, "fixingCalendar");
this.fixingCalendar = fixingCalendar;
return this;
}
/**
* Sets the fixing date associated with the start date of the accrual period.
*
* This is also the first fixing date.
* The overnight rate is observed from this date onwards.
*
* In general, the fixing dates and accrual dates are the same for an overnight index.
* However, in the case of a Tomorrow/Next index, the fixing period is one business day
* before the accrual period.
* @param startDate the new value, not null
* @return this, for chaining, not null
*/
public Builder startDate(LocalDate startDate) {
JodaBeanUtils.notNull(startDate, "startDate");
this.startDate = startDate;
return this;
}
/**
* Sets the fixing date associated with the end date of the accrual period.
*
* The overnight rate is observed until this date.
*
* In general, the fixing dates and accrual dates are the same for an overnight index.
* However, in the case of a Tomorrow/Next index, the fixing period is one business day
* before the accrual period.
* @param endDate the new value, not null
* @return this, for chaining, not null
*/
public Builder endDate(LocalDate endDate) {
JodaBeanUtils.notNull(endDate, "endDate");
this.endDate = endDate;
return this;
}
/**
* Sets the number of business days before the end of the period that the rate is cut off.
*
* When a rate cut-off applies, the final daily rate is determined this number of days
* before the end of the period, with any subsequent days having the same rate.
*
* The amount must be zero or positive.
* A value of zero or one will have no effect on the standard calculation.
* The fixing holiday calendar of the index is used to determine business days.
*
* For example, a value of {@code 3} means that the rate observed on
* {@code (periodEndDate - 3 business days)} is also to be used on
* {@code (periodEndDate - 2 business days)} and {@code (periodEndDate - 1 business day)}.
*
* If there are multiple accrual periods in the payment period, then this
* should typically only be non-zero in the last accrual period.
* @param rateCutOffDays the new value
* @return this, for chaining, not null
*/
public Builder rateCutOffDays(int rateCutOffDays) {
ArgChecker.notNegative(rateCutOffDays, "rateCutOffDays");
this.rateCutOffDays = rateCutOffDays;
return this;
}
//-----------------------------------------------------------------------
@Override
public String toString() {
StringBuilder buf = new StringBuilder(192);
buf.append("OvernightCompoundedRateComputation.Builder{");
buf.append("index").append('=').append(JodaBeanUtils.toString(index)).append(',').append(' ');
buf.append("fixingCalendar").append('=').append(JodaBeanUtils.toString(fixingCalendar)).append(',').append(' ');
buf.append("startDate").append('=').append(JodaBeanUtils.toString(startDate)).append(',').append(' ');
buf.append("endDate").append('=').append(JodaBeanUtils.toString(endDate)).append(',').append(' ');
buf.append("rateCutOffDays").append('=').append(JodaBeanUtils.toString(rateCutOffDays));
buf.append('}');
return buf.toString();
}
}
//-------------------------- AUTOGENERATED END --------------------------
}