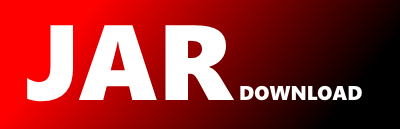
com.opentext.ia.sdk.sip.ContentAssemblerDefault Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infoarchive-sdk-core Show documentation
Show all versions of infoarchive-sdk-core Show documentation
A library that makes it quick and easy to create SIPs in InfoArchive
/*
* Copyright (c) 2016-2017 by OpenText Corporation. All Rights Reserved.
*/
package com.opentext.ia.sdk.sip;
import java.io.IOException;
import java.io.InputStream;
import java.util.Collection;
import java.util.Iterator;
import java.util.Map;
import java.util.TreeMap;
import org.apache.commons.io.output.NullOutputStream;
import com.opentext.ia.sdk.support.io.EncodedHash;
import com.opentext.ia.sdk.support.io.HashAssembler;
import com.opentext.ia.sdk.support.io.IOStreams;
import com.opentext.ia.sdk.support.io.ZipAssembler;
/**
* The default ContentAssembler implementation. Will perform no de-duplication and no validation.
* @param The type of domain object to assemble SIPs from
*/
public class ContentAssemblerDefault implements ContentAssembler {
private static final int BUFFER_SIZE = 4096;
private final DigitalObjectsExtraction contentsExtraction;
private final HashAssembler contentHashAssembler;
private ZipAssembler zip;
private Counters metrics;
public ContentAssemblerDefault(DigitalObjectsExtraction contentsExtraction, HashAssembler contentHashAssembler) {
this.contentsExtraction = contentsExtraction;
this.contentHashAssembler = contentHashAssembler;
}
@Override
public synchronized void begin(ZipAssembler aZip, Counters aMetrics) {
this.zip = aZip;
this.metrics = aMetrics;
}
public DigitalObjectsExtraction getContentsExtraction() {
return contentsExtraction;
}
public HashAssembler getContentHashAssembler() {
return contentHashAssembler;
}
private Counters ensureMetrics() {
if (metrics == null) {
throw new IllegalStateException("Missing metrics; did you call begin()?");
}
return metrics;
}
public synchronized Counters getMetrics() {
return ensureMetrics().forReading();
}
@Override
public Map addContentsOf(D domainObject) throws IOException {
Map result = new TreeMap<>();
Iterator extends DigitalObject> digitalObjects = contentsExtraction.apply(domainObject);
while (digitalObjects.hasNext()) {
DigitalObject digitalObject = digitalObjects.next();
incMetric(SipMetrics.NUM_DIGITAL_OBJECTS, 1);
String entry = digitalObject.getReferenceInformation();
result.put(entry, addContent(entry, digitalObject));
}
return result;
}
protected synchronized void incMetric(String metric, long delta) {
ensureMetrics().inc(metric, delta);
}
protected synchronized ContentInfo addContent(String ri, DigitalObject digitalObject) throws IOException {
if (zip == null) {
throw new IllegalStateException("Missing zip; did youc call begin()?");
}
try (InputStream stream = digitalObject.get()) {
String referenceInformation = digitalObject.getReferenceInformation();
Collection hashes;
long numBytesHashed;
synchronized (zip) {
hashes = zip.addEntry(referenceInformation, stream, contentHashAssembler);
numBytesHashed = contentHashAssembler.numBytesHashed();
}
incMetric(SipMetrics.SIZE_DIGITAL_OBJECTS, numBytesHashed);
return new ContentInfo(ri, hashes);
}
}
protected Collection contentHashFor(InputStream stream) throws IOException {
final HashAssembler hashAssembler = getContentHashAssembler();
synchronized (hashAssembler) {
hashAssembler.initialize();
IOStreams.copy(stream, NullOutputStream.NULL_OUTPUT_STREAM, BUFFER_SIZE, hashAssembler);
return hashAssembler.get();
}
}
protected synchronized void addZipEntry(String name, InputStream content, HashAssembler hashAssembler)
throws IOException {
if (zip == null) {
throw new IllegalStateException("Missing zip; did you call begin()?");
}
zip.addEntry(name, content, hashAssembler);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy