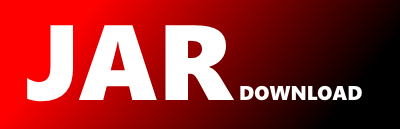
com.opentext.ia.sdk.sip.Counters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infoarchive-sdk-core Show documentation
Show all versions of infoarchive-sdk-core Show documentation
A library that makes it quick and easy to create SIPs in InfoArchive
/*
* Copyright (c) 2016-2017 by OpenText Corporation. All Rights Reserved.
*/
package com.opentext.ia.sdk.sip;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
/**
* Set of related counters.
*/
public class Counters {
private final Map values;
public Counters() {
this(new HashMap<>());
}
Counters(Map values) {
this.values = values;
}
public long get(String name) {
Long result = values.get(name);
return result == null ? 0 : result.longValue();
}
/**
* Increase the metric with the given name by one.
* @param name The name of the metric
*/
public void inc(String name) {
inc(name, 1);
}
/**
* Increase the metric with the given name by the given amount.
* @param name The name of the metric
* @param delta The amount to increase the metric with
*/
public void inc(String name, long delta) {
set(name, get(name) + delta);
}
/**
* Set the metric with the given name to the given value.
* @param name The name of the metric
* @param value The value to set the metric to
*/
public void set(String name, long value) {
values.put(name, value);
}
/**
* Reset all metrics to zero.
*/
public void reset() {
values.clear();
}
public Counters forReading() {
return new Counters(Collections.unmodifiableMap(new HashMap(values)));
}
@Override
public String toString() {
return values.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy