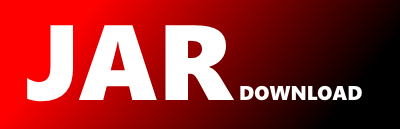
com.opengamma.strata.product.swap.SwapPaymentEvent Maven / Gradle / Ivy
Show all versions of strata-product Show documentation
/*
* Copyright (C) 2014 - present by OpenGamma Inc. and the OpenGamma group of companies
*
* Please see distribution for license.
*/
package com.opengamma.strata.product.swap;
import java.time.LocalDate;
import java.time.temporal.TemporalAdjuster;
import com.opengamma.strata.basics.currency.Currency;
import com.opengamma.strata.basics.date.BusinessDayAdjustment;
/**
* A payment event, where a single payment is made between two counterparties.
*
* Implementations of this interface represent a single payment event.
* Causes include exchange of notional amounts and fees.
*
* This interface imposes few restrictions on the payment events.
* The event must have a payment date and currency.
*
* Implementations must be immutable and thread-safe beans.
*/
public interface SwapPaymentEvent {
/**
* Gets the date that the payment is made.
*
* Each payment event has a single payment date.
* This date has been adjusted to be a valid business day.
*
* @return the payment date
*/
public abstract LocalDate getPaymentDate();
/**
* Gets the currency of the payment resulting from the event.
*
* This is the currency of the generated payment.
* An event has a single currency.
*
* @return the currency of the payment
*/
public abstract Currency getCurrency();
//-------------------------------------------------------------------------
/**
* Adjusts the payment date using the rules of the specified adjuster.
*
* The adjuster is typically an instance of {@link BusinessDayAdjustment}.
* Implementations must return a new instance unless they are immutable and no change occurs.
*
* @param adjuster the adjuster to apply to the payment date
* @return the adjusted payment event
*/
public abstract SwapPaymentEvent adjustPaymentDate(TemporalAdjuster adjuster);
//-------------------------------------------------------------------------
/**
* Checks whether the payment amount of an event is known at a given date.
*
* Each payment event may be a known amount at a given date, else it could be fixed at a later date
*
* @param date the date to check whether payment amount is known or not
* @return true if payment is fixed at given date
*/
public abstract boolean isKnownAmountAt(LocalDate date);
}