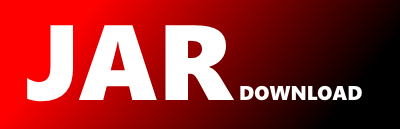
com.opensymphony.xwork2.util.finder.UrlSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xwork Show documentation
Show all versions of xwork Show documentation
XWork is an command-pattern framework that is used to power WebWork
as well as other applications. XWork provides an Inversion of Control
container, a powerful expression language, data type conversion,
validation, and pluggable configuration.
The newest version!
/*
* Copyright (c) 2002-2003 by OpenSymphony
* All rights reserved.
*/
package com.opensymphony.xwork2.util.finder;
import com.opensymphony.xwork2.util.logging.Logger;
import com.opensymphony.xwork2.util.logging.LoggerFactory;
import org.apache.commons.lang.StringUtils;
import java.io.File;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Use with ClassFinder to filter the Urls to be scanned, example:
*
* UrlSet urlSet = new UrlSet(classLoader);
* urlSet = urlSet.exclude(ClassLoader.getSystemClassLoader().getParent());
* urlSet = urlSet.excludeJavaExtDirs();
* urlSet = urlSet.excludeJavaEndorsedDirs();
* urlSet = urlSet.excludeJavaHome();
* urlSet = urlSet.excludePaths(System.getProperty("sun.boot.class.path", ""));
* urlSet = urlSet.exclude(".*?/JavaVM.framework/.*");
* urlSet = urlSet.exclude(".*?/activemq-(core|ra)-[\\d.]+.jar(!/)?");
*
* @author David Blevins
* @version $Rev$ $Date$
*/
public class UrlSet {
private static final Logger LOG = LoggerFactory.getLogger(UrlSet.class);
private final Map urls;
public UrlSet(ClassLoaderInterface classLoader) throws IOException {
this(getUrls(classLoader));
}
public UrlSet(URL... urls){
this(Arrays.asList(urls));
}
/**
* Ignores all URLs that are not "jar" or "file"
* @param urls
*/
public UrlSet(Collection urls){
this.urls = new HashMap();
for (URL location : urls) {
try {
// if (location.getProtocol().equals("file")) {
// try {
// // See if it's actually a jar
// URL jarUrl = new URL("jar", "", location.toExternalForm() + "!/");
// JarURLConnection juc = (JarURLConnection) jarUrl.openConnection();
// juc.getJarFile();
// location = jarUrl;
// } catch (IOException e) {
// }
// this.urls.put(location.toExternalForm(), location);
// }
this.urls.put(location.toExternalForm(), location);
} catch (Exception e) {
e.printStackTrace();
}
}
}
private UrlSet(Map urls) {
this.urls = urls;
}
public UrlSet include(UrlSet urlSet){
Map urls = new HashMap(this.urls);
urls.putAll(urlSet.urls);
return new UrlSet(urls);
}
public UrlSet exclude(UrlSet urlSet) {
Map urls = new HashMap(this.urls);
Map parentUrls = urlSet.urls;
for (String url : parentUrls.keySet()) {
urls.remove(url);
}
return new UrlSet(urls);
}
public UrlSet exclude(ClassLoaderInterface parent) throws IOException {
return exclude(new UrlSet(parent));
}
public UrlSet exclude(File file) throws MalformedURLException {
return exclude(relative(file));
}
public UrlSet exclude(String pattern) throws MalformedURLException {
return exclude(matching(pattern));
}
/**
* Calls excludePaths(System.getProperty("java.ext.dirs"))
* @return
* @throws MalformedURLException
*/
public UrlSet excludeJavaExtDirs() throws MalformedURLException {
return excludePaths(System.getProperty("java.ext.dirs", ""));
}
/**
* Calls excludePaths(System.getProperty("java.endorsed.dirs"))
*
* @return
* @throws MalformedURLException
*/
public UrlSet excludeJavaEndorsedDirs() throws MalformedURLException {
return excludePaths(System.getProperty("java.endorsed.dirs", ""));
}
public UrlSet excludeJavaHome() throws MalformedURLException {
String path = System.getProperty("java.home");
File java = new File(path);
if (path.matches("/System/Library/Frameworks/JavaVM.framework/Versions/[^/]+/Home")){
java = java.getParentFile();
}
return exclude(java);
}
public UrlSet excludePaths(String pathString) throws MalformedURLException {
String[] paths = pathString.split(File.pathSeparator);
UrlSet urlSet = this;
for (String path : paths) {
if (StringUtils.isNotEmpty(path)) {
File file = new File(path);
urlSet = urlSet.exclude(file);
}
}
return urlSet;
}
public UrlSet matching(String pattern) {
Map urls = new HashMap();
for (Map.Entry entry : this.urls.entrySet()) {
String url = entry.getKey();
if (url.matches(pattern)){
urls.put(url, entry.getValue());
}
}
return new UrlSet(urls);
}
public UrlSet relative(File file) throws MalformedURLException {
String urlPath = file.toURL().toExternalForm();
Map urls = new HashMap();
for (Map.Entry entry : this.urls.entrySet()) {
String url = entry.getKey();
if (url.startsWith(urlPath) || url.startsWith("jar:"+urlPath)){
urls.put(url, entry.getValue());
}
}
return new UrlSet(urls);
}
public List getUrls() {
return new ArrayList(urls.values());
}
private static List getUrls(ClassLoaderInterface classLoader) throws IOException {
List list = new ArrayList();
//find jars
ArrayList urls = Collections.list(classLoader.getResources("META-INF"));
for (URL url : urls) {
if ("jar".equalsIgnoreCase(url.getProtocol())) {
String externalForm = url.toExternalForm();
//build a URL pointing to the jar, instead of the META-INF dir
url = new URL(StringUtils.substringBefore(externalForm, "META-INF"));
list.add(url);
} else if (LOG.isDebugEnabled())
LOG.debug("Ignoring URL [#0] because it is not a jar", url.toExternalForm());
}
//usually the "classes" dir
list.addAll(Collections.list(classLoader.getResources("")));
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy