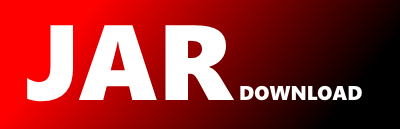
com.opengamma.strata.product.swap.SwapIndex Maven / Gradle / Ivy
Show all versions of strata-product Show documentation
/*
* Copyright (C) 2015 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.product.swap;
import java.time.LocalDate;
import java.time.LocalTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
import org.joda.convert.FromString;
import org.joda.convert.ToString;
import com.opengamma.strata.basics.index.Index;
import com.opengamma.strata.collect.ArgChecker;
import com.opengamma.strata.collect.named.ExtendedEnum;
import com.opengamma.strata.collect.named.Named;
import com.opengamma.strata.product.swap.type.FixedFloatSwapTemplate;
/**
* A swap index.
*
* Swap rates for different currencies are published by several providers. The indices
* can be related to IBOR-linked swaps or Overnight-linked swaps.
* Ref: https://quant.opengamma.io/Interest-Rate-Instruments-and-Market-Conventions.pdf
*
* The most common implementations are provided in {@link SwapIndices}.
*
* All implementations of this interface must be immutable and thread-safe.
*/
public interface SwapIndex
extends Index, Named {
/**
* Obtains an instance from the specified unique name.
*
* @param uniqueName the unique name
* @return the index
* @throws IllegalArgumentException if the name is not known
*/
@FromString
public static SwapIndex of(String uniqueName) {
ArgChecker.notNull(uniqueName, "uniqueName");
return extendedEnum().lookup(uniqueName);
}
/**
* Gets the extended enum helper.
*
* This helper allows instances of the index to be looked up.
* It also provides the complete set of available instances.
*
* @return the extended enum helper
*/
public static ExtendedEnum extendedEnum() {
return SwapIndices.ENUM_LOOKUP;
}
//-----------------------------------------------------------------------
/**
* Gets whether the index is active.
*
* Over time some indices become inactive and are no longer produced.
* If this occurs, this method will return false.
*
* @return true if the index is active, false if inactive
*/
public abstract boolean isActive();
/**
* Gets the fixing time of the index.
*
* The fixing time is related to the fixing date and time-zone.
*
* @return the fixing time
*/
public abstract LocalTime getFixingTime();
/**
* Gets the time-zone of the fixing time.
*
* The fixing time-zone is related to the fixing date and time.
*
* @return the time-zone of the fixing time
*/
public abstract ZoneId getFixingZone();
/**
* Gets the template for creating Fixed-Float swap.
*
* @return the template
*/
public abstract FixedFloatSwapTemplate getTemplate();
//-------------------------------------------------------------------------
/**
* Calculates the fixing date-time from the fixing date.
*
* The fixing date is the date on which the index is to be observed.
* The result combines the date with the time and zone stored in the index.
*
* No error is thrown if the input date is not a valid fixing date.
*
* @param fixingDate the fixing date
* @return the fixing date-time
*/
public default ZonedDateTime calculateFixingDateTime(LocalDate fixingDate) {
return fixingDate.atTime(getFixingTime()).atZone(getFixingZone());
}
//-------------------------------------------------------------------------
/**
* Gets the name that uniquely identifies this index.
*
* This name is used in serialization and can be parsed using {@link #of(String)}.
*
* @return the unique name
*/
@ToString
@Override
public abstract String getName();
}